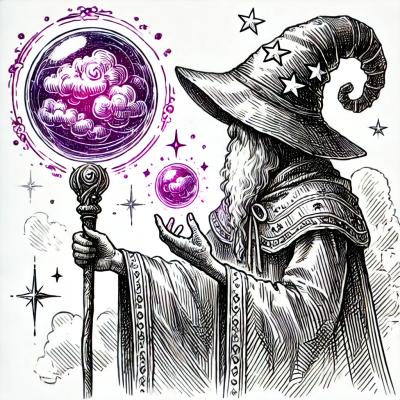
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@medipass/partner-sdk
Advanced tools
The Medipass Partner SDK is a client-side JavaScript library which allows you to interact with Medipass from your web interface.
yarn add @medipass/partner-sdk
or NPM:
npm install @medipass/partner-sdk
import medipassTransactionSDK from '@medipass/partner-sdk';
// or: const medipassTransactionSDK = require('@medipass/partner-sdk');
medipassTransactionSDK.setConfig({
env: 'stg',
apiKey: 'xyz',
appId: 'my-app',
appVersion: '1.0.0'
});
medipassTransactionSDK.renderCreateTransaction(
{},
{
onSuccess: data => {
// handle success
},
onError: data => {
// handle success
},
onCancel: () => {
// handle cancel
}
}
);
<script>
tag<html>
<head>
<script src="https://unpkg.com/@medipass/partner-sdk/umd/@medipass/partner-sdk.min.js"></script>
</head>
<body>
<script>
MedipassTransactionSDK.setConfig({
env: 'stg',
apiKey: 'xyz',
appId: 'my-app',
appVersion: '1.0.0'
});
MedipassTransactionSDK.renderCreateTransaction(
{},
{
onSuccess: data => {
// handle success
},
onError: data => {
// handle success
},
onCancel: () => {
// handle cancel
}
}
);
</script>
</body>
</html>
Object
| required
{
env: 'stg' | 'prod',
apiKey: string,
appId: string,
appVersion: string
}
Object
| required
With the payload
parameter, you are able to pre-populate SDK fields. All attributes in the payload are optional (however, attributes marked with a !
are required) - so you can pre-populate what you wish.
{
/* ====== START: GENERAL PRE-POPULATION ATTRIBUTES ====== */
funder: "medicare" | "hicaps" | "patient-funded" | "ghs",
providerNumber: string,
invoiceReference: string,
memberId: string, // Medipass Member ID
// OR
patient: {
firstName: string,
lastName: string,
mobile: string,
dob: string, // "YYYY-MM-DD" format
accountNumber: string, // Medicare card number / PHI card number
reference: string // Medicare card reference / PHI card rank
},
claimableItems: [{
itemCode!: string,
serviceDateString: string, // "YYYY-MM-DD" format
price: string, // E.g. "$40.00"
quantity: number,
isTaxable: boolean
}],
nonClaimableItems: [{
description!: string,
price: string
}],
webhooks: [{
url!: string,
event!:
'memberApprovedInvoice' |
'memberRejectedInvoice' |
'medipassAutoCancelledInvoice' |
'healthFundApprovedInvoice' |
'healthFundRejectedInvoice',
method!: 'GET' | 'PUT' | 'POST' | 'DELETE',
headers: { [key: string]: string }
}],
/* ====== END: GENERAL PRE-POPULATION ATTRIBUTES ====== */
/* ====== START: FUNDER-SPECIFIC PRE-POPULATION ATTRIBUTES ====== */
funderData: {
hicaps: {
patient: {
healthFundCode: string
},
transactionMethod: 'phone' | 'terminal'
},
medicare: {
isBulkBilled: boolean,
claimantMemberId: string, // Medipass Member ID
// OR
claimant: {
firstName: string,
lastName: string,
mobile: string,
dob: string, // "YYYY-MM-DD" format
accountNumber: string, // Medicare card number / PHI card number
reference: string // Medicare card reference / PHI card rank
},
referral: {
providerNumber: string,
providerName: string,
issueDateString: string,
period: 'standard' | 'non-standard' | 'indefinite'
}
},
ghs: {
isEmergencyTreatment: boolean,
includesHospitalInpatientItems: boolean,
failedToAttendClaim: boolean,
dan: string,
referringJointHealthCentre: string,
referringMedicalOfficer: string,
noDan: boolean,
noDanReason: string,
noEpId: boolean,
noEpIdReason: string
}
}
/* ====== END: FUNDER-SPECIFIC PRE-POPULATION ATTRIBUTES ====== */
}
Object
{
onSuccess: function(transaction) {}, // Invoked when the transaction has submitted successfully.
onError: function(error) {}, // Invoked when the transaction submission failed.
onCancel: function() {}, // Invoked when the 'Cancel' button is clicked.
// Optional config overrides - if not set, will use global config from sdk.setConfig().
env: 'stg' | 'prod',
apiKey: string,
appId: string,
appVersion: string
}
Object
| required
{
// required
transactionId: string,
// Optional override Business ID - by default, it uses business attached to API Key.
businessId: string
}
Object
{
// Optional config overrides - if not set, will use global config from sdk.setConfig().
env: 'stg' | 'prod',
apiKey: string,
appId: string,
appVersion: string
}
Object
| required
Discover a member using query parameters.
