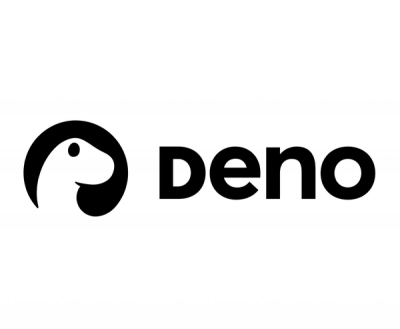
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@mylo.ai/react-native-usebutton
Advanced tools
React native module for the Button's publisher SDK
$ yarn add @mylo.ai/react-native-usebutton
or
$ npm install --save @mylo.ai/react-native-usebutton
$ cd ios && pod install
import ButtonSdk from 'react-native-usebutton';
// Before you do anything you have to configure Button with your appID
const applicationId = Platform.select({
ios: 'app-xxxxxxxxxxxxxxxx',
android: 'app-xxxxxxxxxxxxxxxx',
});
ButtonSdk.identifyButtonApp(applicationId, error => {
// If an error has occured, currently only works on iOS
console.log(error);
});
// Identify a user (on user login)
const userId = 'user-123';
ButtonSdk.setIdentifier(userId);
// Clear all user's data (on user logout)
ButtonSdk.clearAllData();
// Fetch a purchase path from Button
ButtonSDK.startPurchasePath(
{
url: 'https://the.button.url',
token: 'my-tracking-token',
headerTitle: 'My Button Browser Title',
headerSubtitle: 'My Button Browser Subtitle',
headerTitleColor: '#FFFFF',
headerSubtitleColor: '#FFFFF',
headerBackgroundColor: '#FFFFF',
headerTintColor: '#FFFFF',
footerBackgroundColor: '#FFFFF',
footerTintColor: '#FFFFF',
},
(error) => {
// If error while fetching the url, you can get it in here!
console.log(error);
}
);
// Track impression
ButtonSdk.setImpressionView({
url: 'https://the.button.url',
visibleRateType: ButtonSdk.RATE_TYPE.PERCENT,
creativeType: ButtonSdk.CREATIVE_TYPE.HERO,
visibleRate: 7,
});
// Event when the browser is closed
ButtonSdk.addEventListener(
ButtonSdk.EVENT.ON_BROWSER_CLOSED
onHandlePurchaseClosed
);
// Remove event listener
ButtonSdk.removeEventListener(
ButtonSdk.EVENT.ON_BROWSER_CLOSED
onHandlePurchaseClosed
);
startPurchasePath
Property | Description | Required | Default |
---|---|---|---|
url | The merchant's url to fetch | true | None |
token | A unique token that can be usefull for tracking a campaign for example | false | None |
headerTitle | The title displayed in the header | true | None |
headerSubtitle | The subitle displayed in the header | true | None |
headerTitleColor | The title's color | false | rgb(255, 255, 255) |
headerSubtitleColor | The subtitle's color | false | rgb(255, 255, 255) |
headerBackgroundColor | The header's background color | false | rgb(0,0,0) |
headerTintColor | The header's tint color | false | rgb(255, 255, 255) |
footerBackgroundColor | The footer's background color | false | rgb(0,0,0) |
footerTintColor | The footer's tint color | false | rgb(255, 255, 255) |
setImpressionView
Property | Description | Required | Default |
---|---|---|---|
url | The merchant's url to track | true | None |
visibleRateType | The rate type : could be percent or fixed | true | None |
creativeType | The creative type | false | CREATIVE_TYPE.HERO |
visibleRate | The rate the user will be granted | true | None |
Property | Description |
---|---|
RATE_TYPE.PERCENT | The rate type is percentage |
RATE_TYPE.FIXED | The rate type is a fixed amount |
Property | Description |
---|---|
CREATIVE_TYPE.HERO | The hero creative type |
CREATIVE_TYPE.CAROUSEL | The carousel creative type |
CREATIVE_TYPE.LIST | The list creative type |
CREATIVE_TYPE.GRID | The grid creative type |
CREATIVE_TYPE.HERO | The hero creative type |
CREATIVE_TYPE.OTHER | The other creative type |
Property | Description |
---|---|
EVENT.ON_BROWSER_CLOSED | The user has closed the webview |
Here a list of the differrent types available:
interface ButtonEvent {
ON_BROWSER_CLOSED: 'ON_BROWSER_CLOSED';
}
interface RateType {
FIXED: 'FIXED';
PERCENT: 'PERCENT';
}
interface CreativeType {
HERO: 'HERO';
CAROUSEL: 'CAROUSEL';
LIST: 'LIST';
GRID: 'GRID';
DETAIL: 'DETAIL';
OTHER: 'OTHER';
}
interface PurchasePathOptions {
url: string;
token?: string;
headerTitle: string;
headerSubtitle: string;
headerTitleColor?: string;
headerSubtitleColor?: string;
headerBackgroundColor?: string;
headerTintColor?: string;
footerBackgroundColor?: string;
footerTintColor?: string;
}
interface ImpressionViewOptions {
url: string;
visibleRateType: IRateType;
visibleRate: number;
offerId?: string;
creativeType?: ICreativeType;
}
type ErrorCallback = (error: string) => void;
The Button's SDK can cause your Android app not to be able to connect to the metro server, once installed.
To resolve this, you have to add in the directory android/app/src/main/res/xml of your application, a file named network_security_config.xml and copy/paste this content in it:
<?xml version="1.0" encoding="utf-8"?>
<network-security-config>
<domain-config cleartextTrafficPermitted="true">
<domain includeSubdomains="true">localhost</domain>
</domain-config>
</network-security-config>
Then in your AndroidManifest, add this android:networkSecurityConfig="@xml/network_security_config"
inside the application tag.
It has been implemented in the Example app, if you want to see more.
To run the example app :
$ yarn bootstrap #Install all dependencies (node_modules and pods)
$ cd example && yarn start #Run the metro instance
$ cd .. && yarn example ios/android #Run the application on a simulator
Contribution and development instructions can be found in CONTRIBUTING
MIT
FAQs
React native module for the Button SDK
The npm package @mylo.ai/react-native-usebutton receives a total of 0 weekly downloads. As such, @mylo.ai/react-native-usebutton popularity was classified as not popular.
We found that @mylo.ai/react-native-usebutton demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 17 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.