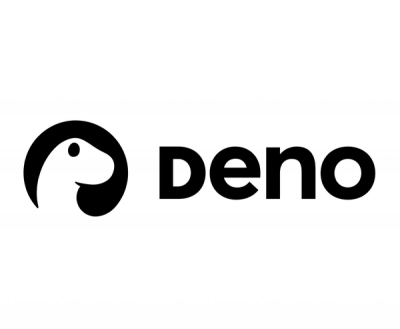
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@marcopeg/utils
Advanced tools
Javascript utils for server and client projects
const cancelEvent = require('@marcopeg/utils/lib/cancel-event)
// use it as simple function with an event as argument
const onClick = (evt) => {
cancelEvent(evt)
...
my handler code
}
// @TODO: use it as middleware
const onClick = cancelEvent((evt) => {
... my handler
})
const config = require('@marcopeg/utils/lib/config)
// will trigger an error if the env variable is not defined
const serverPort = config.get('SERVER_PORT')
// will return a default vailue if the env variable is not defined
const appName = config.get('APP_NAME', 'Default App Name')
// (process.env.NODE_ENV === 'development')
if (config.isDev()) {
...
}
Improve errors stack trace
const pause = require('@marcopeg/utils/lib/pause)
async function foo () {
console.log('before')
await pause(2000) // stop process for 2s
console.log('after')
}
const truncate = require('@marcopeg/utils/lib/truncate)
truncate('123456789', 5, '...')
-> 1...9
FAQs
Javascript utils for server and client projects
The npm package @marcopeg/utils receives a total of 480 weekly downloads. As such, @marcopeg/utils popularity was classified as not popular.
We found that @marcopeg/utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.