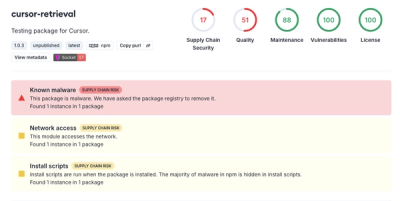
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@lukeed/csprng
Advanced tools
An alias package for `crypto.randomBytes` in Node.js and/or browsers
@lukeed/csprng is a lightweight and fast library for generating cryptographically secure random numbers. It is designed to be simple and efficient, making it suitable for use in various applications where secure random number generation is required.
Generate a random number
This feature allows you to generate a cryptographically secure random number of a specified byte length. The example code generates a 16-byte random number and logs it to the console.
const { random } = require('@lukeed/csprng');
const randomNumber = random(16); // Generates a 16-byte random number
console.log(randomNumber);
Generate a random number with a specific length
This feature allows you to specify the length of the random number in bytes. The example code generates a 32-byte random number and logs it to the console.
const { random } = require('@lukeed/csprng');
const randomNumber = random(32); // Generates a 32-byte random number
console.log(randomNumber);
The 'crypto' module is a built-in Node.js module that provides cryptographic functionality, including a method for generating cryptographically secure random numbers. It is more comprehensive than @lukeed/csprng, offering a wide range of cryptographic operations.
The 'uuid' package is used for generating RFC4122 UUIDs (Universally Unique Identifiers). While it is not specifically designed for generating random numbers, it uses cryptographically secure random number generation under the hood for creating UUIDs.
The 'randombytes' package is a simple library for generating cryptographically secure random bytes. It is similar to @lukeed/csprng in terms of functionality but is more focused on generating random bytes rather than numbers.
A tiny (~90B) isomorphic wrapper for
crypto.randomBytes
in Node.js and browsers.
Why?
This package allows you/dependents to import a cryptographically secure generator (CSPRNG) without worrying about (aka, checking the runtime environment for) the different crypto
implementations. Instead, by extracting a random
function into a third-party/external package, one can rely on bundlers and/or module resolution to load the correct implementation for the desired environment.
In other words, one can include the browser-specific implementation when bundling for the browser, completely ignoring the Node.js code – or vice versa.
By default, this module is set up to work with Rollup, webpack, and Node's native ESM and CommonJS path resolutions.
$ npm install --save @lukeed/csprng
General Usage
// Rely on bundlers/environment detection
import { random } from '@lukeed/csprng';
const array = random(12);
// browser => Uint8Array(12) [...]
// Node.js => <Buffer ...>
Specific Environment
// Choose the "browser" implementation explicitly.
//=> ! NOTE ! Will break in Node.js environments!
import { random } from '@lukeed/csprng/browser';
const array = random(1024);
//=> Uint8Array(1024) [...]
// ---
// Choose the "node" implementation explicitly.
//=> ! NOTE ! Will break in browser environments!
import { random } from '@lukeed/csprng/node';
const array = random(1024);
//=> <Buffer ...>
Returns: Buffer
or Uint8Array
Returns a typed array of given length
.
Type: Number
The desired length of your output TypedArray.
MIT © Luke Edwards
FAQs
An alias package for `crypto.randomBytes` in Node.js and/or browsers
We found that @lukeed/csprng demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.