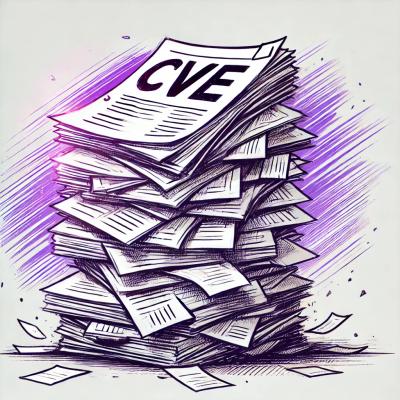
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@liblab/sdk
Advanced tools
The Typescript SDK for Liblab.
npm install sdk
To see whether an endpoint needs a specific type of authentication check the endpoint's documentation.
The Liblab API uses access tokens as a form of authentication. You can set the access token when initializing the SDK through the constructor:
const sdk = new Liblab('YOUR_ACCESS_TOKEN')
Or through the setAccessToken
method:
const sdk = new Liblab()
sdk.setAccessToken('YOUR_ACCESS_TOKEN')
You can also set it for each service individually:
const sdk = new Liblab()
sdk.build.setAccessToken('YOUR_ACCESS_TOKEN')
Here is a simple program demonstrating usage of this SDK. It can also be found in the examples/src/index.ts
file in this directory.
When running the sample make sure to use npm install
to install all the dependencies.
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
try {
const result = await sdk.build
.getBuildStatuses();
console.log(result);
} catch (err) {
const error = err as Error;
console.error(error.message);
}
})();
Here is the list of all available environments:
DEFAULT = 'https://api-dev.liblab.com',
PRODUCTION = 'https://api.liblab.com',
STAGING = 'https://api-staging.liblab.com',
DEVELOPMENT = 'https://api-dev.liblab.com'
How to set the environment:
const sdk = new Liblab();
sdk.setEnvironment(Environment.DEFAULT);
A list of all services and services methods.
Services
Method | Description |
---|---|
createBuild | |
getBuilds | |
createSimpleBuild | |
createBuildArtifact | |
getBuildStatuses | |
getById | |
removeById | |
tag | |
untag | |
approveBuild | |
unApproveBuild |
Method | Description |
---|---|
getApiBuilds | |
getApiBuildTags | |
getApiSdks | |
getApiDocs | |
createApi | |
getApis | |
searchApis | |
getApiById | |
updateApi | |
getApiMembers | |
removeApi | |
getApiByOrgSlugAndApiSlug |
Method | Description |
---|---|
createOrg | |
getOrgs | |
searchOrgs | |
getOrgById | |
updateOrg | |
removeOrg | |
getApisByOrg | |
getOrgJobs | |
getDocsByOrg | |
getBuildByOrg | |
getOrgApiBuilds | |
getOrgArtifacts |
Method | Description |
---|---|
createMember | |
getByOrgId | |
updateMember | |
removeMember | |
leaveOrg | |
enableAllMembers | |
disableAllMembers |
Method | Description |
---|---|
createArtifact | |
getArtifacts | |
getArtifactStatuses | |
getArtifactById | |
removeArtifact |
Method | Description |
---|---|
createSdk | |
findSdks | |
getSdkById | |
removeSdk |
Method | Description |
---|---|
getApprovedByOrgSlugAndApiSlug | |
getAllApprovedByOrgSlugAndApiSlug | |
createDoc | |
findDocs | |
approve | |
unapprove | |
getDocById | |
removeDoc | |
updateDoc | |
getDownloadUrl |
Method | Description |
---|---|
sendShadowForm |
Method | Description |
---|---|
getActiveSubscription | |
cancelActiveSubscription | |
getActiveSubscriptionStatus | |
getSubscriptionPaymentMethodUpdateLink | |
getCheckoutLink |
Method | Description |
---|---|
getSubscriptionsOverview |
Method | Description |
---|---|
stripeWebhook | |
syncStripeSubscriptions |
Method | Description |
---|---|
getCurrentUser | |
createUser | |
getUsers | |
getUserById | |
updateUser | |
removeUser | |
updateEmailSubscription | |
getUserOrgs | |
getUserApis |
Method | Description |
---|---|
getSnippetsByBuildId |
Method | Description |
---|---|
uploadWorkflows |
Method | Description |
---|---|
validateSpec | |
getSpecValidation |
Method | Description |
---|---|
createToken | |
findTokensByUserId | |
getTokenById | |
removeToken |
Method | Description |
---|---|
createOrgInvite | |
redeemInvite | |
declineInvite | |
getReceivedInvites | |
getSentInvites | |
searchInvites | |
getInviteByCode |
Method | Description |
---|---|
resetPasswordAuth0 | |
passwordlessVerifyPassword | |
passwordlessSetupPassword |
Method | Description |
---|---|
getEnabledPlans |
Method | Description |
---|---|
getOrgInvoices |
Method | Description |
---|---|
healthCheckControllerCheck |
Method | Description |
---|---|
create | |
search |
Method | Description |
---|---|
askAboutSpec |
Method | Description |
---|---|
sendFeedback |
Method | Description |
---|---|
getUserEvents | |
exportUserEventsToCsv | |
trackUserPublishPrEvent |
Method | Description |
---|---|
thirdPartyApplicationsControllerCreate | |
thirdPartyApplicationsControllerGetAll | |
thirdPartyApplicationsControllerGetByOrgId | |
thirdPartyApplicationsControllerDeleteById |
Required Parameters
| input | object | Request body. |
Return Type
BuildResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {};
const result = await sdk.build.createBuild(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number | |
orgId | number | |
apiSlug | string |
Return Type
PaginatedBuildResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.getBuilds(
-83215341.94533959,
-67616548.5623457,
20442911.769958094,
'apiSlug',
);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
BuildResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
apiId: 1,
authentication: {},
baseUrl: 'https://api-dev.liblab.com',
docs: ['enhancedApiSpec', 'snippets', 'api'],
languages: ['typescript'],
liblabVersion: '2',
sdkName: 'liblab',
sdkVersion: '1.0.0',
};
