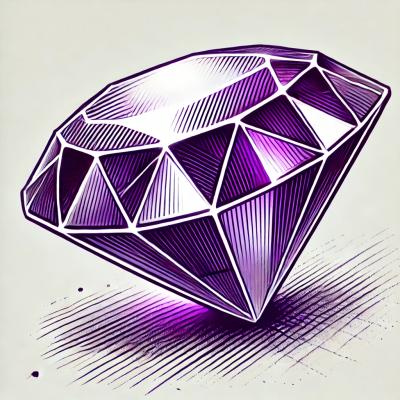
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@littlemissrobot/sass-functions
Advanced tools
Little Miss Robot sass functions library that helps execute reusable and complex tasks.
This package contains Sass (Dart Sass) based functions that we, at Little Miss Robot, like to use to make our wonderful lives in the world of SASS more wondeful. These functions are also mainly used throughout our other libraries to manage recurring tasks.
This package does not contain or generate any CSS. It simply provides a couple
of @function
statements for you to make use of.
Make use of Dart Sass:
This library makes use of Dart Sass, which is the primary implementation of Sass. Make sure that your Sass compiler is making use of Dart Sass.
Generate only what you need:
This library generates classes based on your configuration. The larger the configuration, the more css, the larger the CSS file. DO NOT enable all the config options, but only the ones you actually use and need!
# As a dependency
$ npm install @littlemissrobot/sass-functions
# As a dev-dependency
$ npm install --save-dev @littlemissrobot/sass-functions
@use "YOUR-PATH-TO-NODE_MODULES/@littlemissrobot/sass-functions" as _functions;
_functions
namespace:h1 {
margin-bottom: _functions.math_px-rem(16px);
}
map
are namespaced with
_functions.map_[FUNCTION-NAME]
or a list
would be
_functions.list_[FUNCTION-NAME]
.@use "YOUR-PATH-TO-NODE_MODULES/@littlemissrobot/sass-functions/lib/math" as _math;
_math
namespace:h1 {
margin-bottom: _math.px-rem(16px);
}
That's (mind-blowingly) it! There are a number of partials to use the functions from:
"sass-functions/lib/list" as _list;
"sass-functions/lib/map" as _map;
"sass-functions/lib/math" as _math;
"sass-functions/lib/number" as _number;
"sass-functions/lib/string" as _string;
These functions are namespace with list_:
@use "@littlemissrobot/sass-functions" as _functions;
_functions.list_includes($list, 5);
Or can be included through the partial:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
_list.includes($list, 5);
Checks if the list contains a certain item. Returns a boolean.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list: (1, 2, 3, 4, 5);
_list.includes($list, 5); // true
_list.includes($list, 10); // false
Checks if the numbers in the list are ordered lowest to highest.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list1: (2, 1, 3);
$list2: (1, 2, 3);
_list.is-numbers-order-low-high($list1); // false
_list.is-numbers-order-low-high($list2); // true
Merges multiple lists and removes any duplicates.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list1: (1, 2, 3);
$list2: (1, 2, 3, 4, 5);
$list3: (4, 5, 6, 7, 8);
_list.merge-uniques($list1, $list2, $list3); // (1, 2, 3, 4, 5, 6, 7, 8)
Loops through the list and removes duplicate values.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list: (1, 2, 3, 1, 3, 4, 5);
_list.remove-duplicates($list); // (1, 2, 3, 4, 5)
Removes a list of values from the passed list.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list: (1, 2, 3, 4, 5);
$values: (1, 2);
_list.remove-values($list, $values); // (3, 4, 5)
Removes a value from the passed list.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list: (1, 2, 3, 4, 5);
$value: 1;
_list.remove-value($list, $value); // (2, 3, 4, 5)
Reverses the order of the values within a list.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list: (1, 2, 3, 4, 5);
_list.reverse($list); // (5, 4, 3, 2, 1)
Sorts a list of numbers from lowest to highest.
