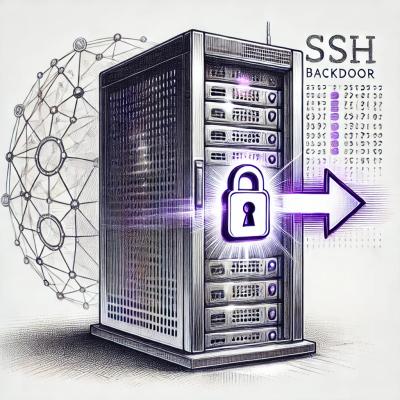
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@mojito-inc/connect-wallet
Advanced tools
Connecting wallet via metamask, wallet connect, email
👨💻 React components for the Mojito Platform, Reference App and third-party projects, including Connect Wallet and Token Gating.
You can install this project with one of these commands:
npm install @mojito-inc/connect-wallet
yarn add @mojito-inc/connect-wallet
yarn add @mui/material@5.11.11 @mui/icons-material@5.11.11 @apollo/client graphql
To config custom theme please follow below steps:
import '@mui/material/styles';
declare module '@mui/material/styles' {
export interface Palette {
backgroundColor?: {
primary?: string
secondary?: string
}
toastError?: ColorPartial
toastSuccess?: ColorPartial
toastWarning?: ColorPartial
iconColor?: string
}
// allow configuration using `createTheme`
export interface PaletteOptions {
backgroundColor?: {
primary?: string
secondary?: string
}
toastError?: ColorPartial
toastSuccess?: ColorPartial
toastWarning?: ColorPartial
iconColor?: string
}
}
Create a new file and place the following code and replace the colors with your custom colors. export the theme and add into the provider.
import { createTheme } from '@mui/material';
export const theme = createTheme({
typography: {
fontFamily: 'Slate',
},
components: {
MuiTextField: {
styleOverrides: {
root: {
'& input::-webkit-outer-spin-button, & input::-webkit-inner-spin-button':
{
display: 'none',
},
'& input[type=number]': {
MozAppearance: 'textfield',
},
},
},
},
MuiCssBaseline: {
styleOverrides: `
@font-face {
font-family: "Slate";
font-style: normal;
font-display: swap;
font-weight: 400;
text-transform: none;
font-size: 16px;
}
`,
},
MuiButton: {
styleOverrides: {
root: {
fontFamily: 'Slate',
textTransform: 'none',
borderRadius: '4px',
fontWeight: 700,
fontSize: '16px',
backgroundColor: '#FDCC35',
color: '#000',
'&:hover': {
backgroundColor: '#FDCC35',
color: '#000',
opacity: 0.5,
},
},
},
},
MuiDialog: {
styleOverrides: {
paper: {
border: '1px solid #D7D8DB',
boxShadow: '0px 8px 16px rgba(0, 0, 0, 0.08)',
borderRadius: '4px',
},
},
},
MuiDivider: {
variants: [
{
props: { orientation: 'horizontal' },
style: {
':before': {
borderTop: 'thin solid #D7D8DB',
},
':after': {
borderTop: 'thin solid #D7D8DB',
},
},
},
],
},
},
palette: {
primary: {
main: '#FDCC35',
},
secondary: {
main: '#356045',
},
backgroundColor: {
primary: '#ffffff',
secondary: '#000000',
},
grey: {
100: '#868b93',
500: '#A1A5AB',
},
toastError: {
500: '#CE2818',
},
iconColor: '#000',
},
});
import { ConnectWalletProvider } from '@mojito-inc/connect-wallet';
const WalletProvider = ({ children }: WalletProviderProps) => {
const [token, setToken] = useState<string>();
const client = useMemo(
() => ({
uri: API_HOSTNAME,
token: token || undefined,
}),
[token],
);
return (
<ConnectWalletProvider
theme={ theme }
clientId={ clientId }
activeChain={ activeChain }
walletConnectProjectId={ walletConnectProjectId }
onAuthenticated={ setToken }
clientOptions={ client }>
{ children }
</ConnectWalletProvider>
);
};
export default WalletProvider;
Param | type | Required | Description |
---|---|---|---|
theme | Theme | ✅ | To Customize the theme |
clientId | string | ✅ | Pass the client id for email wallet |
activeChain | enum | ✅ | pass the network name to connect with email |
walletConnectProjectId | string | ✅ | Pass the wallet connect project id |
onAuthenticated | function | ✅ | in callback you will get an auth token |
clientOptions | object | ✅ | pass the bearer token and api url |
