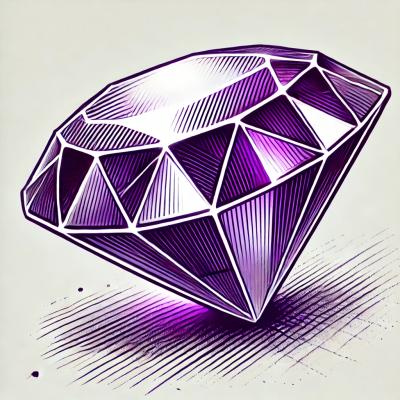
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@mysten/sui.js
Advanced tools
This is the Sui TypeScript SDK built on the Sui JSON RPC API. It provides utility classes and functions for applications to sign transactions and interact with the Sui network.
Note that the SDK is still in development mode and some API functions are subject to change.
$ yarn add @mysten/sui.js
Follow the JSON RPC doc to start a local network and local RPC server
The JsonRpcProvider
class provides a connection to the JSON-RPC Server and should be used for all read-only operations. For example:
Fetch objects owned by the address 0xbff6ccc8707aa517b4f1b95750a2a8c666012df3
import { JsonRpcProvider } from '@mysten/sui.js';
const provider = new JsonRpcProvider('https://gateway.devnet.sui.io:9000/');
const objects = await provider.getOwnedObjectRefs(
'0xbff6ccc8707aa517b4f1b95750a2a8c666012df3'
);
Fetch transaction details from a transaction digest:
import { JsonRpcProvider } from '@mysten/sui.js';
const provider = new JsonRpcProvider('https://gateway.devnet.sui.io:9000/');
const txn = await provider.getTransaction(
'6mn5W1CczLwitHCO9OIUbqirNrQ0cuKdyxaNe16SAME='
);
For any operations that involves signing or submitting transactions, you should use the Signer
API. For example:
To transfer a Coin:
import { Ed25519Keypair, JsonRpcProvider, RawSigner } from '@mysten/sui.js';
// Generate a new Keypair
const keypair = new Ed25519Keypair();
const signer = new RawSigner(
keypair,
new JsonRpcProvider('https://gateway.devnet.sui.io:9000/')
);
const txn = await signer.transferCoin({
signer: keypair.getPublicKey().toSuiAddress(),
objectId: '0x5015b016ab570df14c87649eda918e09e5cc61e0',
gasPayment: '0x0a8c2a0fd59bf41678b2e22c3dd2b84425fb3673',
gasBudget: 10000,
recipient: '0xBFF6CCC8707AA517B4F1B95750A2A8C666012DF3',
});
To sign a raw message: TODO
FAQs
Sui TypeScript API(Work in Progress)
The npm package @mysten/sui.js receives a total of 10,668 weekly downloads. As such, @mysten/sui.js popularity was classified as popular.
We found that @mysten/sui.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.