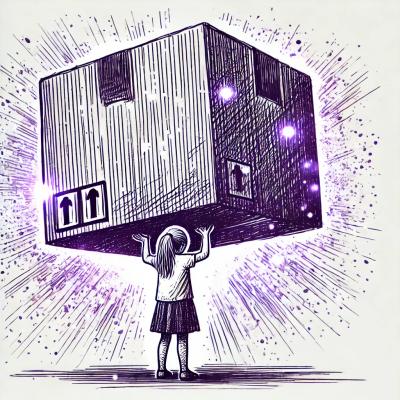
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@littlemissrobot/sass-breakpoints
Advanced tools
Little Miss Robot breakpoints setup for defining breakpoints and applying media queries
This repository contains the logic and functionality to define and insert media queries or breakpoints through the use of a configuration object within SASS. It allows to make use of pre-defined mixins and functions that are only breakpoint related.
This repository does not generate any CSS. It simply makes functions and mixins available to be used in combination with a configuration object to manage your breakpoints. This only works when writing CSS in SASS and is specifically made for that language.
$ npm install --save-dev @littlemissrobot/sass-breakpoints
@import "~@littlemissrobot/sass-breakpoints/lib/main";
$lmr-sass-breakpoints: (
viewport-0: (
width: 0,
),
viewport-3: (
width: 36rem
),
viewport-4: (
width: 48rem
),
viewport-7: (
width: 72rem
),
viewport-9: (
width: 99.2rem
),
viewport-12: (
width: 120rem
)
);
Mixin that applies a media-query to a certain element. This media query can either be placed inside a selector or can wrap around one or multiple selectors. This mixin applies styling to the selector when the viewport is above the passed width. The styling is applied until that breakpoint is reached.
Parameters:
min-width: the minimum width until the breakpoint is reached. The value can be:
// =============================================================================
// Configuration
// =============================================================================
$lmr-sass-breakpoints: (
viewport-7: (
width: 72rem
)
);
// =============================================================================
// Inside selector
// =============================================================================
div {
// Number in pixels
// -------------------------------------------------------------------------
@include respond-at(720px) {
margin: 3rem;
}
// Number is rem
// -------------------------------------------------------------------------
@include respond-at(72rem) {
margin: 3rem;
}
// Name of the breakpoint
// -------------------------------------------------------------------------
@include respond-at("viewport-7") {
margin: 3rem;
}
}
// =============================================================================
// Around selector
// =============================================================================
// Number in pixels
// -----------------------------------------------------------------------------
@include respond-at(720px) {
div {
margin: 3rem;
}
}
// Number is rem
// -----------------------------------------------------------------------------
@include respond-at(72rem) {
div {
margin: 3rem;
}
}
// Name of the breakpoint
// -----------------------------------------------------------------------------
@include respond-at("viewport-7") {
div {
margin: 3rem;
}
}
Mixin that applies a media-query to a certain element. This media query can either be placed inside a selector or can wrap around one or multiple selectors. This mixin applies styling to the selector when the viewport is below the passed width. The styling is applied until that breakpoint is reached.
Parameters:
max-width: the maximum width until the breakpoint is reached. The value can be:
// =============================================================================
// Configuration
// =============================================================================
$lmr-sass-breakpoints: (
viewport-7: (
width: 72rem
)
);
// =============================================================================
// Inside selector
// =============================================================================
div {
// Number in pixels
// -------------------------------------------------------------------------
@include respond-to(720px) {
margin: 3rem;
}
// Number is rem
// -------------------------------------------------------------------------
@include respond-to(72rem) {
margin: 3rem;
}
// Name of the breakpoint
// -------------------------------------------------------------------------
@include respond-to("viewport-7") {
margin: 3rem;
}
}
// =============================================================================
// Around selector
// =============================================================================
// Number in pixels
// -----------------------------------------------------------------------------
@include respond-to(720px) {
div {
margin: 3rem;
}
}
// Number is rem
// -----------------------------------------------------------------------------
@include respond-to(72rem) {
div {
margin: 3rem;
}
}
// Name of the breakpoint
// -----------------------------------------------------------------------------
@include respond-to("viewport-7") {
div {
margin: 3rem;
}
}
Mixin that applies a media-query to a certain element. This media query can either be placed inside a selector or can wrap around one or multiple selectors. This mixin applies styling to the selector when the viewport is between the passed widths.
Parameters:
These parameters can both either be:
* A number in pixels.
* A number in rem. This value is converted automagically to pixels.
* A string, which is the name of the breakpoint defined in
$lmr-sass-breakpoints. This must have a width key. This key can either be
in pixels or in rem.
// =============================================================================
// Configuration
// =============================================================================
$lmr-sass-breakpoints: (
viewport-7: (
width: 72rem
),
viewport-9: (
width: 96rem
)
);
// =============================================================================
// Inside selector
// =============================================================================
div {
// Number in pixels
// -------------------------------------------------------------------------
@include respond-between(720px, 960px) {
margin: 3rem;
}
// Number is rem
// -------------------------------------------------------------------------
@include respond-between(72rem, 96rem) {
margin: 3rem;
}
// Name of the breakpoint
// -------------------------------------------------------------------------
@include respond-between("viewport-7", "viewport-9") {
margin: 3rem;
}
// Combined
// -------------------------------------------------------------------------
@include respond-between(720px, 96rem) {
margin: 3rem;
}
@include respond-between("viewport-7", 960px) {
margin: 3rem;
}
@include respond-between(72rem, "viewport-9") {
margin: 3rem;
}
}
// =============================================================================
// Around selector
// =============================================================================
// Number in pixels
// -----------------------------------------------------------------------------
@include respond-between(720px, 960px) {
div {
margin: 3rem;
}
}
// Number is rem
// -----------------------------------------------------------------------------
@include respond-between(72rem, 96rem) {
div {
margin: 3rem;
}
}
// Name of the breakpoint
// -----------------------------------------------------------------------------
@include respond-between("viewport-7", "viewport-9") {
div {
margin: 3rem;
}
}
// Combined
// -----------------------------------------------------------------------------
@include respond-between(720px, 96rem) {
div {
margin: 3rem;
}
}
@include respond-between("viewport-7", 960px) {
div {
margin: 3rem;
}
}
@include respond-between(72rem, "viewport-9") {
div {
margin: 3rem;
}
}
Function that returns the value of the width key defined within the a breakpoint in the $lmr-sass-breakpoints map. These can be used to retrieve the value and make a calculation or can be used with other media queries.
Parameters:
// =============================================================================
// Configuration
// =============================================================================
$lmr-sass-breakpoints: (
viewport-7: (
width: 72rem
),
viewport-9: (
width: 960px
)
);
// =============================================================================
// Function
// =============================================================================
// Returns 72rem
get-width("viewport-7");
// Returns 960px
get-width("viewport-9");
// =============================================================================
// With media queries
// =============================================================================
div {
// same as respond-at("viewport-9")
@include respond-at(get-width("viewport-9")) {
margin: 3rem;
}
// same as respond-to("viewport-7")
@include respond-to(get-width("viewport-7")) {
margin: 3rem;
}
// same as respond-between("viewport-7", "viewport-9")
@include respond-between(get-width("viewport-7"), get-width("viewport-9")) {
margin: 3rem;
}
}
FAQs
Little Miss Robot breakpoints setup for defining breakpoints and applying media queries.
The npm package @littlemissrobot/sass-breakpoints receives a total of 34 weekly downloads. As such, @littlemissrobot/sass-breakpoints popularity was classified as not popular.
We found that @littlemissrobot/sass-breakpoints demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.