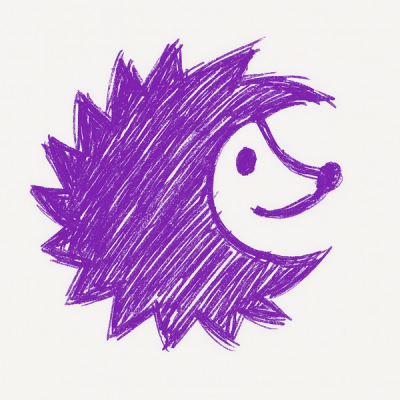
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@ms-cloudpack/worker-pool
Advanced tools
Provides utilities for simplifying worker execution.
It can manage either worker threads or child processes depending on the workerType
option.
Code using the worker pool has two parts:
.js
file which hosts the code to be executed within the workerFor worker reuse to work properly, the method(s) being run must not have any async/scheduled code which continues running after the result is returned. Otherwise, if the scheduled code causes an error after the method returns, the error will either be swallowed (if no method was running in the worker at the time) or associated with the wrong method call (if another method call has been started).
If running arbitrary external code in a worker, it's recommended to overwrite all methods for async scheduling (setTimeout
etc) with no-op versions.
Also be mindful of any cleanup of global state which might need to happen in between runs. This can be done with afterEach
(see below).
First, let's set up the worker entry that will execute work inside the worker. Use initializeWorker
to set up your api surface:
workerEntry.ts
:
import { initializeWorker } from '@ms-cloudpack/worker-pool';
import { methodA, methodB } from './methods';
// Load any environmental side effects here.
// Then initialize the worker with the appropriate listeners and api dictionary.
initializeWorker({
beforeEach: () => {...}, // optional
afterEach: () => {...}, // optional
methods: {
methodA,
methodB,
},
});
In the host where we want to execute things to asynchronously run in the pool of worker threads:
import { WorkerPool } from '@ms-cloudpack/worker-pool';
import path from 'path';
import { fileURLToPath } from 'url';
// or use __dirname if not using ES modules
const dirname = path.dirname(fileURLToPath(import.meta.url));
const pool = new WorkerPool({
entryPath: path.join(dirname, 'workerEntry.js'),
});
Worker threads are recommended due to their better performance. If the child operation specifically requires a new Node process with command line arguments or custom options, use workerType: 'process'
:
const pool = new WorkerPool({
entryPath: path.join(dirname, 'processEntry.js'),
workerType: 'process',
// fill in as needed
childProcessArgs: [],
childProcessOptions: {},
});
Work is executed from the pool created above:
const result = await pool.execute({
method: 'methodA',
args: [arg1, arg2, etc],
});
FAQs
General worker pool helper.
We found that @ms-cloudpack/worker-pool demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.