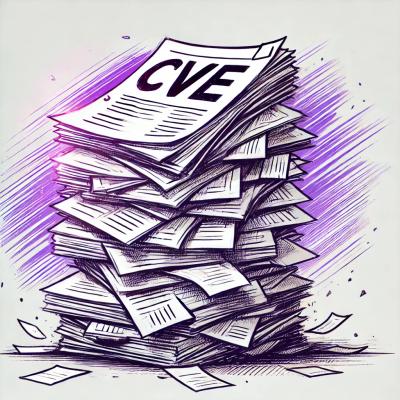
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@material/checkbox
Advanced tools
@material/checkbox is a Material Design implementation of a checkbox component. It provides a customizable and accessible checkbox that can be used in web applications to allow users to make binary choices.
Basic Checkbox
This code demonstrates how to initialize a basic Material Design checkbox using the @material/checkbox package.
import {MDCCheckbox} from '@material/checkbox';
const checkbox = new MDCCheckbox(document.querySelector('.mdc-checkbox'));
Indeterminate State
This code shows how to set a checkbox to an indeterminate state, which is useful for indicating a mixed selection.
import {MDCCheckbox} from '@material/checkbox';
const checkbox = new MDCCheckbox(document.querySelector('.mdc-checkbox'));
checkbox.indeterminate = true;
Handling Change Events
This code demonstrates how to listen for change events on the checkbox and handle them accordingly.
import {MDCCheckbox} from '@material/checkbox';
const checkbox = new MDCCheckbox(document.querySelector('.mdc-checkbox'));
checkbox.listen('change', () => {
console.log('Checkbox state changed to: ', checkbox.checked);
});
react-checkbox-group is a React component for managing a group of checkboxes. It provides a simple API for handling multiple checkboxes and their states. Unlike @material/checkbox, which is a standalone Material Design component, react-checkbox-group is specifically designed for React applications and focuses on managing groups of checkboxes.
rc-checkbox is a React component for creating customizable checkboxes. It offers more flexibility in terms of styling and behavior compared to @material/checkbox. While @material/checkbox follows Material Design guidelines, rc-checkbox allows for more customization options.
react-toggle is a React component for creating toggle switches, which can be used as an alternative to checkboxes. It provides a different UI/UX compared to @material/checkbox but serves a similar purpose of allowing users to make binary choices. It is more suitable for applications that prefer toggle switches over traditional checkboxes.
Selection controls allow the user to select options.
Use checkboxes to:
Contents
Checkboxes allow the user to select one or more items from a set. Checkboxes can be used to turn an option on or off.
npm install @material/checkbox
@use "@material/checkbox";
@use "@material/form-field";
@include checkbox.core-styles;
@include form-field.core-styles;
Note: The form field styles are only required when the checkbox is used with the form field.
The checkbox will work without JavaScript, but you can enhance it with a ripple interaction effect by instantiating MDCCheckbox
on the mdc-checkbox
element. To activate the ripple effect upon interacting with the label, you must also instantiate MDCFormField
on the mdc-form-field
element and set the MDCCheckbox
instance as its input
.
import {MDCFormField} from '@material/form-field';
import {MDCCheckbox} from '@material/checkbox';
const checkbox = new MDCCheckbox(document.querySelector('.mdc-checkbox'));
const formField = new MDCFormField(document.querySelector('.mdc-form-field'));
formField.input = checkbox;
See Importing the JS component for more information on how to import JavaScript.
Material Design spec advises that touch targets should be at least 48px x 48px.