metaData | object | To customize the wallet connect Modal metadata |
After setup ConnectWallet Provider
Following step:
import { ConnectWalletContainer } from '@mojito-inc/connect-wallet';
const ConnectWalletPage = () => {
return (
<ConnectWalletContainer
open={ openModal }
organizationId: organizationId,
walletOptions={{
enableMetamask: true,
enableEmail: true,
enableWalletConnect: true,
}}
customErrorMessage={{
externalWalletConnection: {
wrongWalletMessage: 'Custom Message',
highRiskMessage: 'Custom Message',
defaultMessage: 'Custom Message',
},
}}
image={{
logo: YOUR_LOGO, // pass URL or path, if you are importing an image from local please add ".src" at end
metamask: Images.METAMASK.src,
error: Images.METAMASK.src,
walletConnect: Images.METAMASK.src,
}}
isDisConnect={ disconnect }
isRefetchBalance={ isRefetchBalance }
onCloseModal={ onClickCloseModal } />
);
};
export default ConnectWalletPage;
Param | type | Required | Description |
---|---|---|---|
open | boolean | ✅ | if true it will open modal if false modal will close |
config | Object | ✅ | config |
walletOptions | Object | ✅ | walletOptions |
image | Object | ✅ | image To customize the image |
isDisConnect | boolean | ✅ | if true it will disconnect the wallet |
isRefetchBalance | boolean | if true it will refetch the wallet balance | |
onCloseModal | function | ✅ | To close the Modal |
content | Object | content to customize the title, description in modal | |
isPaperWallet | boolean | if true it will work as paper wallet with email else it will work as thirdweb embedded wallet. | |
isWeb2Login | boolean | if true it will work as web2 login example auth0. | |
skipSignature | boolean | if true it will skip the signature process for connect wallet. | |
userEmail | string | Pass user email address to pre populate email in input fields. | |
customErrorMessage | Object | customErrorMessage to customize the error message |
Param | type | Required | Description |
---|---|---|---|
organizationId | string | ✅ | Pass the organization id |
paperClientId | string | ||
paperNetworkName | enum | paper Network Name |
Param | type | Required | Description |
---|---|---|---|
enableMetamask | boolean | ✅ | |
enableEmail | boolean | ✅ | |
enableWalletConnect | boolean | ✅ |
Param | type | Required | Description |
---|---|---|---|
logo | string | ✅ | |
metamask | string | ✅ | |
error | string | ✅ | |
walletConnect | string | ✅ |
Enum |
---|
Ethereum |
Sepolia |
Goerli |
Polygon |
Mumbai |
Param | type | Required | Description |
---|---|---|---|
walletOptionsContentData | object | ContentData | |
otpContentData | object | ContentData | |
emailContentData | object | ContentData | |
loadingContentData | object | ContentData | |
recoverCodeContentData | object | ContentData |
Param | type | Required | Description |
---|---|---|---|
title | string | ||
description | string |
Param | type | Required | Description |
---|---|---|---|
externalWalletConnection | Object | externalWalletConnection |
Param | type | Required | Description |
---|---|---|---|
wrongWalletMessage | string | customize error for wrongly connect wallet | |
highRiskMessage | string | customize error for highly risk wallet | |
defaultMessage | string | default error |
To get the connected wallet details use these following hooks:
import { useWallet } from '@mojitoinc/mojito-connect-wallet';
const { address, balance } = useWallet();
import { useNetwork } from '@mojitoinc/mojito-connect-wallet';
const { chainID, id, isTestnet, name } = useNetwork();
import { useProvider } from '@mojitoinc/mojito-connect-wallet';
