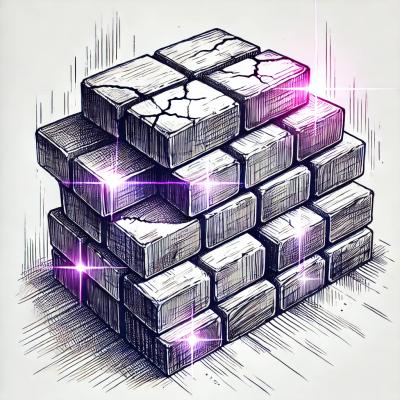
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@microsoft/omnichannel-chat-sdk
Advanced tools
Headless Chat SDK to build your own chat widget against Dynamics 365 Omnichannel Services.
npm install @microsoft/omnichannel-chat-sdk --save
Method | Description | Notes |
---|---|---|
OmnichannelChatSDK.initialize() | Initializes ChatSDK internal data | |
OmnichannelChatSDK.startChat() | Starts OC chat, handles prechat response | |
OmnichannelChatSDK.endChat() | Ends OC chat | |
OmnichannelChatSDK.getPreChatSurvey() | Adaptive card data of PreChat survey | |
OmnichannelChatSDK.getLiveChatConfig() | Get live chat config | |
OmnichannelChatSDK.getCurrentLiveChatContext() | Get current live chat context information to reconnect to the same chat | |
OmnichannelChatSDK.getChatToken() | Get chat token | |
OmnichannelChatSDK.getMessages() | Get all messages | |
OmnichannelChatSDK.sendMessage() | Send message | |
OmnichannelChatSDK.onNewMessage() | Handles system message, client/agent messages, adaptive cards, attachments to download | |
OmnichannelChatSDK.onTypingEvent() | Handles agent typing event | |
OmnichannelChatSDK.onAgentEndSession() | Handler when agent ends session | |
OmnichannelChatSDK.sendTypingEvent() | Sends customer typing event | |
OmnichannelChatSDK.emailLiveChatTranscript() | Email transcript | |
OmnichannelChatSDK.getLiveChatTranscript() | Get transcript data (JSON) | |
OmnichannelChatSDK.uploadFileAttachment() | Send file attachment | |
OmnichannelChatSDK.downloadFileAttachment() | Download file attachment | |
OmnichannelChatSDK.createChatAdapter() | Get IC3Adapter (Web only) |
import OmnichannelChatSDK from '@microsoft/omnichannel-chat-sdk';
const omnichannelConfig = {
orgUrl: "",
orgId: "",
widgetId: ""
};
const chatSDKConfig = { // Optional
DataMasking: {
disable: false,
maskingCharacter: '#'
}
};
const chatSDK = new OmnichannelChatSDK.OmnichannelChatSDK(omnichannelConfig, chatSDKConfig);
await chatSDK.initialize();
const liveChatContext = await chatSDK.getCurrentLiveChatContext();
const chatToken = await chatSDK.getChatToken();
const liveChatConfig = await chatSDK.getLiveChatConfig();
Option 1
const preChatSurvey = await getPreChatSurvey(); // Adaptive Cards JSON payload data
Option 2
const parseToJSON = false;
const preChatSurvey = await getPreChatSurvey(parseToJSON); // Adaptive Cards payload data as string
const optionalParams = {
preChatResponse: '', // PreChatSurvey response
liveChatContext: {} // EXISTING chat context data
};
await chatSDK.startChat(optionalParams);
await chatSDK.endChat();
chatSDK.onNewMessage((message) => {
console.log(`[NewMessage] ${message.content}`); // IC3 protocol message data
console.log(message);
});
chatSDK.onAgentEndSession(() => {
console.log("Session ended!");
});
chatSDK.onTypingEvent(() => {
console.log("Agent is typing...");
})
const messages = await chatSDK.getMessages();
import {DeliveryMode, MessageContentType, MessageType, PersonType} from '@microsoft/omnichannel-chat-sdk';
...
const displayName = "Contoso"
const message = "Sample message from customer";
const messageToSend = {
content: message
};
await chatSDK.sendMessage(messageToSend);
await chatSDK.sendTypingEvent();
const fileInfo = {
name: '',
type: '',
size: '',
data: ''
};
await chatSDK.uploadFileAttachment(fileInfo);
const blobResponse = await chatsdk.downloadFileAttachment(message.fileMetadata);
...
// React Native implementation
const fileReaderInstance = new FileReader();
fileReaderInstance.readAsDataURL(blobResponse);
fileReaderInstance.onload = () => {
const base64data = fileReaderInstance.result;
return <Image source={{uri: base64data}}/>
}
await chatSDK.getLiveChatTranscript();
const body = {
emailAddress: 'contoso@microsoft.com',
attachmentMessage: 'Attachment Message',
locale: 'en-us'
};
await chatSDK.emailLiveChatTranscript(body);
import * as AdaptiveCards, { Action } from "adaptivecards";
...
const preChatSurvey = await chatSDK.getPreChatSurvey();
...