To meet this requirement, add the mdc-checkbox--touch
class to your checkbox as follows:
<div class="mdc-touch-target-wrapper">
<div class="mdc-checkbox mdc-checkbox--touch">
<input type="checkbox"
class="mdc-checkbox__native-control"
id="checkbox-1"/>
<div class="mdc-checkbox__background">
<svg class="mdc-checkbox__checkmark"
viewBox="0 0 24 24">
<path class="mdc-checkbox__checkmark-path"
fill="none"
d="M1.73,12.91 8.1,19.28 22.79,4.59"/>
</svg>
<div class="mdc-checkbox__mixedmark"></div>
</div>
<div class="mdc-checkbox__ripple"></div>
<div class="mdc-checkbox__focus-ring"></div>
</div>
</div>
Note that the outer mdc-touch-target-wrapper
element is only necessary if you want to avoid potentially overlapping touch targets on adjacent elements (due to collapsing margins).
The mdc-checkbox__focus-ring
element ensures that a focus indicator is displayed in high contrast mode around the active/focused checkbox.
We recommend using MDC Checkbox with MDC Form Field for enhancements such as label alignment, label activation of the ripple interaction effect, and RTL-awareness.
<div class="mdc-form-field">
<div class="mdc-checkbox">
<input type="checkbox"
class="mdc-checkbox__native-control"
id="checkbox-1"/>
<div class="mdc-checkbox__background">
<svg class="mdc-checkbox__checkmark"
viewBox="0 0 24 24">
<path class="mdc-checkbox__checkmark-path"
fill="none"
d="M1.73,12.91 8.1,19.28 22.79,4.59"/>
</svg>
<div class="mdc-checkbox__mixedmark"></div>
</div>
<div class="mdc-checkbox__ripple"></div>
<div class="mdc-checkbox__focus-ring"></div>
</div>
<label for="checkbox-1">Checkbox 1</label>
</div>
Note: If you are using IE, you need to include a closing </path>
tag if you wish to avoid console warnings.
Checkboxes can be selected, unselected, or indeterminate. Checkboxes have enabled, disabled, hover, focused, and pressed states.
Note that mdc-checkbox--disabled
is necessary on the root element of CSS-only checkboxes to prevent hover states from activating. Checkboxes that use the JavaScript component do not need this class; a disabled
attribute on the <input>
element is sufficient.
<div class="mdc-checkbox mdc-checkbox--disabled">
<input type="checkbox"
id="basic-disabled-checkbox"
class="mdc-checkbox__native-control"
disabled />
<div class="mdc-checkbox__background">
<svg class="mdc-checkbox__checkmark"
viewBox="0 0 24 24">
<path class="mdc-checkbox__checkmark-path"
fill="none"
d="M1.73,12.91 8.1,19.28 22.79,4.59"/>
</svg>
<div class="mdc-checkbox__mixedmark"></div>
</div>
<div class="mdc-checkbox__ripple"></div>
<div class="mdc-checkbox__focus-ring"></div>
</div>
<label for="basic-disabled-checkbox" id="basic-disabled-checkbox-label">This is my disabled checkbox</label>
Note that data-indeterminate="true"
is necessary on the input element for initial render, or in a CSS-only mode. Checkboxes that use the Javascript component can modify the indeterminate
property at runtime.
<div class="mdc-checkbox">
<input type="checkbox"
id="basic-indeterminate-checkbox"
class="mdc-checkbox__native-control"
data-indeterminate="true"/>
<div class="mdc-checkbox__background">
<svg class="mdc-checkbox__checkmark"
viewBox="0 0 24 24">
<path class="mdc-checkbox__checkmark-path"
fill="none"
d="M1.73,12.91 8.1,19.28 22.79,4.59"/>
</svg>
<div class="mdc-checkbox__mixedmark"></div>
</div>
<div class="mdc-checkbox__ripple"></div>
<div class="mdc-checkbox__focus-ring"></div>
</div>
<label for="basic-indeterminate-checkbox" id="basic-indeterminate-checkbox-label">This is my indeterminate checkbox</label>
MDC Checkbox uses MDC Theme's secondary
color by default for "marked" states (i.e., checked or indeterminate).