const result = await sdk.build.createSimpleBuild(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
input | object | Request body. |
Return Type
BuildResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {};
const result = await sdk.build.createBuildArtifact(input, 99362000.98300713);
console.log(result);
})();
Return Type
GetBuildStatusesResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.getBuildStatuses();
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetBuildByIdResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.getById(63015827.67131135);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
buildId | number | |
apiSlug | string | |
orgId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.removeById(-87790891.51382822, 'apiSlug', 11847050.501020283);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
buildId | number | |
tagId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.tag(42243271.19032255, -26577272.567566454);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
buildId | number | |
tagId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.untag(-97538115.75367513, 81360428.68486172);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
buildId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.approveBuild(45781238.526785195);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
buildId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.build.unApproveBuild(-89324465.47852635);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
sortBy | SortBy | |
direction | Direction | |
statuses | string[] | |
tags | number[] | |
createdByIds | number[] |
Return Type
PaginatedBuildResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApiBuilds(
73122582.83314651,
-76646165.75125955,
-40711089.17931215,
{
sortBy: 'status',
direction: 'asc',
statuses: ['SUCCESS', 'IN_PROGRESS'],
tags: [17013901.21561323, 35085404.26250577],
createdByIds: [-90017651.1106793, 9051541.281343937],
},
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetApiBuildTagsResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApiBuildTags(-22629740.42899652);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
statuses | string[] | |
tags | number[] | |
createdByIds | number[] | |
languages | string[] | |
sortBy | ApiSortBy | |
direction | Direction |
Return Type
PaginatedSdkResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApiSdks(
-47544081.907073244,
-83041543.73743567,
-65057253.91768037,
{
statuses: ['FAIL', 'IN_PROGRESS'],
tags: [87907600.5582884, 84097925.32551038],
createdByIds: [81236044.3826249, -23845287.931775033],
languages: ['CSHARP', 'PHP'],
sortBy: 'createdAt',
direction: 'asc',
},
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
sortBy | ApiSortBy | |
direction | Direction | |
statuses | string[] | |
tags | number[] | |
createdByIds | number[] |
Return Type
PaginatedDocResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApiDocs(
-85119973.52979898,
-14990792.37830703,
-65279828.7217211,
{
sortBy: 'createdAt',
direction: 'asc',
statuses: ['IN_PROGRESS', 'FAIL'],
tags: [4690866.823789671, -34674244.75452545],
createdByIds: [79518371.36911887, 53153983.94148186],
},
);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
ApiResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {};
const result = await sdk.api.createApi(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
apiSlug | string |
Return Type
GetApisResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApis(-94541188.40029979, { apiSlug: 'apiSlug' });
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
name | string | |
sortBy | ApiSortBy | |
orgId | number | |
direction | ApiDirection | |
orgIds | number[] |
Return Type
ApisSearchPaginatedResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.searchApis(46348869.209112704, -95028065.33808433, {
name: 'name',
sortBy: 'createdAt',
orgId: 98045618.3279599,
direction: 'desc',
orgIds: [-55586208.288178615, 74464424.24137682],
});
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
ApiResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApiById(38791569.15292171);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
input | object | Request body. |
Return Type
ApiResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { name: 'My api name', version: '1.0.1' };
const result = await sdk.api.updateApi(input, -9082398.051585153);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetApiMembersResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApiMembers(-8539381.25681442);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
apiSlug | string | |
orgId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.removeApi('apiSlug', -45189898.51708599);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgSlug | string | |
apiSlug | string |
Return Type
GetApiByOrgSlugAndApiSlugResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.api.getApiByOrgSlugAndApiSlug('orgSlug', 'apiSlug');
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
OrgResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
description: 'Example Org Description',
domain: 'business.com',
logoUrl: 'https://liblab.com/images/logo.png',
name: 'Example Org',
website: 'https://example.com',
};
