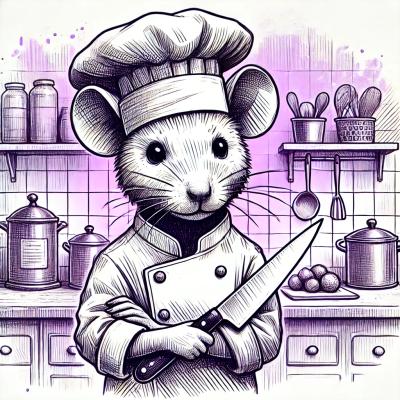
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@lumir-company/admin-server-api
Advanced tools
관리자 시스템을 위한 API SDK 패키지입니다.
npm install @lumir-company/admin-server-api
import { AuthAPI } from '@lumir-company/admin-server-api';
const authApi = new AuthAPI('http://your-api-url');
// 로그인
const token = await authApi.login({
accountId: 'your-id',
password: 'your-password',
});
import { NoticeAPI } from '@lumir-company/admin-server-api';
const noticeApi = new NoticeAPI('http://your-api-url');
noticeApi.setToken(token.accessToken);
// 공지사항 목록 조회
const { data } = await noticeApi.getNotices(1, 10);
// 공지사항 상세 조회
const notice = await noticeApi.getNoticeById('notice-id');
import { NewsAPI } from '@lumir-company/admin-server-api';
const newsApi = new NewsAPI('http://your-api-url');
newsApi.setToken(token.accessToken);
// 뉴스 목록 조회
const { data } = await newsApi.getNews(1, 10);
// 뉴스 상세 조회
const news = await newsApi.getNewsById('news-id');
import { PressReleaseAPI } from '@lumir-company/admin-server-api';
const pressReleaseApi = new PressReleaseAPI('http://your-api-url');
pressReleaseApi.setToken(token.accessToken);
// 보도자료 목록 조회
const { data } = await pressReleaseApi.getPressReleases(1, 10);
// 보도자료 상세 조회
const pressRelease =
await pressReleaseApi.getPressReleaseById('press-release-id');
import { S3API } from '@lumir-company/admin-server-api';
const s3Api = new S3API('http://your-api-url');
s3Api.setToken(token.accessToken);
// 파일 다운로드
const fileResponse = await s3Api.getFile('file-key', true);
import { PageViewAPI, PageName } from '@lumir-company/admin-server-api';
export enum PageName {
MAIN = '메인 페이지',
DISCLOSURE = '전자공고',
SHAREHOLDER = '주주정보',
NEWS = '루미르스토리',
VIDEO = '영상',
BROCHURE = '정보자료',
}
const pageViewApi = new PageViewAPI('http://your-api-url');
pageViewApi.setToken(token.accessToken);
// 페이지 진입 시 기록
await pageViewApi.createPageView({
sessionId: 'user-session-id',
pageName: PageName.MAIN,
enterTime: new Date(),
url: 'https://lumir.space/',
title: '주식상장 이야기', // 페이지 Title
});
// 페이지 이탈 시 업데이트
await pageViewApi.updatePageView({
sessionId: 'user-session-id',
pageName: PageName.MAIN,
exitTime: new Date(),
stayDuration: 60000, // 밀리초 단위
url: 'https://lumir.space/',
title: '주식상장 이야기', // 페이지 Title
});
import { ShareholderMeetingAPI } from '@lumir-company/admin-server-api';
const shareholderMeetingApi = new ShareholderMeetingAPI('http://your-api-url');
shareholderMeetingApi.setToken(token.accessToken);
// 주주총회 목록 조회
const { data } = await shareholderMeetingApi.getMeetings(1, 10);
// 주주총회 상세 조회
const meeting = await shareholderMeetingApi.getMeetingById('meeting-id');
import { DisclosureAPI } from '@lumir-company/admin-server-api';
const disclosureApi = new DisclosureAPI('http://your-api-url');
disclosureApi.setToken(token.accessToken);
// 전자공고 목록 조회
const { data } = await disclosureApi.getDisclosures(1, 10);
// 전자공고 상세 조회
const disclosure = await disclosureApi.getDisclosureById('disclosure-id');
import { VideoAPI } from '@lumir-company/admin-server-api';
const videoApi = new VideoAPI('http://your-api-url');
videoApi.setToken(token.accessToken);
// 비디오 목록 조회
const { data } = await videoApi.getVideos(1, 10);
// 비디오 상세 조회
const video = await videoApi.getVideoById('video-id');