const { provider, providerType } = useProvider();
import { useWalletStatus } from '@mojitoinc/mojito-connect-wallet';
const { disconnectStatus, refetchBalanceStatus, connectStatus } = useWalletStatus();
import { TokenGatingContainer } from '@mojito-inc/connect-wallet';
const TokenGatingPage = () => {
return (
<TokenGatingContainer
onCloseModal={ onCloseModal }
open={ openModal }
config={{
orgId: Configuration.ORG_ID ?? '',
paperClientId: Configuration.PAPER_CLIENT_ID ?? '',
paperNetworkName: Configuration.PAPER_NETWORK_NAME ?? '',
groupId: tokenGatingDetails?.groupId,
ruleId: tokenGatingDetails?.ruleId,
isClaimToken: false,
collectionItemId: tokenGatingDetails?.collectionItemId,
invoiceId,
}}
screenConfig={{
title: ,
subTitle: 'Holders of the Myers Manx collection can now get 50%. Connect your wallet to proceed.',
}}
gatingErrorDetails={ gatingErrorDetails }
walletConnectScreenDetails={ walletConnectScreenDetails }
errorScreenDetails={ errorScreenDetails }
claimTokenScreenDetails={ claimTokenScreenDetails }
setInvoice={ setInvoice } />
)
}
Param | type | Required | Description |
---|---|---|---|
open | Boolean | ✅ | |
config | Object | ✅ | Configuration |
walletConnectScreenDetails | Object | ✅ | walletConnectScreenDetails |
errorScreenDetails | Object | ✅ | errorScreenDetails |
screenConfig | Object | ✅ | screenConfig |
claimTokenScreenDetails | Object | ✅ | claimTokenScreenDetails |
gatingLoaderDetails | Object | ||
gatingErrorDetails | Object | ||
invoiceID | string | ||
onCloseModal | function | ✅ | |
onChangeWalletAddress | function | ||
setInvoice | function | ||
getInvoiceDetails | function |
Param | type | Required | Description |
---|---|---|---|
orgId | string | ✅ | organization id |
chainId | Number | ✅ | |
paperClientId | string | paper client id to connect with email | |
paperNetworkName | any | ||
groupId | string | for Checking Token Gating based on GroupId | |
ruleId | string | for Checking Token Gating based on ruleId | |
isClaimToken | boolean | if true, call mojito claim api | |
collectionItemId | string | ||
invoiceId | string |
Param | type | Required | Description |
---|---|---|---|
title | string | ||
subTitle | string | ||
walletOptions | walletOptions | ✅ | walletOptions |
image | WalletImages | ✅ | WalletImages |
Param | type | Required | Description |
---|---|---|---|
title | string | ||
primaryButtonTitle | string | ||
secondaryButtonTitle | string | ||
tertiaryButtonTitle | string | ||
onClickTertiaryButton | function | ||
onClickSecondaryButton | function | ||
onClickPrimaryButton | function |
Param | type | Required | Description |
---|---|---|---|
title | string | ||
subTitle | string |
Param | type | Required | Description |
---|---|---|---|
tokenDetail | Object | tokenDetail | |
redirectionPageURL | string | ||
onSuccess | function |
Param | type | Required | Description |
---|---|---|---|
tokenImage | string | ||
tokenName | string | ||
tokenSubtitle | string | ||
tokenButtonText | string |
Param | type | Required | Description |
---|---|---|---|
error | string | error icon in svg, png, jpeg or gif format | |
metamask | string | metamask icon in svg, png, jpeg or gif format | |
logo | string | logo icon in svg, png, jpeg or gif format |
Param | type | Required | Description |
---|---|---|---|
enableMetamask | boolean | ✅ | to enable or disable metamask |
enableEmail | boolean | ✅ | to enable or disable email connect |
FAQs
Connecting wallet via metamask, wallet connect, email
We found that @mojito-inc/connect-wallet demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.