const result = await sdk.org.createOrg(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
direction | Direction | |
sortBy | OrgSortBy |
Return Type
AdminPaginatedOrgResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getOrgs(-99689478.85931012, -73610302.9699369, {
direction: 'asc',
sortBy: 'startTime',
});
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
website | string | |
domain | string | |
name | string |
Return Type
AdminPaginatedOrgResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.searchOrgs(-65231341.547675245, -95393184.27051769, {
website: 'website',
domain: 'domain',
name: 'name',
});
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetOrgByIdResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getOrgById(-82005424.77297139);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
input | object | Request body. |
Return Type
OrgResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
description: 'Example Org Description',
domain: 'example.com',
isAllowedForBeta: true,
logoUrl: 'https://liblab.com/images/logo.png',
name: 'Example Org',
remainingCredits: 19,
website: 'https://example.com',
};
const result = await sdk.org.updateOrg(input, -8710292.057702377);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.removeOrg(-80555927.46942294);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetApisByOrgResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getApisByOrg(-59539046.88916496);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
sortBy | OrgSortBy | |
direction | Direction | |
statuses | string[] | |
createdByIds | number[] | |
apiSlug | string | |
apiVersion | string | |
buildType | string[] |
Return Type
PaginatedOrgJobsResponseWithTotalCount
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getOrgJobs(
-8425584.610679105,
-75083795.83248681,
-17677991.079212114,
{
sortBy: 'status',
direction: 'asc',
statuses: ['SUCCESS', 'IN_PROGRESS'],
createdByIds: [40409271.917010814, -77479505.92979994],
apiSlug: 'apiSlug',
apiVersion: 'apiVersion',
buildType: ['DOC', 'SDK'],
},
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetDocsByOrgResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getDocsByOrg(-80024009.40144874);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
sortBy | OrgSortBy | |
direction | Direction | |
statuses | string[] | |
tags | number[] | |
createdByIds | number[] | |
apiSlug | string | |
apiVersion | string |
Return Type
PaginatedOrgBuildsWithJobsResponseWithTotalCount
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getBuildByOrg(
40354778.52739784,
-52073488.43756887,
-81565294.52232155,
{
sortBy: 'status',
direction: 'asc',
statuses: ['IN_PROGRESS', 'IN_PROGRESS'],
tags: [-41172638.86242304, -10922224.555274561],
createdByIds: [-79837103.07438563, 56025660.461156964],
apiSlug: 'apiSlug',
apiVersion: 'apiVersion',
},
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetOrgApiBuildsResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getOrgApiBuilds(-63499547.471065655);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
sortBy | OrgSortBy | |
direction | OrgDirection | |
artifactTypes | ArtifactTypes | |
statuses | OrgStatuses | |
createdByIds | number[] |
Return Type
PaginatedOrgArtifactsResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.org.getOrgArtifacts(
96898176.24526751,
8628543.6252639,
-92761027.76756868,
{
sortBy: 'startTime',
direction: 'desc',
artifactTypes: ['DOC', 'SDK'],
statuses: [{ imports: [] }, { imports: [] }],
createdByIds: [24013387.06917602, 44921439.06854901],
},
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number | |
input | object | Request body. |
Return Type
OrgMemberResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { role: 'MEMBER', userId: 1 };
const result = await sdk.orgMember.createMember(input, -28149763.41898538);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number | |
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
string | ||
firstName | string | |
lastName | string | |
sortBy | OrgMemberSortBy | |
direction | Direction |
Return Type
PaginatedOrgMemberResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgMember.getByOrgId(
90027733.61251837,
25823948.840221122,
-86258213.58463855,
{
email: 'email',
firstName: 'firstName',
lastName: 'lastName',
sortBy: 'createdAt',
direction: 'asc',
},
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
userId | number | |
orgId | number | |
input | object | Request body. |
Return Type
OrgMemberResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { orgId: 1, role: 'MEMBER' };
const result = await sdk.orgMember.updateMember(input, 67387276.54156339, -80791721.6214693);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
userId | number | |
orgId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgMember.removeMember(-33572158.607363135, 19831152.721365303);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgMember.leaveOrg(40619385.2057533);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Return Type
UpdateManyOrgMembersResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgMember.enableAllMembers(37911071.82434389);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Return Type
UpdateManyOrgMembersResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgMember.disableAllMembers(58312339.508603066);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
ArtifactResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
artifactType: 'DOC',
bucketKey: 'bucketKey',
bucketName: 'bucketName',
buildId: 1,
status: 'SUCCESS',
};
const result = await sdk.artifact.createArtifact(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
buildId | number |
Return Type
GetArtifactsResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.artifact.getArtifacts(41911406.76875889);
console.log(result);
})();
Return Type
GetArtifactStatusesResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.artifact.getArtifactStatuses();
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
ArtifactResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.artifact.getArtifactById(94016051.5604473);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.artifact.removeArtifact(-59966937.95277568);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
SdkResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
artifactId: 1,
fileLocation: 'https://my-file.location',
language: 'JAVA',
version: '1.0.0',
};
const result = await sdk.sdk.createSdk(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
artifactId | number | |
sortBy | SdkSortBy | |
direction | Direction | |
languages | string[] |
Return Type
PaginatedSdkResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.sdk.findSdks(-31124935.740910724, -51735411.72089415, {
artifactId: -16617744.312318414,
sortBy: 'version',
direction: 'asc',
languages: ['TERRAFORM', 'TYPESCRIPT'],
});
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
SdkResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.sdk.getSdkById(60387710.818670064);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.sdk.removeSdk(-21852972.692654938);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgSlug | string |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
apiSlug | string | |
apiVersion | string |
Return Type
DocResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.getApprovedByOrgSlugAndApiSlug('orgSlug', {
apiSlug: 'apiSlug',
apiVersion: 'apiVersion',
});
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgSlug | string |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
apiSlug | string | |
apiVersion | string |
Return Type
GetAllApprovedByOrgSlugAndApiSlugResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.getAllApprovedByOrgSlugAndApiSlug('orgSlug', {
apiSlug: 'apiSlug',
apiVersion: 'apiVersion',
});
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
DocCreatedResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
apiId: -52163707.012023374,
artifactId: 1,
fileLocation: 'https://example.com',
previewSlug: 'previewSlug',
version: '1.0.0',
};
const result = await sdk.doc.createDoc(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number | |
artifactId | number |
Return Type
PaginatedDocResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.findDocs(
22167091.544198006,
-31973874.040948853,
-75183554.20543864,
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
previewSlug | string |
Return Type
DocResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.approve('previewSlug');
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
previewSlug | string |
Return Type
DocResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.unapprove('previewSlug');
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
DocResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.getDocById(25596416.20404589);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.removeDoc(4077165.4730666876);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
input | object | Request body. |
Return Type
DocResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { fileLocation: 'https://example.com', version: '1.0.0' };
const result = await sdk.doc.updateDoc(input, -61401299.312518895);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
DocDownloadResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.doc.getDownloadUrl(33286979.682141155);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { fields: [{ name: 'test-name', value: 'test-field' }] };
const result = await sdk.hubSpot.sendShadowForm(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Return Type
SubscriptionResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgSubscriptions.getActiveSubscription(82491487.84293982);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Return Type
SubscriptionResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgSubscriptions.cancelActiveSubscription(88247792.79261377);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Return Type
GetActiveSubscriptionStatusResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgSubscriptions.getActiveSubscriptionStatus(-35399477.57252699);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number | |
subscriptionId | number |
Return Type
CheckoutLinkResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgSubscriptions.getSubscriptionPaymentMethodUpdateLink(
-90878399.28328724,
-74308026.39640644,
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number | |
planId | number | |
billingInterval | BillingInterval |
Return Type
CheckoutLinkResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.orgSubscriptions.getCheckoutLink(
-59719059.54607135,
-48096601.571066566,
'year',
);
console.log(result);
})();
Return Type
SubscriptionsOverviewResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.subscriptions.getSubscriptionsOverview();
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
stripeSignature | string |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.paymentProvider.stripeWebhook('stripe-signature');
console.log(result);
})();
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.paymentProvider.syncStripeSubscriptions();
console.log(result);
})();
Return Type
CurrentUserResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.user.getCurrentUser();
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
UserResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
auth0Id: 'auth0|123',
email: 'someone@example.com',
firstName: 'John',
lastName: 'Doe',
password: 'Password123!',
signupMethod: 'DEFAULT',
};
const result = await sdk.user.createUser(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
orgId | number | |
string | ||
firstName | string | |
lastName | string | |
orgIds | number[] | |
sortBy | UserSortBy | |
direction | UserDirection |
Return Type
UsersResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.user.getUsers(19669944.431398228, -97748849.35278863, {
orgId: -49057939.84840266,
email: 'email',
firstName: 'firstName',
lastName: 'lastName',
orgIds: [-26364785.142752737, 92116343.20718268],
sortBy: 'createdAt',
direction: 'desc',
});
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
UserResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.user.getUserById(-34024660.77657325);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number | |
input | object | Request body. |
Return Type
UserResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
auth0Id: 'auth0Id',
email: 'someone@example.com',
firstName: 'John',
isEnabled: true,
isLiblabAdmin: true,
isLiblabFinanceAdmin: false,
lastName: 'Doe',
refreshTokenHash: 'refreshTokenHash',
utmParams: {},
};
const result = await sdk.user.updateUser(input, -44381185.20403735);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.user.removeUser(-77376500.19288468);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { isSubscribedToEmails: true };
const result = await sdk.user.updateEmailSubscription(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Return Type
PaginatedOrgResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.user.getUserOrgs(6719537.392789692, -13688248.110467881);
console.log(result);
})();
Return Type
GetUserApisResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.user.getUserApis();
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
SnippetsResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.snippets.getSnippetsByBuildId(75473230.04976979);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
CreateWorkflowsResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {};
const result = await sdk.workflows.uploadWorkflows(input);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
SpecValidationResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { apiId: 1 };
const result = await sdk.specValidation.validateSpec(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
SpecValidationResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.specValidation.getSpecValidation(56875084.772369325);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
CreateTokenResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
expiresAt: '2025-01-01T01:01:01.0Z',
name: 'My token',
scope: ['API', 'DOC', 'SDK', 'BUILD', 'ARTIFACT', 'ORG'],
};
const result = await sdk.token.createToken(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
userId | number |
Return Type
FindTokensByUserIdResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.token.findTokensByUserId(-33619416.07427017);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
GetTokenResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.token.getTokenById(66298940.83619732);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.token.removeToken(7383298.7622538805);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number | |
input | object | Request body. |
Return Type
InvitationResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { email: 'harry@liblab.com' };
const result = await sdk.invitation.createOrgInvite(input, 30906176.020276234);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
inviteCode | string |
Return Type
InvitationResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.invitation.redeemInvite('inviteCode');
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
inviteCode | string |
Return Type
InvitationResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.invitation.declineInvite('inviteCode');
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Return Type
PaginatedOrgInvitesResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.invitation.getReceivedInvites(60400507.19605091, -32707400.074951097);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Return Type
PaginatedOrgInvitesResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.invitation.getSentInvites(-87054814.25675425, -67860905.79077382);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
