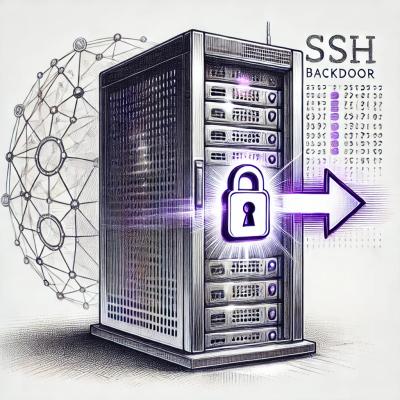
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@lottiefiles/lottie-interactivity
Advanced tools
This is a small effects and interactivity library written to be paired with the Lottie Web Player
This is a small library to add scrolling and cursor interactivity to your Lottie Animations. This can be used with the Lottie Web-Player Component or the Lottie Player.
yarn add @lottiefiles/lottie-interactivity
npm install --save @lottiefiles/lottie-interactivity
<script src="https://unpkg.com/@lottiefiles/lottie-interactivity@latest/dist/lottie-interactivity.min.js"></script>
If you would like to take a look at some examples, feel free to have a look in the examples folder to see how each of the Lotties have been implemented.
<script src="https://unpkg.com/@lottiefiles/lottie-player@0.4.0/dist/lottie-player.js"></script> // place this in your body element
<lottie-player
id="firstLottie"
src="https://assets2.lottiefiles.com/packages/lf20_i9mxcD.json"
style="width:400px; height: 400px;"
>
</lottie-player>
The name of the player ie: 'firstLottie' in this example is the ID set to the lottie web component on the html page. Configration will contain an actions object. This object takes an array named actions which consists of an array of objects. Multiple objects can be added into this array and therefore multiple actions such as "seek","play", "stop" and "loop", can be set.
Each object has a start and end which is essentially a percentage for the height of the lottie container and is a value between 0 and 1. The visibility arrays first value is the start and the second value is the end. This refers to the percentage of the viewport.
Ensure that the ending frame is the frame you want the interactivity to end at. This could be the last frame or a frame of your choosing. In this case it is set to 100.
Configuration modes include "scroll" where the animation will be synced to the scrolling of the window and "cursor" where the scrolling of the animation will be synced to the cursor position within the container.
The configuration can include a container field as shown in the next example. If a container is not provided the parent div will be taken as a container.
ensure that the interactivity library is imported to the body of your html DOM
LottieInteractivity.create({
mode: 'scroll',
player: '#firstLottie',
actions: [
{
visibility: [0, 1],
type: 'seek',
frames: [0, 100],
},
],
});
The configuration for the library remains the same for react apps. However usage and initialization is as follows. Import the create function from the lottie interactivity library and call the create function. With frameworks like react it is ideal to add an event listener that waits for the lottie player to load before calling the interactivity library. An example is as follows for a very basic react class component.
import React from 'react';
import '@lottiefiles/lottie-player';
import { create } from '@lottiefiles/lottie-interactivity';
class App extends React.Component {
constructor(props) {
super(props);
this.myRef = React.createRef(); // 1. create a reference for the lottie player
}
componentDidMount() {
// 3. listen for player load. see lottie player repo for other events
this.myRef.current.addEventListener('load', function (e) {
// 4. configure the interactivity library
create({
mode: 'scroll',
player: '#firstLottie',
actions: [
{
visibility: [0, 1],
type: 'seek',
frames: [0, 100],
},
],
});
});
}
render() {
return (
<div className="App">
<div style={{ height: '400px' }}></div>
<lottie-player
ref={this.myRef} // 2. set the reference for the player
id="firstLottie"
controls
mode="normal"
src="https://assets3.lottiefiles.com/packages/lf20_UJNc2t.json"
style={{ width: '320px' }}
></lottie-player>
</div>
);
}
}
export default App;
The configuration for the library remains the same for vue apps. However usage and initialization is as follows. Import the create function from the lottie interactivity library and call the create function. With frameworks like vue it is ideal to add an event listener that waits for the lottie player to load before calling the interactivity library. An example is as follows for a very basic vue class component.