// Web implementation
const renderPreChatSurvey = () => {
const adaptiveCard = new AdaptiveCards.AdaptiveCard();
adaptiveCard.parse(preChatSurvey); // Parses Adaptive Card JSON data
adaptiveCard.onExecuteAction = async (action: Action) => { // Adaptive Card event handler
const preChatResponse = (action as any).data;
const optionalParams: any = {};
if (preChatResponse) {
optionalParams.preChatResponse = preChatResponse;
}
await chatSDK.startChat(optionalParams);
}
const renderedCard = adaptiveCard.render(); // Renders as HTML element
return <div ref={(n) => { // Returns React element
n && n.firstChild && n.removeChild(n.firstChild); // Removes duplicates fix
renderedCard && n && n.appendChild(renderedCard);
}} />
}
await chatSDK.startChat(); // Starts NEW chat
const liveChatContext = await chatSDK.getCurrentLiveChatContext(); // Gets chat context
cache.saveChatContext(liveChatContext); // Custom logic to save chat context to cache
...
// Page/component reloads, ALL previous states are GONE
...
const liveChatContext = cache.loadChatContext() // Custom logic to load chat context from cache
const optionalParams = {};
optionalParams.liveChatContext = liveChatContext;
await chatSDK.startChat(optionalParams); // Reconnects to EXISTING chat
...
const messages = await chatSDK.getMessages(); // Gets all messages from EXISTING chat
messages.reverse().forEach((message: any) => renderMessage(message)); // Logic to render all messages to UI
// add if using against an authenticated chat endpoint
// see https://docs.microsoft.com/en-us/dynamics365/omnichannel/administrator/create-chat-auth-settings on how to set up an authenticated chat widget
const chatSDKConfig = {
getAuthToken: async () => {
const response = await fetch("http://contosohelp.com/token");
if (response.ok) {
return await response.text();
}
else {
return null
}
}
}
const chatSDK = new OmnichannelChatSDK.OmnichannelChatSDK(omnichannelConfig, chatSDKConfig);
await chatSDK.initialize();
// from this point, this acts like a regular chat widget
NOTE: Currently supported on web only
import OmnichannelChatSDK from '@microsoft/omnichannel-chat-sdk';
import ReactWebChat from 'botframework-webchat';
...
const chatSDK = new OmnichannelChatSDK.OmnichannelChatSDK(omnichannelConfig, chatSDKConfig);
await chatSDK.initialize();
const optionalParams = {
preChatResponse: '' // PreChatSurvey response
};
await chatSDK.startChat(optionalParams);
const chatAdapter = await chatSDK.createChatAdapter();
// Subscribes to incoming message (OPTION 1)
chatSDK.onNewMessage((message) => {
console.log(`[NewMessage] ${message.content}`); // IC3 protocol message data
console.log(message);
});
// Subscribes to incoming message (OPTION 2)
(chatAdapter as any).activity$.subscribe((activity: any) => {
if (activity.type === "message") {
console.log("[Message activity]");
console.log(activity); // DirectLine protocol activity data
}
});
...
<ReactWebChat
userID="teamsvisitor"
directLine={chatAdapter}
sendTypingIndicator={true}
/>
Custom Control | WebChat Control | |
---|---|---|
Features | ||
Chat Widget UI | Not provided | Basic chat client provided |
Data Masking | Embedded | Requires Attachment Middleware implementation |
Send Typing indicator | Embedded | Requires sendTypingIndicator flag set to true |
PreChat Survey | Requires Adaptive Cards renderer | Requires Adaptive Cards renderer |
Display Attachments | Requires implementation | Provided & Customizable |
Incoming messages handling | IC3 protocol message data | DirectLine activity data |
Custom Control | Gifted Chat Control | WebChat Control | |
---|---|---|---|
Features | Currently not supported | ||
Chat Widget UI | Not provided | Basic chat client provided | X |
Data Masking | Embedded | Embedded | X |
Send Typing indicator | Embedded | WIP | X |
PreChat Survey | Requires Adaptive Cards renderer | Requires Adaptive Cards renderer | X |
Display Attachments | Requires implementation | Embedded | X |
Incoming messages handling | IC3 protocol message data | IC3 protocol message data | X |
This project welcomes contributions and suggestions. Most contributions require you to agree to a Contributor License Agreement (CLA) declaring that you have the right to, and actually do, grant us the rights to use your contribution. For details, visit https://cla.opensource.microsoft.com.
When you submit a pull request, a CLA bot will automatically determine whether you need to provide a CLA and decorate the PR appropriately (e.g., status check, comment). Simply follow the instructions provided by the bot. You will only need to do this once across all repos using our CLA.
This project has adopted the Microsoft Open Source Code of Conduct. For more information see the Code of Conduct FAQ or contact opencode@microsoft.com with any additional questions or comments.
FAQs
Microsoft Omnichannel Chat SDK
The npm package @microsoft/omnichannel-chat-sdk receives a total of 13,018 weekly downloads. As such, @microsoft/omnichannel-chat-sdk popularity was classified as popular.
We found that @microsoft/omnichannel-chat-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.