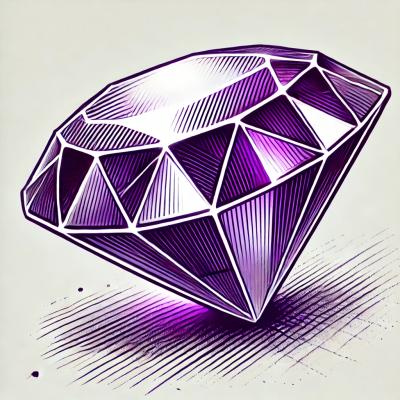
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@mtproto/core
Advanced tools
Telegram API (MTProto) client library for browser
yarn add @mtproto/core -E
# or
npm i @mtproto/core -E
It is not recommended to use it in production.
<script src="https://cdn.jsdelivr.net/npm/@mtproto/core@1.2.0/dist/mtproto.min.js"></script>
You need api_id and api_hash. If you do not have them yet, then get them according to the official instructions: creating your Telegram application.
const MTProto = require('@mtproto/core');
const api_id = 'YOU_API_ID';
const api_hash = 'YOU_API_HASH';
// 1. Create an instance
const mtproto = new MTProto({
api_id,
api_hash,
// Use test servers
test: true,
});
// 2. Get the user country code
mtproto.call('help.getNearestDc').then(result => {
console.log(`country:`, result.country);
});
// https://core.telegram.org/api/auth#test-phone-numbers
const phone = '+9996621111';
const code = '22222';
mtproto
.call('auth.sendCode', {
phone_number: phone,
settings: {
_: 'codeSettings',
},
})
.then(result => {
mtproto
.call('auth.signIn', {
phone_code: code,
phone_number: phone,
phone_code_hash: result.phone_code_hash,
})
.then(result => {
console.log(`auth.signIn[result]:`, result);
})
.catch(error => {
if (error.error_message === 'SESSION_PASSWORD_NEEDED') {
// Need use 2FA
// https://github.com/alik0211/mtproto-core#2fa-two-factor-authentication
}
});
});
const { getSRPParams } = require('@mtproto/core');
const password = 'YOU_PASSWORD';
mtproto
.call('account.getPassword')
.then(async result => {
const { srp_id, current_algo, srp_B } = result;
const { salt1, salt2, g, p } = current_algo;
const { A, M1 } = await getSRPParams({
g,
p,
salt1,
salt2,
gB: srp_B,
password,
});
return mtproto.call('auth.checkPassword', {
password: {
_: 'inputCheckPasswordSRP',
srp_id,
A,
M1,
},
});
})
.then(result => {
console.log(`auth.checkPassword[result]:`, result);
});
mtproto.call(method, options) => Promise
Select method and options from methods list. Promise.then
contain result. Promise.catch
contain error with the error_code
and error_message
properties.
Example:
mtproto.call('help.getNearestDc').then(result => {
console.log(`result:`, result);
}).catch(error => {
console.log(`error:`, error);
});
mtproto.updates.on(UpdatesName, handler)
Authorized users are being Updates. They can be handled using mtproto.updates.on
. Example of handling a updateShort with updateUserStatus:
mtproto.updates.on('updateShort', message => {
const { update } = message;
if (update._ === 'updateUserStatus') {
const { user_id, status } = update;
console.log(`User with id ${user_id} change status to ${status}`);
}
});
MTProto.getSRPParams({ g, p, salt1, salt2, gB, password }) => { A, M1 }
For more information about parameters, see the article on the Telegram website.
Example in 2FA (Two-factor authentication)
FAQs
Telegram API JS (MTProto) client library for Node.js and browser
The npm package @mtproto/core receives a total of 1,076 weekly downloads. As such, @mtproto/core popularity was classified as popular.
We found that @mtproto/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.