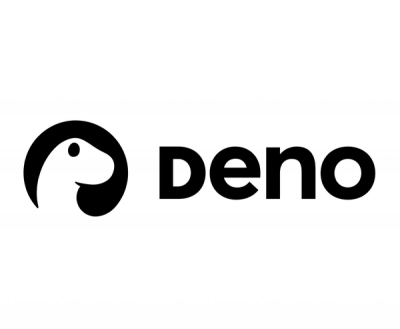
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@lildiary/vk-bot
Advanced tools
🤖 VK bot framework for Node.js, based on Bots Long Poll API, Promises, EventEmmiter and TypeScript
🤖 Scalable VK bot framework for Node.js, based on Bots Long Poll API and Callback API. Based on node-vk-bot-api
$ npm i @lildiary/vk-bot
import { VkBot } from "@lildiary/vk-bot";
const bot = new VkBot("TOKEN");
bot.command("/start", ctx => {
ctx.reply("Hello!");
});
bot.once("startPoll", () => {
console.log("Long polling started");
});
bot.startPolling();
There's a few simple examples.
$ npm test
Create bot.
// Simple usage
const bot = new VkBot("TOKEN");
// Advanced usage
const bot = new VkBot({
token: process.env.TOKEN,
group_id: process.env.GROUP_ID,
execute_timeout: process.env.EXECUTE_TIMEOUT, // in ms (50 by default)
polling_timeout: process.env.POLLING_TIMEOUT // in secs (25 by default),
v: '5.103', // Vk version, we do not recomend to change it
pollingVersion: 3 // Vk Polling version, we do not recommend to change it
});
Add simple middleware.
bot.use((ctx, next) => {
ctx.message.timestamp = new Date().getTime();
next();
});
Add middlewares with triggers for message_new
event.
bot.command("start", ctx => {
ctx.reply("Hello!").then(() => {
console.log("The message is successfuly sent");
});
});
Add middlewares with triggers for selected events.
bot.event("message_edit", ctx => {
ctx.reply("Your message was editted");
});
Add reserved middlewares without triggers.
bot.noCommand(ctx => {
ctx.reply("No commands for you.");
});
Send message to user.
// Simple usage
bot.sendMessage(145003487, "Hello!", "photo1_1");
// Multiple recipients
bot.sendMessage([145003487, 145003488], "Hello!", "photo1_1");
// Advanced usage
bot.sendMessage(145003487, {
message: "Hello!",
lat: 59.939095,
lng: 30.315868
});
// Send image
const file = fs.readFileSync('C:/Users/M4k5y/Projects/lilDiary/Services/bot/logo.png');
bot.sendMessage(145003487, 'Look at my images!', file); // file is a png image Buffer
Start polling ts is timestamp of the last event you can get events after ts is not required
bot.startPolling(ts);
Set event listener, useful for saving last ts to DataBase
bot.on("poll", ts => {
console.log(`Poll is done, ts: ${ts}`);
});
bot.on("error", err => {
console.log(err);
});
bot.startPolling();
"startPoll" - emits when polling starts "poll" - when poll ends, returns ts "error" - emmits error
Set event listener which excecutes once
bot.once("startPoll", ts => {
console.log("Bot started");
});
bot.startPolling();
Helper method for reply to the current user.
bot.command("start", ctx => {
ctx.reply("Hello!");
});
Markup.keyboard(buttons, options)
: Create keyboardMarkup.button(label, color, payload)
: Create custom buttonMarkup.oneTime()
: Set oneTime to keyboardMarkup.inline()
: Send keyboard with the messagectx.reply(
"Select your sport",
null,
Markup.keyboard(["Football", "Basketball"]).inline()
);
ctx.reply(
"How are you doing?",
null,
Markup.keyboard([
[Markup.button("Normally", "primary")],
[Markup.button("Fine", "positive"), Markup.button("Bad", "negative")]
])
);
Create keyboard with optional settings.
/*
Each string has maximum 2 columns.
| one | two |
| three | four |
| five | six |
*/
Markup.keyboard(["one", "two", "three", "four", "five", "six"], { columns: 2 });
/*
By default, columns count for each string is 4.
| one | two | three |
*/
Markup.keyboard(["one", "two", "three"]);
Create custom button.
Markup.button("Start", "positive", {
foo: "bar"
});
Helper method for create one time keyboard.
Markup.keyboard(["Start", "Help"]).oneTime();
Send keyboard in message box
Markup.keyboard(["test", "Help"]).inline();
Store anything for current user in local memory.
import { VkBot } from "@lildiary/vk-bot";
import Session from "@lildiary/vk-bot/lib/session";
const bot = new VkBot(process.env.TOKEN);
const session = new Session();
bot.use(session.middleware());
bot.on(ctx => {
ctx.session.counter = ctx.session.counter || 0;
ctx.session.counter++;
ctx.reply(`You wrote ${ctx.session.counter} messages.`);
});
bot.startPolling();
key
: Context property name (default: session
)getSessionKey
: Getter for session keygetSessionKey(ctx)
const getSessionKey = ctx => {
const userId = ctx.message.from_id || ctx.message.user_id;
return `${userId}:${userId}`;
};
Scene manager.
import { VkBot, Scene, Session, Stage } from "@lildiary/vk-bot";
const bot = new VkBot(process.env.TOKEN);
const scene = new Scene(
"meet",
ctx => {
ctx.scene.next();
ctx.reply("How old are you?");
},
ctx => {
ctx.session.age = +ctx.message.text;
ctx.scene.next();
ctx.reply("What is your name?");
},
ctx => {
ctx.session.name = ctx.message.text;
ctx.scene.leave();
ctx.reply(
`Nice to meet you, ${ctx.session.name} (${ctx.session.age} years old)`
);
}
);
const session = new Session();
const stage = new Stage(scene);
bot.use(session.middleware());
bot.use(stage.middleware());
bot.command("/meet", ctx => {
ctx.scene.enter("meet");
});
bot.startPolling();
constructor(...scenes)
: Register scenesconstructor(name, ...middlewares)
: Create scene.command(triggers, ...middlewares)
: Create commands for scenectx.scene.enter(name, [step]) // Enter in scene
ctx.scene.leave() // Leave from scene
ctx.scene.next() // Go to the next step in scene
ctx.scene.step // Getter for step in scene
ctx.scene.step= // Setter for step in scene
MIT.
FAQs
🤖 VK bot framework for Node.js, based on Bots Long Poll API, Promises, EventEmmiter and TypeScript
The npm package @lildiary/vk-bot receives a total of 6 weekly downloads. As such, @lildiary/vk-bot popularity was classified as not popular.
We found that @lildiary/vk-bot demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.