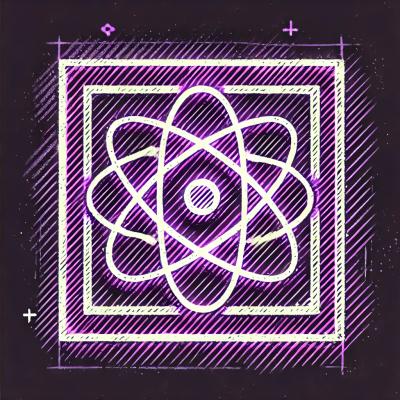
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@nasa-jpl/explorer-1
Advanced tools
Packaged frontend assets for JPL's design system, Explorer 1
This package aims to include all of the frontend assets (JS and SCSS) necessary to create components using the HTML markup examples in the Explorer 1 Storybook.
@nasa-jpl/explorer-1/
├── dist/
│ ├── css/
│ │ ├── explorer-1.min.css
│ │ └── font-face.css
│ ├── fonts/
│ │ ├── archivo-narrow/
│ │ └── metropolis/
│ └── js/
│ └── explorer-1.min.js
├── src/
│ ├── fonts/
│ ├── js/
│ └── scss/
└── tailwind.config.js
This package includes the base styles of Explorer 1 (typography, colors, spacing, etc.) which can be used in your projects via the TailwindCSS classes that are generated by Explorer 1's TailwindCSS configuration. Learn more about how to use TailwindCSS.
Not all components are included. Components will be added as needed by DesignLab's priority projects. If there is a component you would like to have included, please submit a feature request.
List of included components:
components: [
'AnimationCaret',
'ArticleCarousel',
'ArticleCarouselItem',
'BaseButton',
'BaseCarouselCards',
'BaseHeading',
'BaseImage',
'BaseImageCaption',
'BaseImagePlaceholder',
'BaseLink',
'BasePlaceholder',
'BlockIframeEmbed',
'BlockInlineImage',
'BlockImage',
'BlockImageCarousel',
'BlockImageCarouselItem',
'BlockImageGallery',
'BlockKeyPoints',
'BlockQuote',
'BlockRelatedLinks',
'BlockTable',
'BlockTeaser',
'BlockText',
'BlockVideoEmbed',
'HeroFeature',
'HeroFocalPoint',
'HeroMedia',
'Icons',
'RoboticsDetailFacts',
'RoboticsDetailFactsItem',
'SearchResultCard',
],
npm install @nasa-jpl/explorer-1
Include all styles and scripts by adding the bundled CSS and JS to your project's HTML:
<!-- CSS -->
<link href="/path/to/explorer-1.min.css" rel="stylesheet" />
<!-- JavaScript -->
<script src="/path/to/explorer-1.min.js"></script>
(You will need to get the two files out of the installed package in node_modules/@nasa-jpl/explorer-1/dist/
, place them somewhere in your project, and update the paths to point to the right location.)
Then reference the Explorer 1 Storybook for HTML snippets you can use to create components.
The bundled CSS references fonts with the relative path of ../fonts/
. You will need to add the fonts to your build process to ensure they are included in the relative path. An example of how to handle this is to write a Node script that copies the /dist/fonts/
folder to the correct path in your project.
your-project
├── css/
│ ├── explorer-1.min.css
└── fonts/
├── archivo-narrow/
└── metropolis/
Note: if you are using explorer-1.min.css
, then you do not need to include font-face.css
. The font face stylesheet is provided for including font styles a la carte.
Instead of including all of the bundled CSS and JS, you can import individual assets as needed. Below are some examples:
// a la carte component SCSS
@import '@nasa-jpl/explorer-1/src/scss/components/_BasePlaceholder';
// a la carte JS
require('@nasa-jpl/explorer-1/src/js/_detect-ie.js')
You can also include the font-face styles on their own. This is often desireable to improve font loading performance. To do this, use the font-face.css
stylesheet in dist/css/
with the fonts
folder using the same relative path structure as in dist
:
your-project
├── css/
│ ├── font-face.css
└── fonts/
├── archivo-narrow/
└── metropolis/
Below is an example of how to use the Explorer 1 TailwindCSS config in your own TailwindCSS config file. This can be useful if you want to set custom purge settings or any other overrides:
// custom-app/tailwind.config.js
// import Explorer 1's Tailwind config
const explorer1Config = require('@nasa-jpl/explorer-1/tailwind.config.js')
module.exports = {
...explorer1Config,
purge: ['../**/*.html'], // this will override Explorer 1's purge settings
}
Learn more about TailwindCSS configuration
Documentation on how to run this project locally and add more components.
npm i
npm run dev
Note: You may need to refresh if you've never run the project before.dist/
npm run build
Refer to and maintain the list of included components. Eventually this list will be derived from Storybook rather than maintained manually.
Adding a component requires the following:
Storybook serves as the documentation for how to use the design system's foundational styles and the included components. New components can also be developed directly in Storybook.
Create a folder: all stories live in /storybook/stories/
. If you are adding a component, create a new folder for your component in /storybook/stories/components/
.
