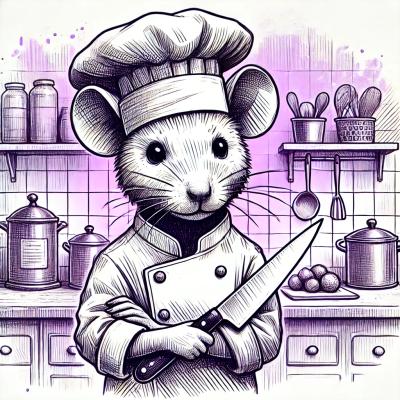
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@medipass/checkout-sdk
Advanced tools
The Medipass Checkout SDK is a client-side TypeScript library which allows you to interact with Medipass from your web interface. It has full TypeScript support.
yarn add @medipass/checkout-sdk
or NPM:
npm install @medipass/checkout-sdk
A payment request URL is passed to the createCheckout function, which opens a secure pop-up window to Medipass to take the payment.
import medipassCheckoutSDK from '@medipass/checkout-sdk';
// or: const medipassCheckoutSDK = require('@medipass/checkout-sdk');
medipassCheckoutSDK.init({
env: 'stg',
onSuccess: ({ transactionId }) => {
// handle success
},
onFailure: ({ transactionId }) => {
// handle failure
},
onCancel: ({ transactionId }) => {
// handle cancel
},
onClose: () => {
// handle close
}
});
const paymentRequestUrl = getPaymentRequestUrl();
await medipassCheckoutSDK.createCheckout({
paymentRequestUrl // the paymentRequestUrl returned after creating a Medipass invoice
});
<script>
tag<html>
<head>
<script src="https://unpkg.com/@medipass/checkout-sdk/dist/umd/index.min.js"></script>
</head>
<body>
<script>
medipassCheckoutSDK.init({
env: 'stg',
onSuccess: ({ transactionId }) => {
// handle success
},
onFailure: ({ transactionId }) => {
// handle failure
},
onCancel: ({ transactionId }) => {
// handle cancel
},
onClose: () => {
// handle close
}
});
const paymentRequestUrl = getPaymentRequestUrl();
medipassCheckoutSDK.createCheckout({
paymentRequestUrl // the paymentRequestUrl returned after creating a Medipass invoice
});
</script>
</body>
</html>
You can update a patient's payment details using the requestUpdatePaymentDetails function.
import medipassCheckoutSDK from '@medipass/checkout-sdk';
// or: const medipassCheckoutSDK = require('@medipass/checkout-sdk');
await medipassCheckoutSDK.requestUpdatePaymentDetails({
apiKey, // apiKey | undefined
token, // token | undefined
patientRefId, // patientRefId
env, // 'stg' | 'prod'
onSuccess, // Invoked when the payment method update has been successful
onFailure, // Invoked when the payment method update has failed
onCancel, // Invoked when the payment method update has been rejected
onClose, // Invoked when the pop-up window has been closed by the user
callbackOrigin // The origin of the window invoking the checkout SDK
});
<script>
tag<html>
<head>
<script src="https://unpkg.com/@medipass/checkout-sdk/dist/umd/index.min.js"></script>
</head>
<body>
<script>
medipassCheckoutSDK.requestUpdatePaymentDetails({
apiKey, // apiKey | undefined
token, // token | undefined
patientRefId, // patientRefId
env, // 'stg' | 'prod'
onSuccess, // Invoked when the payment method update has been successful
onFailure, // Invoked when the payment method update has failed
onCancel, // Invoked when the payment method update has been rejected
onClose, // Invoked when the pop-up window has been closed by the user
callbackOrigin // The origin of the window invoking the checkout SDK
});
</script>
</body>
</html>
Take note of the following:
Object
| required
{
env: 'stg' | 'prod',
onSuccess: function({ transactionId }) {}, // Invoked when the payment has been successful
onFailure: function({ transactionId }) {}, // Invoked when the payment has failed
onCancel: function({ transactionId }) {}, // Invoked when the payment has been rejected
onClose: function() {} // Invoked when the pop-up window has been closed by the user before approving or rejecting the payment
}
Object
| required
{
paymentRequestUrl: string,
}
FAQs
Medipass Checkout SDK
We found that @medipass/checkout-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.