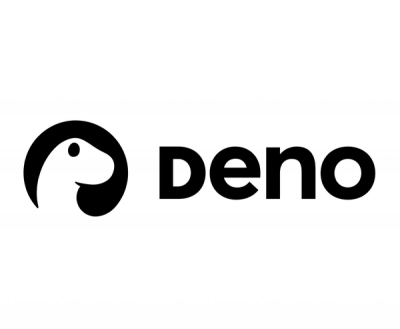
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@leafygreen-ui/polymorphic
Advanced tools
pol·y·morph (noun)
- an organism, inorganic object or material which takes various forms.
pol·y·mor·phic (adjective)
- occurring in several different forms, in particular with reference to species or genetic variation.
- (of a feature of a programming language) allowing routines to use variables of different types at different times.
Polymorphic
is a suite of types, hooks, components and factories that allows users to create components that can render as any HTML element or React component.
There are two main ways to use Polymorphic
, depending on whether the as
prop is defined internally by your component, or passed in as an external prop.
If the logic defining the as
prop is defined internally within your component, use the standalone Polymorph
component.
interface MyProps {
someProp: string;
}
const MyComponent = (props: MyProps) => {
const shouldRenderAs = 'button';
return <Polymorph as={shouldRenderAs} {...props} />;
};
If you want to expose as
as a prop of your component, use the Polymorphic
factory function and related hooks.
Note that any inherited props will be indeterminate in the factory function, since the value as
is not known. (i.e. the attributes of rest
in the following example are unknown).
interface MyProps {
someProp: string;
}
const MyComponent = Polymorphic<MyProps>(({ as, ...rest }) => {
const { Component, ref } = usePolymorphic(as);
return <Component ref={ref} {...rest} />;
});
Accepting a forwarded ref to a Polymorphic component is as simple as passing in a ref into the render function (same as React.forwardRef
).
interface MyProps {
someProp: string;
}
const MyComponent = Polymorphic<MyProps>(({ as, ...rest }, forwardedRef) => {
const { Component } = usePolymorphic(as);
return <Component ref={forwardedRef} {...rest} />;
});
as
propComponents extended using the Polymorphic
factory function can be made to infer the as
prop value based on the href
passed in.
Ensure the custom props are wrapped in InferredPolymorphicProps
, and use the useInferredPolymorphic
hook.
Make sure to pass both as
and a rest
object (that may contain href
) into the hook.
export const MyInferredComponent = Polymorphic<
InferredPolymorphicProps<MyProps>
>(({ as, ...rest }) => {
const { Component, ref } = useInferredPolymorphic(as, rest);
return (
<Component ref={ref} {...rest}>
{title}
</Component>
);
});
//
<MyInferredComponent href="mongodb.design" />; // renders as <a>
styled
APIPolymorphic
also supports usage with Emotions styled
API. To get TypeScript to accept the Polymorphic props you'll need to explicitly type your styled component as PolymorphicComponentType
.
const StyledPolymorph = styled(Polymorph)`
color: hotpink;
` as PolymorphicComponentType;
// or
const MyStyledComponent = styled(MyComponent)`
color: hotpink;
` as PolymorphicComponentType;
This also works with InferredPolymorphic components
const StyledInferred = styled(MyInferredComponent)`
color: hotpink;
` as InferredPolymorphicComponentType;
Note: TSDocs will not compile for styled polymorphs. This can be remedied by creating a wrapper around the styled function that explicitly returns a PolymorphicComponentType
While it is possible to use the Polymorph
component to extend polymorphic behavior, it can be much more verbose and error prone than using the Polymorphic
factory function. For completeness, an example of how to do this is provided below:
type MyProps<T extends PolymorphicAs> = PolymorphicPropsWithRef<
T,
{
someProp: string;
}
>;
export const MyComponent = <T extends PolymorphicAs = 'div'>(
{ as, title, ...rest }: MyProps<T>,
forwardedRef: PolymorphicRef<T>,
) => {
return (
<Polymorph as={as as PolymorphicAs} ref={forwardedRef} {...rest}>
{title}
</Polymorph>
);
};
FAQs
LeafyGreen UI Kit Polymorphic
The npm package @leafygreen-ui/polymorphic receives a total of 433,433 weekly downloads. As such, @leafygreen-ui/polymorphic popularity was classified as popular.
We found that @leafygreen-ui/polymorphic demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.