Create a template file: create a file for your HTML template. The filename should match your component name, e.g. MyComponent.js
. It should include one exported constant, named with your components name, and appended with Template
. It will look something like this:
// MyComponent.js
export const MyComponentTemplate = () => {
return `<div class="MyComponent"><!-- more html markup --></div>`
}
You could make your component reactive by passing arguments:
// MyComponent.js
export const MyComponentTemplate = ({ text }) => {
return `<div class="MyComponent">${text}</div>`
}
Add stories for your component: In the same folder, create your stories file. The filename should be your component name appended with .stories.js
, e.g. MyComponent.stories.js
. This file will import your HTML template and configure the stories that will be displayed. We are using the Component Story Format (CSF) to write our stories. Below is an example of CSF boilerplate for a component that has one argument:
// MyComponent.stories.js
import { MyComponentTemplate } from './MyComponent.js'
export default {
// the title also determines where the story will appear in the sidebar
title: 'Components/MyComponent',
// argTypes are optional
argTypes: {
text: {
type: 'string',
description: 'Text to display within the component',
},
},
// parameters are optional
parameters: {
viewMode: 'docs', // default to docs instead of canvas
docs: {
description: {
component:
'A brief description of the component. This will appear at the top of the docs page',
},
},
},
}
// Each export is a story. A minimum of one is required
export const StoryName1 = MyComponentTemplate.bind({})
StoryName1.args = { text: 'Example text 1' }
export const StoryName2 = MyComponentTemplate.bind({})
StoryName2.args = { text: 'Example text 2' }
CSF is a flexible format that allows for adding more controls and documentation to your stories. Please read the storybook documentation to learn more about CSF.
If your component reuses other components (e.g. if you were building a dialog box component that uses BaseButton
), you can also do the same when creating your storybook template. Here is an example component template that imports MyComponent
and reuses its template:
// AnotherComponent.js
import { MyComponentTemplate } from 'path/to/MyComponent.js'
export const AnotherComponentTemplate = () => {
const MyComponent = MyComponentTemplate({
text: 'Custom text for MyComponent',
})
return `<div class="AnotherComponent">
<p>Another component that reuses MyComponent</p>
${MyComponent}
</div>`
}
Decorators are useful if you want to modify the layout of your component without including it in your template or HTML output. To use a decorator, wrap your template with an immediate parent of #storyDecorator
and whatever surrounding layout that is needed. You can then set the html root of your story parameters to use the #storyDecorator
as the root.
// MyComponent.js
export const MyComponentTemplate = () => {
return `<div class="container mx-auto">
<div id="#storyDecorator" class="lg:w-1/2">
<div class="MyComponent"><!-- more html markup --></div>
</div>
</div>`
}
// MyComponent.stories.js
export default {
// parameters are optional
parameters: {
...
html: {
root: '#storyDecorator',
},
...
},
}
As long as parameters.html.root
matches the parent wrapper element of your template, then this will work. #storyDecorator
is recommended for consistency.
SCSS lives in /src/scss/
and Parcel is used to compile all partials and imports. If you are writing SCSS for your component, all SCSS should be scoped by the component name. In general, try to avoid custom SCSS if possible, as most styling can be achieved by using inline TailwindCSS classes.
If you are adding SCSS for component, create a SCSS partial for it in /src/scss/components/
and import it in /src/scss/_components.scss
. Partials for global styles should be imported in /src/scss/styles.scss
. Filenames for component SCSS partials should use CamelCase, e.g. _MyComponent.scss
Below is an example walkthrough of adding SCSS for a new component named MyComponent
:
Create a SCSS partial for your component: /src/scss/components/_MyComponent.scss
Namepsace all styles with your component name:
// _MyComponent.scss
.MyComponent {
@apply some-tailwind-class;
.sub-class {
// more styles
}
}
Import the partial in /src/scss/_components.scss
// _components.scss
@import 'components/MyComponent';
JavaScript lives in /src/js/
and Parcel is used to compile all imports and script files.
You can add more scripts as require()
statements to the /src/js/scripts.js
file. A few files are already included: _detect-ie.js
, _lazysizes_.js
, and _swiper.js
.
Any script that will be required by scripts.js
should start with an underscore. If the script is for a component, it should use the component name CamelCase e.g.: _MyComponent.js
.
If you want to use Node modules, install the package as usual and add the necessary imports directly to scripts.js
or a separate JS file that is then required in scripts.js
. See _lazysizes.js
as an example.
Once you are done adding your component, be sure to update the list of included components.
Ultimately, the diff for adding a new component that required custom SCSS and JavaScript would look something like this (excluding build to dist):
@nasa-jpl/explorer-1/
├── README.md # modified
├── src/
│ ├── js/
│ │ ├── _MyComponent.js # new
│ │ └── scripts.js # modified
│ └── scss/
│ ├── components/
│ │ └── _MyComponent.scss # new
│ └── _components.scss # modified
└── storybook/
└── stories/
└── components/
└── MyComponent/ # new
├── MyComponent.js # new
└── MyComponent.stories.js # new
If any changes were made to the src files, be sure to build to dist before publishing to NPM.
npm login
npm publish
FAQs
Packaged frontend assets for JPL's design system, Explorer 1
The npm package @nasa-jpl/explorer-1 receives a total of 23 weekly downloads. As such, @nasa-jpl/explorer-1 popularity was classified as not popular.
We found that @nasa-jpl/explorer-1 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.