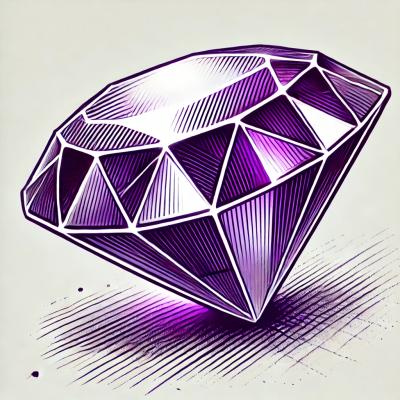
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@material/slider
Advanced tools
@material/slider is a Material Design slider component for the web. It provides a range of features for creating sliders that are consistent with the Material Design guidelines. This package allows developers to create sliders with various configurations, including discrete and continuous sliders, and provides customization options for styling and behavior.
Basic Slider
This code sample demonstrates how to create a basic slider using the @material/slider package. The slider is initialized and an event listener is added to log the value whenever it changes.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://unpkg.com/@material/slider/dist/mdc.slider.css">
<script src="https://unpkg.com/@material/slider/dist/mdc.slider.js"></script>
</head>
<body>
<div class="mdc-slider" tabindex="0" role="slider" aria-valuemin="0" aria-valuemax="100" aria-valuenow="50" aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
<script>
const slider = new mdc.slider.MDCSlider(document.querySelector('.mdc-slider'));
slider.listen('MDCSlider:change', () => console.log(`Value changed to ${slider.value}`));
</script>
</body>
</html>
Discrete Slider with Tick Marks
This code sample demonstrates how to create a discrete slider with tick marks using the @material/slider package. The slider is configured to display discrete values and markers along the track.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://unpkg.com/@material/slider/dist/mdc.slider.css">
<script src="https://unpkg.com/@material/slider/dist/mdc.slider.js"></script>
</head>
<body>
<div class="mdc-slider mdc-slider--discrete mdc-slider--display-markers" tabindex="0" role="slider" aria-valuemin="0" aria-valuemax="100" aria-valuenow="50" aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
<div class="mdc-slider__track-marker-container"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
<script>
const slider = new mdc.slider.MDCSlider(document.querySelector('.mdc-slider'));
slider.listen('MDCSlider:change', () => console.log(`Value changed to ${slider.value}`));
</script>
</body>
</html>
Range Slider
This code sample demonstrates how to create a range slider using the @material/slider package. The range slider allows users to select a range of values within a specified minimum and maximum.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://unpkg.com/@material/slider/dist/mdc.slider.css">
<script src="https://unpkg.com/@material/slider/dist/mdc.slider.js"></script>
</head>
<body>
<div class="mdc-slider mdc-slider--range" tabindex="0" role="slider" aria-valuemin="0" aria-valuemax="100" aria-valuenow="50" aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
<script>
const slider = new mdc.slider.MDCSlider(document.querySelector('.mdc-slider'));
slider.listen('MDCSlider:change', () => console.log(`Value changed to ${slider.value}`));
</script>
</body>
</html>
rc-slider is a React component for creating sliders. It offers a wide range of customization options and supports both single and range sliders. Compared to @material/slider, rc-slider is more focused on React applications and provides more flexibility in terms of customization and styling.
nouislider is a lightweight JavaScript range slider with full touch support. It is highly customizable and supports a wide range of features, including tooltips, pips, and more. Compared to @material/slider, nouislider is more versatile and can be used in various frameworks and environments, not just Material Design.
react-slider is a small, accessible slider component for React. It is designed to be simple and easy to use, with a focus on accessibility. Compared to @material/slider, react-slider is more lightweight and specifically tailored for React applications, making it a good choice for projects that prioritize accessibility and simplicity.
MDC Slider provides an implementation of the Material Design slider component. It is modeled after
the browser's <input type="range">
element. Sliders are fully RTL-aware, and conform to the
WAI-ARIA slider authoring practices.
Note that vertical sliders and range (multi-thumb) sliders are not supported, due to their absence from the material design spec.