orgName | string | Name of the organization |
status | Status | Status of the invitation |
sortBy | InvitationSortBy | Field to sort by |
direction | InvitationDirection | Direction to sort by |
Return Type
PaginatedOrgInvitesResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.invitation.searchInvites(-33864949.04397579, -35153948.74386892, {
orgName: 'orgName',
status: 'REDEEMED',
sortBy: 'createdAt',
direction: 'desc',
});
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
inviteCode | string |
Return Type
InvitationResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.invitation.getInviteByCode('inviteCode');
console.log(result);
})();
Return Type
Auth0ResetPasswordResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.auth0.resetPasswordAuth0();
console.log(result);
})();
Return Type
VerifyPasswordResponseDto
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.auth0.passwordlessVerifyPassword();
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { password: 'P@ssw0rd123' };
const result = await sdk.auth0.passwordlessSetupPassword(input);
console.log(result);
})();
Return Type
GetEnabledPlansResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.plan.getEnabledPlans();
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
orgId | number |
Return Type
OrgInvoicesResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.invoice.getOrgInvoices(34417706.94895059);
console.log(result);
})();
Return Type
HealthCheckResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.healthCheck.healthCheckControllerCheck();
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
TagResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { name: 'tag' };
const result = await sdk.tags.create(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number | |
searchQuery | string |
Return Type
SearchResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.tags.search(5833277.400742397, -74395721.36800645, 'searchQuery');
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
AskAboutSpecResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { buildId: 12345, prompt: 'How can I login in this api?' };
const result = await sdk.ai.askAboutSpec(input);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { message: 'message', title: 'title' };
const result = await sdk.feedback.sendFeedback(input);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
offset | number | |
limit | number |
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
string | ||
orgId | number | |
sortBy | UserEventSortBy | |
direction | UserEventDirection | |
orgIds | number[] | |
eventIds | number[] |
Return Type
PaginatedUserEventsResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.userEvent.getUserEvents(61932207.06150472, -59754773.50568741, {
email: 'email',
orgId: 13889079.682712018,
sortBy: 'timestamp',
direction: 'desc',
orgIds: [-90447434.59200265, -65964410.4207942],
eventIds: [-53763964.83424015, 65144471.04700917],
});
console.log(result);
})();
Optional Parameters
Optional parameters are passed as part of the last parameter to the method. Ex. {optionalParam1 : 'value1', optionalParam2: 'value2'}
Name | Type | Description |
---|---|---|
string | ||
orgId | number | |
filename | string | |
orgIds | number[] | |
eventIds | number[] |
Return Type
ExportUserEventsToCsvResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.userEvent.exportUserEventsToCsv({
email: 'email',
orgId: 64324217.4852781,
filename: 'filename',
orgIds: [-92228321.42203777, 51774758.403790146],
eventIds: [79154458.70100442, 84449590.64936987],
});
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = { language: 'typescript', sdk: 'My SDK', success: true };
const result = await sdk.userEvent.trackUserPublishPrEvent(input);
console.log(result);
})();
Required Parameters
| input | object | Request body. |
Return Type
ThirdPartyApplicationResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const input = {
callbackUrls: ['http://localhost:3000/api/auth/callback'],
logoUrl: 'https://liblab.com/img/logo.svg',
name: 'third-party application',
orgId: 1,
};
const result = await sdk.thirdPartyApplications.thirdPartyApplicationsControllerCreate(input);
console.log(result);
})();
Return Type
ThirdPartyApplicationsControllerGetAllResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.thirdPartyApplications.thirdPartyApplicationsControllerGetAll();
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
ThirdPartyApplicationsControllerGetByOrgIdResponse
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.thirdPartyApplications.thirdPartyApplicationsControllerGetByOrgId(
-25288976.18974875,
);
console.log(result);
})();
Required Parameters
Name | Type | Description |
---|---|---|
id | number |
Return Type
Returns a dict object.
Example Usage Code Snippet
import { Liblab } from '@liblab/sdk';
const sdk = new Liblab({ accessToken: process.env.LIBLAB_ACCESS_TOKEN });
(async () => {
const result = await sdk.thirdPartyApplications.thirdPartyApplicationsControllerDeleteById(
-26711852.81312667,
);
console.log(result);
})();
License: MIT. See license in LICENSE.
FAQs
Liblab
We found that @liblab/sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.