The response will be the matching member's internal ID. If a custodians
array field is also present, the
matching member is a dependant. Each custodian object contains the custodian's member ID, first name and
last name. The custodian is important when creating an invoice for a dependant because the claim/payment
authorisation can only be performed by a custodian. A dependant inherits their custodians' email and mobile,
and therefore will be included in such a search.
Email and mobile are the primary search parameters. The order of search parameter priorities are as
follows: email
> mobile
> dobString
> lastName
> firstName
.
The following search sets are recommended:
{ email }
{ mobile, dobString }
{ mobile, firstName, lastName }
{ dobString, firstName, lastName }
The following query sets are not allowed:
{ firstName }
{ lastName }
{ dobString }
{ firstName, dobString }
{ lastName, dobString }
{
query: {
email: string,
mobile: string,
dobString: string, // "YYYY-MM-DD"
firstName: string,
lastName: string
}
}
medipassTransactionSDK.members.discoverMember({ email: 'john@example.com' });
Object
| required
Retrieve a business' provider and their status.
A provider number must be supplied as a query param to filter the results and return a single provider.
{
query: {
providerNumber: string
}
}
Object
| required
Retrieve a patient's payment methods.
A patient id must be supplied as a query parameter.
{
query: {
patientId: string
}
}
Get payment methods by patient id
Object
| required
Retrieve a patient's payment methods by a patient's ref id.
A patient's ref id must be supplied as a query parameter.
{
query: {
refId: string
}
}
Get payment methods by patient ref id
Object
| required
Get a list of claim items. A claim item describes a service that a patient can claim against.
{
query: {
page: number,
limit: number,
searchText: string
itemCode: string,
funderCode: string,
funderId: string,
modalityId: string,
serviceDateString: string,
isBulkBilled: string,
isInHospital: string
},
// Optional override Business ID - by default, it uses business attached to API Key.
businessId: string
}
medipassTransactionSDK.claimItems.getBusinessClaimItems({
query: {
page: 1,
limit: 10,
funderCode: 'medicare',
searchText: 'Awesome Item'
}
});
Object
| required
Get transaction details for a business.
{
// Required
transactionId: string,
// Optional override Business ID - by default, it uses business attached to API Key.
businessId: string
}
medipassTransactionSDK.transactions.getBusinessTransaction({ transactionId: '123' });
Async function that returns a PDF blob.
Object
| required
Downloads the transaction summary in PDF format.
Note: this method is only supported for Medicare transactions and downloads the Medicare statement.
{
// Required
transactionId: string,
// Optional override Business ID - by default, it uses business attached to API Key.
businessId: string
}
Object
| optional
Object
| optional
{
// Optional - when set to true, the PDF will not be automatically downloaded on the client.
suppressLocalDownload: string
}
medipassTransactionSDK.transactions.downloadPDF({ transactionId: '123' });
Object
| required
Fetches the payment report for a given transaction. Response is in JSON format.
Note: this method is only supported for Medicare transactions.
{
// Required
transactionId: string,
query: {
// When true, the payment report is retrieved in real time and the cache is updated if successful.
forceRefresh: boolean
},
// Optional override Business ID - by default, it uses business attached to API Key.
businessId: string
}
{
requestedDate: string,
reportResult: number,
sessionId: number,
paymentRunDateString: string,
paymentRunNumber: string,
amountBenefitPaidString: string,
bankAccount: {
accountNumber: string,
bsb: string,
accountName: string
},
payments: [
{
claimId: string,
claimDateString: string,
amountChargedString: string,
amountBenefitPaidString: string,
amountDepositedString: string
}
]
}
medipassTransactionSDK.transactions.getPaymentReport({ transactionId: '123', query: { forceRefresh: true } });
Object
| required
Fetches the processing report for a given transaction. Response is in JSON format.
Note: this method is only supported for Medicare transactions.
{
// Required
transactionId: string,
query: {
// When true, the processing report is retrieved in real time and the cache is updated if successful.
forceRefresh: boolean
},
// Optional override Business ID - by default, it uses business attached to API Key.
businessId: string
}
{
requestedDate: string,
reportResult: number,
sessionId: number,
amountChargedString: string,
amountBenefitPaidString: string,
providerNumber: string,
claimItems: [
{
serviceDateString: string,
itemCode: string,
claimId: string,
serviceId: string,
amountBenefitString: string,
gatewayCode: string,
gatewayDescription: string,
amountChargedString: string,
healthFundAccount: {
membershipNumber: string,
cardRank: string
},
patient: {
firstName: string,
lastName: string
},
cardFlag: string,
cardFlagDescription: string,
numberOfPatientsSeen: string,
voucherId: string
}
]
}
medipassTransactionSDK.transactions.getProcessingReport({ transactionId: '123', query: { forceRefresh: true } });
Object
| required
Object
| required
Returns a standalone URL.
Examples can be found in the in the examples/
folder.
The Medipass Transaction SDK supports all popular browsers, including Internet Explorer 11 and above. Unfortunately, support for Internet Exporer 10 and below is discontinued.
FAQs
Medipass Partner SDK
We found that @medipass/partner-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 16 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.