Mixin | Description |
---|---|
container-colors($unmarked-stroke-color, $unmarked-fill-color, $marked-stroke-color, $marked-fill-color, $generate-keyframes) | Sets stroke & fill colors for both marked and unmarked state of enabled checkbox. Set $generate-keyframes to false to prevent the mixin from generating @keyframes. |
disabled-container-colors($unmarked-stroke-color, $unmarked-fill-color, $marked-stroke-color, $marked-fill-color) | Sets stroke & fill colors for both marked and unmarked state of disabled checkbox. |
ink-color($color) | Sets the ink color of the checked and indeterminate icons for an enabled checkbox |
disabled-ink-color($color) | Sets the ink color of the checked and indeterminate icons for a disabled checkbox |
focus-indicator-color($color) | Sets the color of the focus indicator (ripple) when checkbox is selected or is in indeterminate state. |
ripple-size($ripple-size) | Sets the ripple size of the checkbox. |
density($density-scale) | Sets density scale for checkbox, Supported density scales are -3 , -2 , -1 , and 0 (default). |
The ripple effect for the Checkbox component is styled using MDC Ripple mixins.
MDCCheckbox
properties and methodsProperty Name | Type | Description |
---|---|---|
checked | boolean | Setter/getter for the checkbox's checked state |
indeterminate | boolean | Setter/getter for the checkbox's indeterminate state |
disabled | boolean | Setter/getter for the checkbox's disabled state |
value | string | Setter/getter for the checkbox's |
If you are using a JavaScript framework, such as React or Angular, you can create a Checkbox for your framework. Depending on your needs, you can use the Simple Approach: Wrapping MDC Web Vanilla Components, or the Advanced Approach: Using Foundations and Adapters. Please follow the instructions here.
MDCCheckboxAdapter
Method Signature | Description |
---|---|
addClass(className: string) => void | Adds a class to the root element. |
removeClass(className: string) => void | Removes a class from the root element. |
forceLayout() => void | Force-trigger a layout on the root element. This is needed to restart animations correctly. If you find that you do not need to do this, you can simply make it a no-op. |
isAttachedToDOM() => boolean | Returns true if the component is currently attached to the DOM, false otherwise. |
isIndeterminate() => boolean | Returns true if the component is in the indeterminate state. |
isChecked() => boolean | Returns true if the component is checked. |
hasNativeControl() => boolean | Returns true if the input is present in the component. |
setNativeControlDisabled(disabled: boolean) => void | Sets the input to disabled. |
setNativeControlAttr(attr: string, value: string) => void | Sets an HTML attribute to the given value on the native input element. |
removeNativeControlAttr(attr: string) => void | Removes an attribute from the native input element. |
MDCCheckboxFoundation
Method Signature | Description |
---|---|
setDisabled(disabled: boolean) => void | Updates the disabled property on the underlying input. Does nothing when the underlying input is not present. |
handleAnimationEnd() => void | animationend event handler that should be applied to the root element. |
handleChange() => void | change event handler that should be applied to the checkbox element. |
14.0.0 (2022-04-27)
unset
is unsupported in IE. (f460e23)validateTooltipWithCaretDistances
method. (3e30054)theme-styles
mixin... the value being retreived from the $theme
map and css property name was swapped. The mixin would request font-size
/font-weight
/letter-spacing
from the $theme
map (which expects size
/weight
/tracking
)... so these values would always be null
. (32b3913)attachTo
. (05db65e)showTimeout
is not set (indicating that this tooltip is about to be re-shown). (6ca8b8f)minRange
param to range sliders to request a minimum gap between the two thumbs. (8fffcb5)valueToAriaValueTextFn
and valueToValueIndicatorTextFn
functions are called for. (b6510c8)PiperOrigin-RevId: 444830518
PiperOrigin-RevId: 419837612
FAQs
The Material Components for the web checkbox component
The npm package @material/checkbox receives a total of 575,828 weekly downloads. As such, @material/checkbox popularity was classified as popular.
We found that @material/checkbox demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.