<template>
<!-- 1. Create a lottie player with a reference -->
<lottie-player id="firstLottie"
ref="lottie"
controls
mode="normal"
src="https://assets3.lottiefiles.com/packages/lf20_UJNc2t.json"
style="width: 320px;">
</lottie-player>
</template>
<script>
import '@lottiefiles/lottie-player';
import { create } from '@lottiefiles/lottie-interactivity';
export default {
name: 'App',
mounted() {
// 2. listen for player load. See lottie player repo for other events
this.$refs.lottie.addEventListener('load', function() {
// 3. configure the interactivity library
create({
mode: 'scroll',
player: '#firstLottie',
actions: [
{
visibility: [0, 1],
type: 'seek',
frames: [0, 100],
},
],
});
})
}
}
</script>
There may be situations where you would like to wrap the lottie player inside a container or just in general sync the lottie scroll with a div on your page. In which case you may pass a container variable with the container id into the action object as shown below. The scroll effect in this case will be activate once the "MyContainerId" is in viewport.
LottieInteractivity.create({
player: '#secondLottie',
container: '#MyContainerId',
mode: 'scroll',
actions: [
{
visibility: [0, 1.0],
type: 'seek',
frames: [0, 100],
},
],
});
If you would like to add an offset to the top of the container or player you may add an extra action object to the array. As per the example config below, from 0 to 30% visibility of the container, the lottie will be stopped and from 30% to 100% visibility of the container the lottie will be synced with the scroll.
LottieInteractivity.create({
player: '#firstLottie',
mode: 'scroll',
actions: [
{
visibility: [0, 0.3],
type: 'stop',
frames: [0],
},
{
visibility: [0.3, 1],
type: 'seek',
frames: [0, 100],
},
],
});
In cases where you would like the animation to loop from a specific frame to a specific frame, you can add an additional object to actions in which you can specify the frames. In the example below, the lottie loops frame 150 to 300 once 45% of the container is reached.
LottieInteractivity.create({
player: '#firstLottie',
mode: 'scroll',
actions: [
{
visibility: [0, 0.3],
type: 'stop',
frames: [0],
},
{
visibility: [0.3, 0.45],
type: 'seek',
frames: [0, 150],
},
{
visibility: [0.45, 1],
type: 'loop',
frames: [150, 300],
},
],
});
If you would like to play the animation and loop it only from a certain frame to a certain frame of your choosing, then you can utilize the loop action and frames variable. The config below shows this example.
LottieInteractivity.create({
player: '#firstLottie',
mode: 'scroll',
actions: [
{
visibility: [0.45, 1],
type: 'loop',
frames: [17, 63],
},
],
});
To play the animation on hover you can pair the cursor mode with the play action. You may even utilize this to stop the animation on hover via the "stop" type action instead of "play". Available cursor actions are "loop","seek","play" and "stop".
LottieInteractivity.create({
player: '#firstLottie',
mode: 'cursor',
actions: [
{
position: { x: [0, 1], y: [0, 1] },
type: 'loop',
frames: [50, 139],
},
],
});
To sync the position of the cursor with the frames of the animation , you will need to add a position object to the action. This tells the library which position in the container that the animation should end at. As you move the cursor along the given container (No container provided in this example and the library takes the wrapper div for the Lottie as the container) the frames will move according to the cursors position.
LottieInteractivity.create({
player: '#firstLottie',
mode: 'cursor',
actions: [
{
position: { x: [0, 1], y: [0, 1] },
type: 'seek',
frames: [0, 100],
},
],
});
The cursor sync function can be used to either sync with the horizontal movement of the cursor or the vertical movement of the cursor. This example shows the horizontal sync.
LottieInteractivity.create({
player: '#firstLottie',
mode: 'cursor',
actions: [
{
position: { x: [0, 1], y: [-1, 2] },
type: 'seek',
frames: [0, 179],
},
{
position: { x: -1, y: -1 },
type: 'stop',
frames: [0],
},
],
});
Play a Lottie when clicked on. Clicking multiple times won't restart the animation if its already playing. However if you want the animation to restart as soon as it's clicked on set the 'forceFlag' property to true.
LottieInteractivity.create({
player:'#firstLottie',
mode:"cursor",
actions: [{
type: "click",
forceFlag: false
}]
});
Play a Lottie when hovered. Hovering multiple times won't restart the animation if its already playing. However if you want the animation to restart as soon as it's hovered on set the 'forceFlag' property to true.
LottieInteractivity.create({
player:'#firstLottie',
mode:"cursor",
actions: [{
type: "hover",
forceFlag: false
}]
});
Toggle a Lottie when clicked on.
LottieInteractivity.create({
player:'#toggleLottie',
mode: "cursor",
actions: [{
type: "toggle",
}]
});
Play a Lottie when it is visible. Visibility can be customised to play the animation when a percentage of the container is reached. Here [0.50, 1.0] means the animation will play when 50% of the container is reached, [0, 1.0] will play as soon as the container is visible.
LottieInteractivity.create({
mode:"cursor",
player:'#firstLottie',
actions: [{
visibility: [0.50, 1.0],
type: "play"
}]
});
The animation will play when the cursor is placed over the animation, and in reverse if the cursor leaves the animation.
LottieInteractivity.create({
mode:"cursor",
player:'#firstLottie',
actions: [{
type: "hold"
}]
});
The animation will play when the cursor is placed over the animation, and pause if the cursor leaves the animation.
LottieInteractivity.create({
mode:"cursor",
player:'#fourteenthLottie',
actions: [{
type: "pauseHold"
}]
});
When using this mode you now have the power to chain different segments of animations depending on how the user interacts (click x amount of times, hover..) with the segment but also with Lottie events (onComplete). By using the 'chain' mode Lottie-interactivity can even load separate animations in succession depending on the interaction.
In this example 3 segments are present. The pigeon running on loop, an explosion when the user clicks and the feathers falling once the explosion has finished.
LottieInteractivity.create({
mode:"chain",
player:'#explodingBird',
actions: [
{
state: 'loop',
transition: 'click',
frames: 'bird'
},
{
state: 'autoplay',
transition: 'onComplete',
frames: 'explosion'
},
{
state: 'autoplay',
transition: 'onComplete',
frames: 'feathers',
reset: true
}
],
});
The name of the player ie: 'explodingBird' in this example is the ID set to the lottie-player component on the html page. Interaction chaining can be activated by using the 'chain' mode. An 'actions' array serves to configure each segment and how to interact with it. Multiple objects can be added into this array and therefore multiple different ways to interact and transit through different segments of an animation.
Each object in the actions array should have a 'state' and 'transition' property.
State defines how the segment of the animation will be playing when loaded and waiting for an interaction.
State can have the following values:
Plays the animation once on load.
Loops the animation.
Optionally a 'loop' property can be defined to loop x amount of times
Plays the animation on click.
Plays the animation on hover.
Animation won't play
Transition defines the interaction that will cause Lottie-Interactivity to go to the next interaction link in the chain.
Transition can have the following values:
Causes a transition when clicking on the animation is detected.
Optionally a 'count' property can be defined to transit after x amount of clicks
Causes a transition when hovering over the animation is detected.
Optionally a 'count' property can be defined to transit after x amount of hovers
Play the animation x amount of times before transiting.
A 'repeat' property containing a number can then be used to define how many times the animation will repeat before transiting
Hover over the animation for the length of the 'frames' property to cause a transition. If the cursor leaves the animation, it plays in reverse.
Hover over the animation for the length of the 'frames' property to cause a transition. If the cursor leaves the animation, it pauses.
Sync animation with cursor position. A 'position' object will be needed as well as a 'frames' array.
When the animation has finished playing the defined segment, a transition will occur.
Animation won't transit.
Each link in the interaction chain can have a defined segment of frames to play. The 'frames' array's first value is the start and the second value is the end. If you don't like defining frame numbers, named markers can also be used. More information about named markers here.
If no frames are provided the entirety of the animation is played.
A 'path' property can be used to define where to load the animation from.
Jumps to the action defined at the submitted index after the necessary interaction is detected.
Useful for the last action, if true will go back to the first action. The 'transition' event is fired from the lottie-player element every time a transition occurs. The event contains the following details: oldIndex newIndex
If true, click and hover interactions will play straight away. Otherwise, will ignore if animation is already playing.
Will delay all interactions and playback of the animation until the delay is finished.
Set the speed of the animation, 1 being the default speed.
FAQs
This is a small effects and interactivity library written to be paired with the Lottie Web Player
The npm package @lottiefiles/lottie-interactivity receives a total of 11,051 weekly downloads. As such, @lottiefiles/lottie-interactivity popularity was classified as popular.
We found that @lottiefiles/lottie-interactivity demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.