Also note that we have taken certain deviations from the UX within the spec, e.g. nuances as to the slider's motion across the track, as well as the style of the slider thumb when in the "off" state in dark mode. Thus, there may be some treatments which deviate from the mocks. These deviations arose out of design feedback from seeing sliders used on the web, and thus have been endorsed by the Material Design team.
npm i --save @material/slider
<div class="mdc-slider" tabindex="0" role="slider"
aria-valuemin="0" aria-valuemax="100" aria-valuenow="0"
aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
</div>
<div class="mdc-slider__thumb-container">
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
<div class="mdc-slider mdc-slider--discrete" tabindex="0" role="slider"
aria-valuemin="0" aria-valuemax="100" aria-valuenow="0"
aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
</div>
<div class="mdc-slider__thumb-container">
<div class="mdc-slider__pin">
<span class="mdc-slider__pin-value-marker"></span>
</div>
<svg class="mdc-slider__thumb" width="21" height="21">
<circle cx="10.5" cy="10.5" r="7.875"></circle>
</svg>
<div class="mdc-slider__focus-ring"></div>
</div>
</div>
Then in JS
import {MDCSlider} from '@material/slider';
const slider = new MDCSlider(document.querySelector('.mdc-slider'));
slider.listen('MDCSlider:change', () => console.log(`Value changed to ${slider.value}`));
You can also include MDCSlider via its UMD version located at dist/mdc.slider[.min].js
// CommonJS
const {MDCSlider} = require('@material/slider/dist/mdc.slider');
// AMD
require(['/path/to/@material/slider/dist/mdc.slider'], ({MDCSlider}) => {
// Use MDCSlider
});
// Global
const {MDCSlider} = mdc.slider;
When MDCSlider
is initialized, it reads the element's aria-valuemin
, aria-valuemax
, and
aria-valuenow
values if present and uses them to set the component's min
, max
, and value
properties. This means you can use these attributes to set these values for the slider within the
DOM.
<div class="mdc-slider" tabindex="0" role="slider"
aria-valuemin="-5" aria-valuemax="50" aria-valuenow="10"
aria-label="Select Value">
<!-- ... -->
</div>
NOTE: If a slider contains a step value it does not mean that the slider is a "discrete" slider. "Discrete slider" is a UX treatment, while having a step value is behavioral.
MDCSlider
supports quantization by allowing users to supply a floating-point step
value via a
data-step
attribute.
<div class="mdc-slider" tabindex="0" role="slider"
aria-valuemin="0" aria-valuemax="100" aria-valuenow="0"
data-step="2" aria-label="Select Value">
<!-- ... -->
</div>
When a step value is given, the slider will quantize all values to match that step value, except for the minimum and maximum values, which can always be set. This is to ensure consistent behavior.
The step value can be any positive floating-point number, or 0
. When the step value is 0
, the
slider is considered to not have any step. A error will be thrown if you are trying to set step
value to be a negative number.
Discrete sliders are required to have a positive step value other than 0. If a step value of 0 is provided, or no value is provided, the step value will default to 1.
Discrete sliders support display markers on their tracks by adding the mdc-slider--display-markers
modifier class to mdc-slider
, and <div class="mdc-slider__track-marker-container"></div>
to the
track container.
<div class="mdc-slider mdc-slider--discrete mdc-slider--display-markers" tabindex="0" role="slider"
aria-valuemin="0" aria-valuemax="100" aria-valuenow="0"
data-step="2" aria-label="Select Value">
<div class="mdc-slider__track-container">
<div class="mdc-slider__track"></div>
<div class="mdc-slider__track-marker-container"></div>
</div>
<!-- ... -->
</div>
NOTE: When the provided step is indivisble to distance between max and min, we place the secondary to last marker proportionally at where thumb could reach and place the last marker at max value.
Adding an aria-disabled
attribute to a slider will initially disable it.
<div class="mdc-slider" tabindex="0" role="slider"
aria-valuemin="0" aria-valuemax="100" aria-valuenow="0"
aria-label="Select Value" aria-disabled="true">
<!-- ... -->
</div>
The MDCSlider
API is modeled after the <input type="range">
element and supports a subset of the
properties that element supports. It also emits events equivalent to a range input's input
and
change
events.
