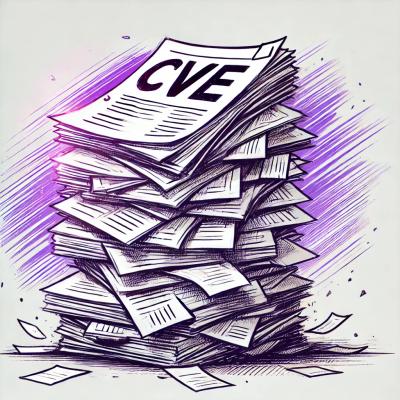
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@modulor-js/html
Advanced tools
Yet another template engine based on tagged template literals
npm install --save @modulor-js/html
modulor-html
provides a way to efficiently (re)render templates to DOM.
It is highly influenced by lit-html
and designed to be compatible with it.
The main exports are:
html
: creates template function (wire)
render
: renders template into DOM container
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const tpl = (myVar) => html`
<span>Hello ${myVar}</span>
`;
render(tpl('world'), $container);
//or alternative way
tpl('world')($container);
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const tpl = (date) => html`
<span>Time: ${date.toLocaleTimeString()}</span>
`;
setInterval(() => {
render(tpl(new Date()), $container);
}, 1000);
Can be used in production and is already battle tested
Designed to be compatible with CustomElements
Small size (3.3kb minigzipped)
Performance is comparable to lit-html
Native js syntax for templates
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const tpl = (names) => html`
<span>Hello:</span>
<ul>
${names.map((name) => html`
<li>${name}</li>
`)}
</ul>
`;
render(tpl(['Steven', 'John']), $container);
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const tpl = (myVar) => html`
<span>Hello ${myVar}</span>
`;
render(tpl(Promise.resolve('world')), $container);
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const tpl = ($el) => html`
<div class="wrapper">${$el}</div>
`;
const $element = document.createElement('span');
$element.classList.add('my-class');
$element.innerText = 'i am element';
render(tpl($element), $container);
Values as functions can be used to get low-level access to rendering process. It most cases you won't need it.
Such functions are called with only one argument container
, which has standart Node
api such as .appendChild()
and so on.
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const tpl = (fn) => html`
<span>counter: ${fn}</span>
`;
const fn = (container) => {
let i = 0;
const $text = document.createTextNode(i);
container.appendChild($text);
setInterval(() => {
$text.textContent = i++;
}, 1000);
}
render(tpl(fn), $container);
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const tpl = ({ checked, dynamicAttr }) => html`
<input type="checkbox" checked="${checked}" "${dynamicAttr}" />
`;
render(tpl({
checked: true,
dynamicAttr: 'disabled'
}), $container);
If target element has a property
of attribute name, then value will be set as that property. This makes possible to pass values other than primitive ones
Values can be promises. In such case attribute value will be set once promise is resolved
If attribute key is a function, it will be called with parameters target, attributeValue
. E.g.:
html`
<input ${(target, value) => {
//target === <input element>
//value === 'test'
}}="test">
`
Following example shows such case:
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
const on = (eventName) => ($el, callback) => {
$el.addEventListener(eventName, callback);
};
const handler = (event) => console.log(event.target);
const tpl = (handler) => html`
<span ${on('click')}=${handler}>click me</span>
`;
render(tpl(handler), $container);
until(promise, placeholderContent)
(demo)Renders placeholderContent
until promise
resolves
modulor-html
works perfect with native custom elements and their existing polyfills.
tested with https://github.com/webcomponents/webcomponentsjs and https://github.com/WebReflection/document-register-element
import { html, render } from '@modulor-js/html';
const $container = document.querySelector('#container');
customElements.define('my-component', class extends HTMLElement {
constructor(){
super();
console.log('constructor');
}
connectedCallback(){
console.log('connected');
}
set prop(val){
console.log(`prop set to: ${val}`);
}
static get observedAttributes() {
return ['attr'];
}
attributeChangedCallback(name, oldValue, newValue) {
console.log(`attribute ${name} set to: ${newValue}`);
}
});
const tpl = (scope) => html`
<my-component prop="${scope.prop}" attr="${scope.attr}"></my-conponent>
`;
render(tpl({ prop: 'foo', attr: 'bar' }), $container);
//"constructor"
//"prop set to: foo"
//"attribute attr set to: bar"
//"connected"
IE >= 11 and all evergreens
templates must have valid html markup
attribute value can be set without quotes (<input type="${type}" />
== <input type=${type} />
)
self-closing tags (except for ones from this list) are not supported yet
IE shuffles attributes in a strange manner so execution order might be unexpected (this is importnant to know when using CustomElements
)
calling render
without second attribute generates DocumentFragment
out of template
In real life templates can (and will) be way bigger and more complex so you might want to split them out of js code
For this case there is modulor-html-loader
npm run build
: build the app
npm run test
: test the app
npm run benchmark
: runs node-based benchmarks
npm run benchmark:browser
: runs benchmarks in browser
Found a bug or issue? Please report it
FAQs
Template engine based on tagged template literals
The npm package @modulor-js/html receives a total of 8 weekly downloads. As such, @modulor-js/html popularity was classified as not popular.
We found that @modulor-js/html demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.