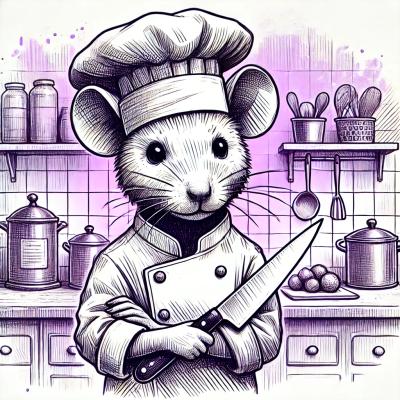
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@namecheap/error-extender
Advanced tools
Simplifies creation of custom Error
classes for Node.js and Browser!
...which then produces stack
with appended stacks of supplied cause
(very much like in Java)!
const extendError = require('@namecheap/error-extender');
const CustomError = extendError('CustomError');
const rootCause = new Error('the root cause');
console.log(new CustomError({ message: 'An error has occurred.', cause: rootCause }));
Shall output:
CustomError: An error has occurred.
at Object.<anonymous> (/opt/app/index.js:7:13)
at Module._compile (internal/modules/cjs/loader.js:702:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10)
at Module.load (internal/modules/cjs/loader.js:612:32)
at tryModuleLoad (internal/modules/cjs/loader.js:551:12)
at Function.Module._load (internal/modules/cjs/loader.js:543:3)
at Function.Module.runMain (internal/modules/cjs/loader.js:744:10)
at startup (internal/bootstrap/node.js:240:19)
at bootstrapNodeJSCore (internal/bootstrap/node.js:564:3)
Caused by: Error: the root cause
at Object.<anonymous> (/opt/app/index.js:5:19)
at Module._compile (internal/modules/cjs/loader.js:702:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10)
at Module.load (internal/modules/cjs/loader.js:612:32)
at tryModuleLoad (internal/modules/cjs/loader.js:551:12)
at Function.Module._load (internal/modules/cjs/loader.js:543:3)
at Function.Module.runMain (internal/modules/cjs/loader.js:744:10)
at startup (internal/bootstrap/node.js:240:19)
at bootstrapNodeJSCore (internal/bootstrap/node.js:564:3)
Oh, by the way, 100% test coverage. See for yourself (via npm test
)!
It's quite simple! See below:
const extendError = require('@namecheap/error-extender');
const AppError = extendError('AppError'); // extends `Error` (default)
Or... A bit more complex using the second argument (options):
const extendError = require('@namecheap/error-extender');
const AppError = extendError('AppError', {
defaultMessage: 'An unhandled error has occurred.',
defaultData: { status: 503, message: 'An unhandled error has occurred.' }
});
const ServiceError = extendError('ServiceError', {
parent: AppError, // extends `AppError`
defaultMessage: 'A service error has occurred.',
defaultData: { status: 500, message: 'A service error has occurred.' }
});
const DatabaseError = extendError('DatabaseError', {
parent: ServiceError, // extends `ServiceError`
defaultMessage: 'A service database error has occurred.',
defaultData: { message: 'A service database error has occurred.' }
});
require('assert').deepStrictEqual(
DatabaseError.defaultData, {
status: 500,
message: 'A service database error has occurred.'
});
// no error
Yes, defaultData
merges!
error-extender
Argumentserror-extender
accepts a single object literal as second argument.
The options (object literal keys) are as follows:
key | expected type |
---|---|
parent | Error.prototype or one that extends it |
defaultMessage | string |
defaultData | any |
Error
classes (child/subclass) : "Extended Errors".cause
(Error
); very much like how it is with Java Exception
.cause
to the bottom (or top) of instantiated "Extended Errors" stack.new
):
new ExtendedError(options)
ExtendedError(options)
Yes, much like JavaScript's native Error
, "Extended Errors" can be written/used "factory-like" (without the new
keyword).
"Extended Errors" accepts a single object literal as argument:
const extendError = require('@namecheap/error-extender');
const ServiceError = extendError('ServiceError');
try {
// ... something throws something
} catch (error) {
throw new ServiceError({
message: 'An error has occurred',
data: { ref: '7e9f876ca116' },
cause: error
});
}
The options (object literal keys) are as follows:
key | alias | expected type |
---|---|---|
message | m | string |
data | d | any |
cause | c | instancedof Error |
Given the alias, you may construct extended errors by:
const extendError = require('@namecheap/error-extender');
const ServiceError = extendError('ServiceError');
try {
// ... something throws something
} catch (error) {
throw new ServiceError({
m: 'An error has occurred',
d: { ref: '7e9f876ca116' },
c: error
});
}
Note: Aliases are evaluated first; hence if you have both m
and message
, if m
's value is truthy, then m
's value will be used.
As with Error
, "Extended Errors" would have the following properties:
name
message
stack
... "Extended Errors" shall have the following additiona properties:
data
- (as set in constructor args)cause
- (as set in constructor args)data
merging w/ defaultData
Yes, you heard right, instance data
merges with defaultData
!!!
See example below:
const extendError = require('@namecheap/error-extender');
const AppError = extendError('ServiceError', {
defaultData: { status: 503, message: 'An unhandled error has occurred.' }
});
const appError = new AppError({ d: { status: 401 } });
require('assert').deepStrictEqual(
appError.data, {
status: 401,
message: 'An unhandled error has occurred.'
});
// no error
bluebird
!):const Promise = require('bluebird');
// ...
const extendError = require('@namecheap/error-extender');
// ...
const ServiceError = extendError('ServiceError');
const ServiceStateError = extendError(
'ServiceStateError',
{ parent: ServiceError });
// ...
function aServiceFunction() {
return new Promise(
function (resolve, reject) {
// ... multiple things that may throw your
// custom "expected" errors
})
.catch(ServiceStateError, function (error) {
// ... your "common way" of handling
// ServiceStateError
// ... then propagate
})
.catch(ServiceError, function (error) {
// ... your "common generc way" of handling
// ServiceError
// ... then propagate
})
.catch(function (error) {
// ... the "catch all"
// ... then propagate
});
}
With JavaScript, I felt quite stifled when I was limited to:
throw new Error('..')
.With error-extender
with help from syntactic-sugar from bluebird
, you can improve (or even standardize) your way of propagating/handling errors throughout your application.
callers.
FAQs
error-extender
We found that @namecheap/error-extender demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.