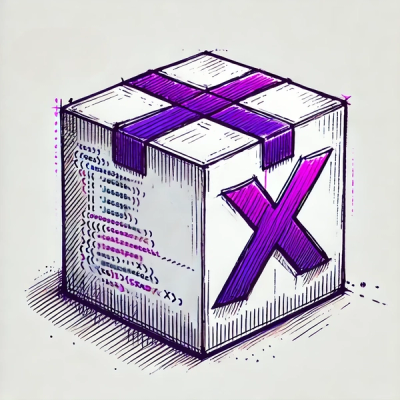
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@myparcel/checkout
Advanced tools
This is the MyParcel delivery options module for use in any e-commerce platform's checkout. It's used to show your customers the possible delivery and/or pickup options for their location, based on your settings. It only has the bare minimum css styling so it should integrate with the design of your webshop easily.
This app is written in Vue.js, it supports IE9 and up.
An example of the delivery options functionality can be found in our sandbox. Here you can try out every combination of settings and copy the code for use in your project.
$ npm i
$ npm run build
dist/myparcel.js
in your project.<div id="myparcel-delivery-options"></div>
in your HTML.window.MyParcelConfig = {
config: {
platform: 'belgie',
carriers: ['bpost', 'dpd'],
// All settings below can be overridden per carrier via the carrierSettings object
// The price for each option
priceMorningDelivery: 7.95,
priceStandardDelivery: 5.85,
priceEveningDelivery: 6.25,
priceSignature: 0.35,
priceOnlyRecipient: 0.30,
pricePickup: 5.85,
// Shipment options
allowSaturdayDelivery: true,
allowPickupLocations: true,
allowSignature: true,
// Other settings
dropOffDays: '1;2;3;4;5;6',
cutoffTime: '15:00',
deliveryDaysWindow: 4,
dropOffDelay: 1,
// Use this object to override settings per carrier.
carrierSettings: {
bpost: {
deliveryDaysWindow: 7,
},
dpd: {},
},
},
strings: {
wrongPostalCodeCity: 'Zaterdaglevering',
saturdayDeliveryTitle: 'Combinatie postcode/plaats onbekend',
// Address strings
city: 'Plaats',
postcode: 'Postcode',
houseNumber: 'Huisnummer',
addressNotFound: 'Adresgegevens niet ingevuld',
// Delivery moment titles.
// If any of these is not set, the delivery time will be visible instead.
deliveryEveningTitle: 'Avondlevering',
deliveryMorningTitle: 'Ochtendlevering',
deliveryStandardTitle: 'Standaardlevering',
deliveryTitle: 'Bezorgen op',
pickupTitle: 'Afhalen op locatie',
// Shipment options
onlyRecipientTitle: 'Home address only',
signatureTitle: 'Handtekening',
pickUpFrom: 'Afhalen vanaf',
openingHours: 'Openingstijden',
// Other strings
closed: 'Gesloten',
discount: 'korting',
free: 'Gratis',
from: 'Vanaf',
loadMore: 'Laad meer',
retry: 'Opnieuw',
},
address: {
cc: 'BE',
city: 'Antwerpen',
postalCode: '2000',
}
};
When there is no title set for deliveryMorningTitle
, deliveryStandardTitle
or deliveryEveningTitle
, the delivery time will automatically be visible. For more in-depth explanation of each config item and string and an interactive demo please see our sandbox
To get the object with the selected options from the delivery options do the following:
const data = document.querySelector('#mypa-input').value;
const obj = JSON.parse(data);
// `obj` will be something like this:
// {
// "delivery": "deliver",
// "deliveryDate": "8-8-2019",
// "deliveryMoment": "standard",
// "shipmentOptions": {signature: true, only_recipient: false}
// }
These examples assume you've already loaded the delivery options in your page. See Installation if you haven't.
You have to provide a configuration file in the following format as window.MyParcelConfig
and initialize the delivery options with an event.
This is an example.
window.MyParcelConfig = {
config: {
platform: 'belgie', // REQUIRED!
carrierSettings: {
bpost: {
allowDeliveryOptions: true,
allowPickupLocations: true,
},
dpd: {
allowDeliveryOptions: true,
allowPickupLocations: true,
},
},
},
address: {
cc: 'BE',
city: 'Antwerpen',
postalCode: '2000',
},
};
// Trigger this event on the document to tell the delivery options to update.
// Usually only needed on initializing and when the address changes.
document.dispatchEvent(new Event('myparcel_update_delivery_options'));
/**
* Get data from form fields and put it in the global MyParcelConfig.
*/
function updateAddress() {
window.MyParcelConfig.address = {
cc: document.querySelector('#country').value,
postalCode: document.querySelector('#house_number').value,
number: document.querySelector('#postcode').value,
city: document.querySelector('#address_1').value,
};
/*
* Send the event that tells the delivery options module to reload data.
*/
document.dispatchEvent(new Event('myparcel_update_delivery_options'));
// IE9–11 compatible example
var event = document.createEvent('HTMLEvents');
event.initEvent('myparcel_update_delivery_options', true, false);
document.querySelector('body').dispatchEvent(event);
}
// ES6 example, use var for older environments.
const addressFields = [
'<Country field selector>',
'<Postal code field selector>',
'<Address line 1 field selector>',
];
addressFields.forEach((field) => {
document.querySelector(field).addEventListener('change', updateAddress);
});
addressFields
the window.MyParcelConfig
will be updated and the delivery options module will receive the event that tells it to update. The delivery options will reload and fetch the available options for the new address.You'll often want to use the delivery options module in a checkout form in your webshop software. We have implemented it in WooCommerce and Magento2 using the following method to get its data in the $_POST
variable on submitting the form.
npm install
npm run serve
If you're experiencing trouble with the implementation we're ready to help you out! Please reach out to us via support@sendmyparcel.be or join our support community on Slack.
FAQs
MyParcel delivery options module for checkouts.
We found that @myparcel/checkout demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.