Property Name | Type | Description |
---|---|---|
value | number | The current value of the slider. Changing this will update the slider's value. |
min | number | The minimum value a slider can have. Values set programmatically will be clamped to this minimum value. Changing this property will update the slider's value if it is lower than the new minimum |
max | number | The maximum value a slider can have. Values set programmatically will be clamped to this maximum value. Changing this property will update the slider's value if it is greater than the new maximum |
step | number | Specifies the increments at which a slider value can be set. Can be any positive number, or 0 for no step. Changing this property will update the slider's value to be quantized along the new step increments |
disabled | boolean | Whether or not the slider is disabled |
Method Signature | Description |
---|---|
layout() => void | Recomputes the dimensions and re-lays out the component. This should be called if the dimensions of the slider itself or any of its parent elements change programmatically (it is called automatically on resize). |
stepUp(amount = 1) => void | Increases the slider value by the given amount , or 1 if no amount is given |
stepDown(amount = 1) => void | Decrease the slider value by the given amount , or 1 if no amount is given |
MDCSlider
emits a MDCSlider:input
custom event from its root element whenever the slider value
is changed by way of a user event, e.g. when a user is dragging the slider or changing the value
using the arrow keys. The detail
property of the event is set to the slider instance that was
affected.
MDCSlider
emits a MDCSlider:change
custom event from its root element whenever the slider value
is changed and committed by way of a user event, e.g. when a user stops dragging the slider or
changes the value using the arrow keys. The detail
property of the event is set to the slider
instance that was affected.
The @material/slider
package ships with an MDCSliderFoundation
class that framework authors can
use to build a custom MDCSlider component for their framework.
Method Signature | Description |
---|---|
hasClass(className: string) => boolean | Checks if className exists on the root element |
addClass(className: string) => void | Adds a class className to the root element |
removeClass(className: string) => void | Removes a class className from the root element |
getAttribute(name: string) => string? | Returns the value of the attribute name on the root element, or null if that attribute is not present on the root element. |
setAttribute(name: string, value: string) => void | Sets an attribute name to the value value on the root element. |
removeAttribute(name: string) => void | Removes an attribute name from the root element |
computeBoundingRect() => ClientRect | Computes and returns the bounding client rect for the root element. Our implementations calls getBoundingClientRect() for this. |
getTabIndex() => number | Returns the value of the tabIndex property on the root element |
registerInteractionHandler(type: string, handler: EventListener) => void | Adds an event listener handler for event type type to the slider's root element |
deregisterInteractionHandler(type: string, handler: EventListener) => void | Removes an event listener handler for event type type from the slider's root element |
registerThumbContainerInteractionHandler(type: string, handler: EventListener) => void | Adds an event listener handler for event type type to the slider's thumb container element |
deregisterThumbContainerInteractionHandler(type: string, handler: EventListener) => void | Removes an event listener handler for event type type from the slider's thumb container element |
registerBodyInteractionHandler(type: string, handler: EventListener) => void | Adds an event listener handler for event type type to the <body> element of the slider's document |
deregisterBodyInteractionHandler(type: string, handler: EventListener) => void | Removes an event listener handler for event type type from the <body> element of the slider's document |
registerResizeHandler(handler: EventListener) => void | Adds an event listener handler that is called when the component's viewport resizes, e.g. window.onresize . |
deregisterResizeHandler(handler: EventListener) => void | Removes an event listener handler that was attached via registerResizeHandler . |
notifyInput() => void | Broadcasts an "input" event notifying clients that the slider's value is currently being changed. The implementation should choose to pass along any relevant information pertaining to this event. In our case we pass along the instance of the component for which the event is triggered for. |
notifyChange() => void | Broadcasts a "change" event notifying clients that a change to the slider's value has been committed by the user. Similar guidance applies here as for notifyInput() . |
setThumbContainerStyleProperty(propertyName: string, value: string) => void | Sets a dash-cased style property propertyName to the given value on the thumb container element. |