import { BrochureAPI } from '@lumir-company/admin-server-api';
const brochureApi = new BrochureAPI('http://your-api-url');
brochureApi.setToken(token.accessToken);
// 브로슈어 목록 조회
const { data } = await brochureApi.getBrochures(1, 10);
// 브로슈어 상세 조회
const brochure = await brochureApi.getBrochureById('brochure-id');
login({ accountId, password })
: 로그인하여 토큰을 반환setToken(token)
: API 요청에 사용할 토큰 설정getNotices(page, limit)
: 공지사항 목록 조회getNoticeById(id)
: 특정 공지사항 조회setToken(token)
: API 요청에 사용할 토큰 설정getNews(page, limit)
: 뉴스 목록 조회getNewsById(id)
: 특정 뉴스 조회setToken(token)
: API 요청에 사용할 토큰 설정getPressReleases(page, limit)
: 보도자료 목록 조회getPressReleaseById(id)
: 특정 보도자료 조회setToken(token)
: API 요청에 사용할 토큰 설정getFile(key, download)
: 파일 다운로드/조회createPageView(data)
: 페이지뷰 데이터 생성updatePageView(data)
: 페이지뷰 데이터 업데이트 (종료 시간 및 체류 시간)setToken(token)
: API 요청에 사용할 토큰 설정getMeetings(page, limit)
: 주주총회 목록 조회getMeetingById(id)
: 특정 주주총회 조회setToken(token)
: API 요청에 사용할 토큰 설정getDisclosures(page, limit)
: 전자공고 목록 조회getDisclosureById(id)
: 특정 전자공고 조회setToken(token)
: API 요청에 사용할 토큰 설정getVideos(page, limit)
: 비디오 목록 조회getVideoById(id)
: 특정 비디오 조회setToken(token)
: API 요청에 사용할 토큰 설정getBrochures(page, limit)
: 브로슈어 목록 조회getBrochureById(id)
: 특정 브로슈어 조회파일 정보를 나타내는 인터페이스
interface FileInfo {
fileName: string; // 파일 이름
filePath: string; // 파일 경로
}
API 응답의 기본 형태
interface Response<T> {
result: 'success' | 'fail'; // 응답 결과
message: string; // 응답 메시지
status: number; // HTTP 상태 코드
data: T; // 응답 데이터
}
페이지네이션된 목록 응답
interface PaginationResponse<T> {
items: T[]; // 아이템 목록
total: number; // 전체 아이템 수
page: number; // 현재 페이지
limit: number; // 페이지당 아이템 수
hasMore: boolean; // 다음 페이지 존재 여부
}
주주총회 정보
interface ShareholderMeeting {
_id: string;
title: string; // 제목
content: string; // 내용
date: string; // 개최일
isPublic: boolean; // 공개 여부
files: FileInfo[]; // 첨부파일
order: number; // 정렬 순서
createdAt: string; // 생성일
updatedAt: string; // 수정일
}
전자공고 정보
interface Disclosure {
_id: string;
title: string; // 제목
content: string; // 내용
date: string; // 공고일
isPublic: boolean; // 공개 여부
files: FileInfo[]; // 첨부파일
order: number; // 정렬 순서
createdAt: string; // 생성일
updatedAt: string; // 수정일
}
비디오 카테고리 정보
interface Category {
_id: string;
name: string; // 카테고리명
createdAt: string; // 생성일
updatedAt: string; // 수정일
}
비디오 정보
interface Video {
_id: string;
title: string; // 제목
description?: string; // 설명
link: string; // 비디오 링크
files: FileInfo[]; // 첨부파일
isPublic: boolean; // 공개 여부
category: Category; // 카테고리
order: number; // 정렬 순서
createdAt: string; // 생성일
updatedAt: string; // 수정일
}
브로슈어 정보
interface Brochure {
_id: string;
title: string; // 제목
description?: string; // 설명
files: FileInfo[]; // 첨부파일
isPublic: boolean; // 공개 여부
order: number; // 정렬 순서
createdAt: string; // 생성일
updatedAt: string; // 수정일
}
페이지 식별자 열거형
enum PageName {
MAIN = '메인 페이지',
DISCLOSURE = '전자공고',
SHAREHOLDER = '주주정보',
NEWS = '루미르스토리',
VIDEO = '영상',
BROCHURE = '정보자료',
}
페이지뷰 추적 정보
interface PageView {
_id: string;
sessionId: string; // 세션 ID
pageName: PageName; // 페이지 식별자
enterTime: Date; // 진입 시간
exitTime?: Date; // 이탈 시간
stayDuration?: number; // 체류 시간(ms)
url: string; // 페이지 URL
}
FAQs
관리자 시스템을 위한 API SDK 패키지입니다.
We found that @lumir-company/admin-server-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.