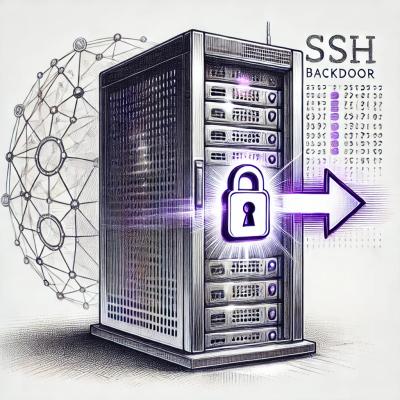
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@neon-exchange/nash-protocol
Advanced tools
Implementation of Nash cryptographic routines
This document is very WIP and should be checked for accuracy by someone with more expertise!
yarn install
yarn build
yarn test
/*
Secure-randomly generates a series of random bytes.
* publicKey is used to verify signatures.
* secretKey is the "master key" used to sign stuff and to generate mnemonic.
Uses crypto-browserify/randombytes which polyfills node crypto.
Spec: SIGGEN()
*/
getEntropy = () => { publicKey: Buffer, secretKey: Buffer }
/*
Converts entropy to wordlist. We can supply our own wordlist for i18n.
This is not an encryption, it's a simple mapping.
Uses bitcoinjs/bip39 implementation.
*/
secretKeyToMnemonic = (secretKey: Buffer) => Array<string>
/*
Converts wordlist back to entropy.
*/
mnemonictoSecretKey = (Array<string>) => Buffer
/*
Creates the master seed which is the foundation of all wallet secret keys.
Use PBKDF2.
Uses bitcoinjs/bip39 implementation.
*/
mnemonicToMasterSeed = (mnemonic: Array<string>) => Buffer
/*
Hashes user password using scrypt with parameters N = 16384, r = 8, p = 1.
Spec: SCRYPT()
Uses scrypt-js implementation.
*/
hashPassword = (password: string) => Promise<Buffer>
/*
Derives two symmetric secret keys from password via HKDF. Uses user ID as salt.
* authKey is stored server side for use in authentication
* encryptionKey is used to encrypt the secret key
Uses futoin-hkdf implementation.
Spec: HKDF()
*/
getHKDFKeysFromPassword = (password: string, salt: string) => Promise<{ authKey: Buffer, encryptionKey: Buffer }>
/*
Encrypts master key using encryptionKey. Uses AEAD. Reversible.
aead is stored server-side.
Uses crypto-browserify/browserify-aes implementation, which polyfills Node `crypto`.
Spec: ENC(), DEC()
*/
interface AEAD = {
encryptedSecretKey: Buffer
nonce: Buffer
tag: Buffer
}
encryptSecretKey = (encryptionKey: Buffer, secretKey: Buffer) => AEAD
decryptSecretKey = (encryptionKey: Buffer, aead: AEAD) => Promise<Buffer>
/*
Regenerates mnemonic. Because the only information the user has is their
password, we have to go through several hash/encrypt/decrypt steps.
aead can come from the server as it is secure.
*/
regenerateMnemonic = (aead: AEAD, password: string): Array<String>
getEntropy()
generates their keys.secretKeyToMnemonic()
. Asynchronously, the master seed is used to generate the master seed with mnemonicToMasterSeed()
.hashPassword()
and getHKDFKeysFromPassword()
. Auth key is sent to server.encryptSecretKey()
. Output is sent to server.aead
which can be decrypted using the encryption key.PBKDF2(mnemonic, passphrase = "")
Iteration = 2048, uses HMAC-SHA512. Should be 512 bits (64 bytes). Used to generate wallet addresses.PBKDF2()
encryption function.We will NOT support the user supplying their own wallet keys. While users will control their own wallets, we will generate the wallets for them. This is partially because we want wallets to be deterministically derivable from the master seed.
Gitlab CI will automatically publish a version if it receives a new Git tag (see also the publish_to_npm
step in .gitlab-ci.yml
).
Here's the specific steps: Start with decide on a new release version, eg. v1.2.3
. Then create a branch and tag and push everything to Gitlab:
# Make sure you are on master and that all work for this release is committed and merged.
# Next step is to create a branch for this release:
git checkout -b release/v1.2.3
# `yarn prepare-release` will do a hard git reset, run the tests and update the version
# number based on the input you provide in the prompt. It also creates a git tag.
yarn prepare-release
git push origin release/v1.2.3
# Based on that branch, create a PR, and as soon as that is in master, push the tag
# that was created with `yarn prepare-release`:
git push origin refs/tags/v1.2.3
# At this point, the CI will run and if successful push to npm
2.0.11 (2019-08-16)
<a name="2.0.9"></a>
FAQs
TypeScript implementation of Nash crypto routines
The npm package @neon-exchange/nash-protocol receives a total of 141 weekly downloads. As such, @neon-exchange/nash-protocol popularity was classified as not popular.
We found that @neon-exchange/nash-protocol demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.