Parameters:
@use "@littlemissrobot/sass-functions/lib/list" as _list;
$list: (2, 1, 5, 3, 4);
_list.order-numbers-low-high($list); // (1, 2, 3, 4, 5)
These functions are namespace with map_:
@use "@littlemissrobot/sass-functions" as _functions;
_functions.map_includes($map, color);
Or can be included through the partial:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
_map.includes($map, color);
The standard map-merge function only lets you merge 2 maps. This function makes use of the method, but merges as many maps together as you want.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$map1: (
display: flex;
justify-content: center,
align-items: center,
);
$map2: (
font-size: 16px,
line-height: 24px,
);
$map3: (
color: white,
background-color: black,
);
_map.collect($map1, $map2, $map3);
// result: (
// display: flex;
// justify-content: center,
// align-items: center,
// font-size: 16px,
// line-height: 24px,
// color: white,
// background-color: black,
// );
Retrieve a value from a map which can contain mulitple more maps with values. Pass a path as a string, where each key is seperated by a space. Each key within that string represents the keys until you reach a certain value within the map.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$colors: (
brand: (
primary: black,
secondary: white
),
typo: (
title: (
h1: black,
h2: red,
h3: blue
),
text: (
p: black,
small: (
base: gray,
inverse: white,
),
)
)
);
_map.get($colors, "brand primary"); // black
_map.get($colors, "typo title h1"); // black
_map.get($colors, "typo text small base"); // gray
Generate a new map of items, based on the values within the list, which represent the keys in the map.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$breakpoints: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
);
$list1: (viewport-7, viewport-9);
$list2: (viewport-3, viewport-9, viewport-12);
_map.filter-by-list($breakpoints, $list1);
// result: (
// viewport-7: 720px,
// viewport-9: 992px,
// );
_map.filter-by-list($breakpoints, $list2);
// result: (
// viewport-3: 360px,
// viewport-9: 992px,
// viewport-12: 1200px,
// );
Generate a new list of keys from a map, based on the items within a map and a list representing the values in that map.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$breakpoints: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
);
$list1: (720px, 992px);
$list2: (360px, 992px, 1200px);
_map.filter-keys-by-list($breakpoints, $list1);
// result: (viewport-7, viewport-9);
_map.filter-keys-by-list($breakpoints, $list2);
// result: (viewport-3, viewport-9, viewport-12);
Generate a new list of values from a map, based on the items within a map and a list representing the keys in that map.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$breakpoints: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
);
$list1: (viewport-7, viewport-9);
$list2: (viewport-3, viewport-9, viewport-12);
_map.filter-values-by-list($breakpoints, $list1);
// result: (720px, 992px);
_map.filter-values-by-list($breakpoints, $list2);
// result: (360px, 992px, 1200px);
Checks if a map contains a certain key.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$breakpoints: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
);
_map.includes($breakpoints, viewport-7); // true
_map.includes($breakpoints, viewport-2); // false
Checks if the numbers, as values, in the map are ordered lowest to highest.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$map1: (
viewport-7: 720px,
viewport-3: 360px,
viewport-9: 960px
);
$map2: (
viewport-3: 360px,
viewport-7: 720px,
viewport-9: 960px
);
_map.is-numbers-order-low-high($map1); // false
_map.is-numbers-order-low-high($map2); // true
Merge 2 maps together, but respect and preserve the order of the keys in $parent-map and attack any unique keys at the end.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$map1: (
font-size: 16px,
line-height: 24px,
);
$map2: (
line-height: 30px,
font-size: 24px,
);
_map.none-destructive-merge($map1, $map2);
Reverses the order of the items within a map.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$breakpoints: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
);
_map.reverse($breakpoints);
// result: (
// viewport-12: 1200px
// viewport-9: 992px,
// viewport-7: 720px,
// viewport-4: 480px,
// viewport-3: 360px,
// );
Trims a map to a certain target key. Returns a new map that is trimmed down until the key is met.
Parameters:
@use "@littlemissrobot/sass-functions/lib/map" as _map;
$breakpoints: (
viewport-3: 360px,
viewport-4: 480px,
viewport-7: 720px,
viewport-9: 992px,
viewport-12: 1200px
);
_map.trim($breakpoints, viewport-7);
// result: (
// viewport-3: 360px,
// viewport-4: 480px,
// viewport-7: 720px
// );
These functions are namespace with math_:
@use "@littlemissrobot/sass-functions" as _functions;
_functions.math_pow(8, 2);
Or can be included through the partial:
@use "@littlemissrobot/sass-functions/lib/math" as _math;
_math.pow(8, 2);
Round a number's value after the comma (decimals) to a certain amount.
Parameters:
@use "@littlemissrobot/sass-functions/lib/math" as _math;
_math.round-decimal(1.12345, 2); // 1.12
_math.round-decimal(1.123456789, 5); // 1.12346
Power function / exponent operator which accepts positive, negative (integer, float) exponents.