setTrackStyleProperty(propertyName: string, value: string) => void | Sets a dash-cased style property propertyName to the given value on the track element. |
setMarkerValue(value: number) => void | Sets pin value marker's value when discrete slider thumb moves. |
appendTrackMarkers(numMarkers: number) => void | Appends track marker element to track container. |
removeTrackMarkers() => void | Removes existing marker elements to track container. |
setLastTrackMarkersStyleProperty(propertyName: string, value: string) => void | Sets a dash-cased style property propertyName to the given value on the last element of the track markers. |
isRTL() => boolean | True if the slider is within an RTL context, false otherwise. |
Method Signature | Description |
---|---|
layout() => void | Same as layout() detailed within the component methods table. Does the majority of the work; the component's layout method simply proxies to this. |
getValue() => number | Returns the current value of the slider |
setValue(value: number) => void | Sets the current value of the slider |
getMax() => number | Returns the max value the slider can have |
setMax(max: number) => void | Sets the max value the slider can have |
getMin() => number | Returns the min value the slider can have |
setMin(min: number) => number | Sets the min value the slider can have |
getStep() => number | Returns the step value of the slider |
setStep(step: number) => void | Sets the step value of the slider |
isDisabled() => boolean | Returns whether or not the slider is disabled |
setDisabled(disabled: boolean) => void | Disables the slider when given true, enables it otherwise. |
setupTrackMarker() => void | Put correct number of markers in track for discrete slider that display track markers. No-op if it doesn't meet those criteria. |
All thematic elements of sliders make use of the primary theme color, including when the component is used within a dark mode context.
One tricky issue with sliders is how the thumb is supposed to look when in the disabled or "off" states. In these cases, certain portions of the slider's thumb and track are supposed to become "transparent" and reveal the background color behind it. However, this presents a problem as there is no elegant way to derive what the background color behind the slider should be. We could theoretically walk up the DOM until we found an ancestor with a set background, but that would break the component's encapsulation model.
To solve this, you can supply a css custom property --mdc-slider-bg-color-behind-component
. When
used, this will override the default colors used for the disabled/off state slider thumb and use
the color specified:
.container {
background: #fafafa;
}
.container > .mdc-slider {
--mdc-slider-bg-color-behind-component: #fafafa;
}
If you need to accomplish this in a browser which does not support custom properties (IE11 and
older versions of Edge), you can override the Sass variables $mdc-slider-default-assumed-bg-color
.
$mdc-slider-default-assumed-bg-color: #fafafa;
@import "@material/slider/mdc-slider";
If you're using the .mdc-theme--dark
classes, you'll want to override $mdc-slider-dark-theme-assumed-bg-color
instead.
Finally, if you'd prefer not to use Sass, you can use the following CSS snippet which should be
included after the @material/slider
styles have been loaded onto the page:
.mdc-slider--off .mdc-slider__thumb {
fill: YOUR_CUSTOM_COLOR;
}
.mdc-slider--disabled .mdc-slider__thumb {
stroke: YOUR_CUSTOM_COLOR !important;
}
Note that the following snippet assumes you are not using mdc-theme--dark
. If you are, you may
need to add .mdc-theme--dark
as an additional ancestor selector before the .mdc-slider--*
classes.
Because MDCSlider
updates its UI based on the values it reads in when it is instantiated, there is
potential for an incorrect first render before the script containing the MDCSlider
initialization
logic executes. To avoid this, there are a few things you can attempt to do:
If the slider's aria-valuenow
is set to "0"
, you can manually add the class mdc-slider--off
to the root mdc-slider
element.
If you know how wide the slider will be at the time of instantiation, you can add an inline style
to the mdc-slider__thumb-container
/mdc-slider__track
elements which will position it correctly
by using similar logic to that within our code:
(value - min) / (max - min)
. We'll call this pctComplete
.pctComplete
. We'll call this translatePx
. Note that if you're using the slider in an RTL
content, modify translatePx
such that translatePx = <width of the slider element> - translatePx
.transform
style on mdc-slider__thumb-container
to translateX(${translatePx}px) translateX(-50%)
.transform
style on mdc-slider__track
to scale(pctComplete)
.FAQs
The Material Components for the web slider component
The npm package @material/slider receives a total of 447,296 weekly downloads. As such, @material/slider popularity was classified as popular.
We found that @material/slider demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.