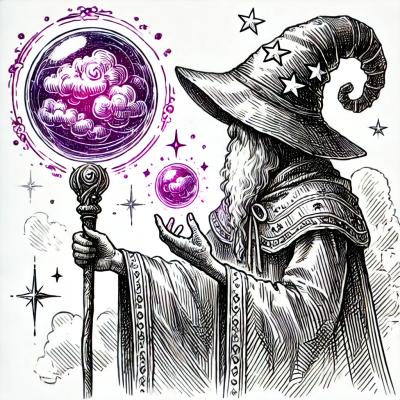
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@material/snackbar
Advanced tools
The MDC Snackbar component is a spec-aligned snackbar/toast component adhering to the Material Design snackbars & toasts requirements. It requires JavaScript to show and hide itself.
npm install --save @material/snackbar
<div class="mdc-snackbar"
aria-live="assertive"
aria-atomic="true"
aria-hidden="true">
<div class="mdc-snackbar__text"></div>
<div class="mdc-snackbar__action-wrapper">
<button type="button" class="mdc-button mdc-snackbar__action-button"></button>
</div>
</div>
MDC Snackbar can be start aligned (including in RTL contexts). To create a start-aligned
snackbar, add the mdc-snackbar--align-start
modifier class to the root element.
<div class="mdc-snackbar mdc-snackbar--align-start"
aria-live="assertive"
aria-atomic="true"
aria-hidden="true">
<div class="mdc-snackbar__text"></div>
<div class="mdc-snackbar__action-wrapper">
<button type="button" class="mdc-button mdc-snackbar__action-button"></button>
</div>
</div>
MDC Snackbar ships with a Component / Foundation combo which provides the API for showing snackbar messages with optional action.
import {MDCSnackbar, MDCSnackbarFoundation} from '@material/snackbar';
const mdcSnackbar = require('mdc-snackbar');
const MDCSnackbar = mdcSnackbar.MDCSnackbar;
const MDCSnackbarFoundation = mdcSnackbar.MDCSnackbarFoundation;
require(['path/to/mdc-snackbar'], mdcSnackbar => {
const MDCSnackbar = mdcSnackbar.MDCSnackbar;
const MDCSnackbarFoundation = mdcSnackbar.MDCSnackbarFoundation;
});
const MDCSnackbar = mdc.snackbar.MDCSnackbar;
const MDCSnackbarFoundation = mdc.snackbar.MDCSnackbarFoundation;
If you do not care about retaining the component instance for the snackbar, simply call attachTo()
and pass it a DOM element.
mdc.snackbar.MDCSnackbar.attachTo(document.querySelector('.mdc-snackbar'));
Snackbars can easily be initialized using their default constructors as well, similar to attachTo
.
import {MDCSnackbar} from '@material/snackbar';
const snackbar = new MDCSnackbar(document.querySelector('.mdc-snackbar'));
Once you have obtained an MDCSnackbar instance attached to the DOM, you can use
the show
method to trigger the display of a message with optional action. The
show
method takes an object for snackbar data. The table below shows the
properties and their usage.
Property | Effect | Remarks | Type |
---|---|---|---|
message | The text message to display. | Required | String |
timeout | The amount of time in milliseconds to show the snackbar. | Optional (default 2750) | Integer |
actionHandler | The function to execute when the action is clicked. | Optional | Function |
actionText | The text to display for the action button. | Required if actionHandler is set | String |
multiline | Whether to show the snackbar with space for multiple lines of text | Optional | Boolean |
actionOnBottom | Whether to show the action below the multiple lines of text | Optional, applies when multiline is true | Boolean |
To respond to a snackbar action, assign a function to the optional actionHandler
property in the object that gets passed to the show
method. If you choose to set this property, you must also set the actionText
property.
<div class="mdc-snackbar"
aria-live="assertive"
aria-atomic="true"
aria-hidden="true">
<div class="mdc-snackbar__text"></div>
<div class="mdc-snackbar__action-wrapper">
<button type="button" class="mdc-button mdc-snackbar__action-button"></button>
</div>
</div>
import {MDCSnackbar} from '@material/snackbar';
const snackbar = new MDCSnackbar(document.querySelector('.mdc-snackbar'));
const dataObj = {
message: messageInput.value,
actionText: 'Undo',
actionHandler: function () {
console.log('my cool function');
}
};
snackbar.show(dataObj);
By default the snackbar will be dimissed when the user presses the action button.
If you want the snackbar to remain visible until the timeout is reached (regardless of
whether the user pressed the action button or not) you can set the dismissesOnAction
property to false
:
const snackbar = new MDCSnackbar(document.querySelector('.mdc-snackbar'));
snackbar.dismissesOnAction = false
MDC Snackbar ships with an MDCSnackbarFoundation
class that external frameworks and libraries can
use to integrate the component. As with all foundation classes, an adapter object must be provided.
The adapter for snackbars must provide the following functions, with correct signatures:
Method Signature | Description |
---|---|
addClass(className: string) => void | Adds a class to the root element. |
removeClass(className: string) => void | Removes a class from the root element. |
setAriaHidden() => void | Sets aria-hidden="true" on the root element. |
unsetAriaHidden() => void | Removes the aria-hidden attribute from the root element. |
setActionAriaHidden() => void | Sets aria-hidden="true" on the action element. |
unsetActionAriaHidden() => void | Removes the aria-hidden attribute from the action element. |
setActionText(actionText: string) => void | Set the text content of the action element. |
setMessageText(message: string) => void | Set the text content of the message element. |
setFocus() => void | Sets focus on the action button. |
visibilityIsHidden() => boolean | Returns document.hidden property. |
registerBlurHandler(handler: EventListener) => void | Registers an event handler to be called when a blur event is triggered on the action button |
deregisterBlurHandler(handler: EventListener) => void | Deregisters a blur event handler from the actionButton |
registerVisibilityChangeHandler(handler: EventListener) => void | Registers an event handler to be called when a 'visibilitychange' event occurs |
deregisterVisibilityChangeHandler(handler: EventListener) => void | Deregisters an event handler to be called when a 'visibilitychange' event occurs |
registerCapturedInteractionHandler(evtType: string, handler: EventListener) => void | Registers an event handler to be called when the given event type is triggered on the body |
deregisterCapturedInteractionHandler(evtType: string, handler: EventListener) => void | Deregisters an event handler from the body |
registerActionClickHandler(handler: EventListener) => void | Registers an event handler to be called when a click event is triggered on the action element. |
deregisterActionClickHandler(handler: EventListener) => void | Deregisters an event handler from a click event on the action element. This will only be called with handlers that have previously been passed to registerActionClickHandler calls. |
registerTransitionEndHandler(handler: EventListener) => void | Registers an event handler to be called when an transitionend event is triggered on the root element. Note that you must account for vendor prefixes in order for this to work correctly. |
deregisterTransitionEndHandler(handler: EventListener) => void | Deregisters an event handler from an transitionend event listener. This will only be called with handlers that have previously been passed to registerTransitionEndHandler calls. |
If you are loading the mdc-snackbar
CSS asynchronously, you may experience a brief flash-of-unstyled-content (FOUC) due to the
snackbar's translate transition running once the CSS loads. To avoid this temporary FOUC, you can add the following simple style
before the mdc-snackbar
CSS is loaded:
.mdc-snackbar { transform: translateY(100%); }
This will move the snackbar offscreen until the CSS is fully loaded and avoids a translate transition upon load.
FAQs
The Material Components for the web snackbar component
The npm package @material/snackbar receives a total of 813,856 weekly downloads. As such, @material/snackbar popularity was classified as popular.
We found that @material/snackbar demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.