Parameters:
@use "@littlemissrobot/sass-functions/lib/math" as _math;
_math.pow(2, 8); // 256
_math.pow(4, 2); // 16
Generate a random number between a minimum and maximum value.
Parameters:
@use "@littlemissrobot/sass-functions/lib/math" as _math;
_math.randomize(0, 10); // 4
_math.randomize(0, 10); // 5
_math.randomize(2, 8); // 6
_math.randomize(2, 8); // 7
These functions are namespace with number_:
@use "@littlemissrobot/sass-functions" as _functions;
_functions.number_strip-unit(16px);
Or can be included through the partial:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.strip-unit(16px);
Check if a number is of type integer (no decimals).
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.is-integer("sass-functions"); // false
_number.is-integer(20); // true
_number.is-integer(20.5); // false
Removes the unit from a value.
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.strip-unit(10px); // 10
_number.strip-unit(7rem); // 7
Checks whether or not the passed value is a number. Very similar to is-integer, but this value can have decimals.
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.is-number(10px); // true
_number.is-number(7rem); // true
_number.is-number(2.5px); // true
_number.is-number("sass-functions"); // false
_number.is-number(center); // false
Converts a pixel value to em.
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.px-em(16px); // 1em
_number.px-em(10px, 16px); // 0.625em
_number.px-em(32px); // 2em
_number.px-em(20px, 10px); // 2em
Converts a pixel value to rem.
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.px-rem(16px); // 1rem
_number.px-rem(10px, 16px); // 0.625rem
_number.px-rem(32px); // 2rem
_number.px-rem(20px, 10px); // 2rem
Converts a rem value to px.
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.rem-px(1rem); // 16px
_number.rem-px(2rem); // 32px
_number.rem-px(1rem, 10px); // 10px
_number.rem-px(2rem, 10px); // 20px
Converts a value to a value in px
. Supported conversions (at the moment) are:
rem
to px
em
to px
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.rem(16px, 16px); // 1rem
_number.rem(32px); // 2rem
Converts a value to a value in rem
. Supported conversions (at the moment) are:
px
to rem
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.rem(16px, 16px); // 1rem
_number.rem(32px); // 2rem
Converts a value to a value in em
. Supported conversions (at the moment) are:
px
to em
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.em(16px, 16px); // 1em
_number.em(32px); // 2em
Converts a value from unit value to another. Supported conversions (at the moment) are:
Parameters:
@use "@littlemissrobot/sass-functions/lib/number" as _number;
_number.convert(16px, rem, 16px); // 1rem
_number.convert(1rem, px, 16px); // 16px
These functions are namespace with string_:
@use "@littlemissrobot/sass-functions" as _functions;
_functions.string_includes("Functions", "F");
Or can be included through the partial:
@use "@littlemissrobot/sass-functions/lib/string" as _string;
_string.includes("Functions", "F");
Escapes a value and adds extra slashes to special characters. This can be useful for creating a class with special characters.
Parameters:
@use "@littlemissrobot/sass-functions/lib/string" as _string;
_string.escape(100%); // 100\%
_string.escape(l-grid__col:12/12); // l-grid__col\:12\/12
Check if string contains certain characters. Returns a boolean.
Parameters:
@use "@littlemissrobot/sass-functions/lib/string" as _string;
_string.escape(100%); // 100\%
_string.escape(l-grid__col:12/12); // l-grid__col\:12\/12
Replace a certain part of a string by another string.
Parameters:
@use "@littlemissrobot/sass-functions/lib/string" as _string;
_string.replace("sass-functions", "sass", "dart-sass"); // dart-sass-functions
Create a list from a string by defining a character to split the string at.
Parameters:
@use "@littlemissrobot/sass-functions/lib/string" as _string;
_string.split("sass-functions", "-"); // (sass, functions)
Converts a value to a string
Parameters:
@use "@littlemissrobot/sass-functions/lib/string" as _string;
_string.to(10px); // "10px"
FAQs
Little Miss Robot sass functions library that helps execute reusable and complex tasks.
The npm package @littlemissrobot/sass-functions receives a total of 26 weekly downloads. As such, @littlemissrobot/sass-functions popularity was classified as not popular.
We found that @littlemissrobot/sass-functions demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.