What is @nestjs/common?
The @nestjs/common package is a core part of the NestJS framework, which is a framework for building efficient, reliable and scalable server-side applications. This package provides a wide range of basic functionalities necessary for any NestJS application, including decorators, modules, middleware, filters, pipes, guards, and utilities for handling HTTP requests and responses.
What are @nestjs/common's main functionalities?
Decorators
Decorators are used to annotate classes and their members to define their roles within the application. For example, `@Injectable()` marks a class as a service that can be injected, and `@Controller()` defines a class as a controller with a specific route.
@Injectable()
class MyService {}
@Controller('my-route')
class MyController {
constructor(private myService: MyService) {}
}
Modules
Modules are used to organize components by encapsulating them within bounded contexts. This code sample demonstrates how to define a module that includes controllers and providers.
@Module({
imports: [],
controllers: [MyController],
providers: [MyService],
})
class MyModule {}
Middleware
Middleware are functions that execute during the request-response cycle. They can perform tasks like logging, request validation, etc. This code sample shows a basic middleware that logs a message on each request.
@Injectable()
class MyMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: NextFunction) {
console.log('Request...');
next();
}
}
Exception Filters
Exception filters handle exceptions that occur during the processing of a request. This code sample demonstrates a filter that catches HTTP exceptions and formats the response.
@Catch(HttpException)
class HttpExceptionFilter implements ExceptionFilter {
catch(exception: HttpException, host: ArgumentsHost) {
const ctx = host.switchToHttp();
const response = ctx.getResponse<Response>();
const status = exception.getStatus();
response
.status(status)
.json({
statusCode: status,
timestamp: new Date().toISOString(),
path: ctx.getRequest<Request>().url,
});
}
}
Other packages similar to @nestjs/common
express
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. While it serves a similar purpose in building server-side applications, it is less opinionated than NestJS and does not provide the same level of structure and abstraction out of the box.
koa
Koa is a new web framework designed by the team behind Express, aiming to be a smaller, more expressive, and more robust foundation for web applications and APIs. Koa uses async functions, which allows for a simpler and more powerful way to handle asynchronous operations compared to Express. However, like Express, it is less opinionated than NestJS.
hapi
Hapi is a rich framework for building applications and services, allowing developers to focus on writing reusable application logic instead of spending time building infrastructure. It is designed to be more configurable and extensible than Express, offering a more comprehensive set of features out of the box. However, it still lacks the full-fledged module system and decorators provided by NestJS.
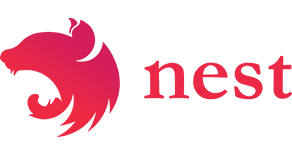
Modern, powerful web application framework for Node.js.

Description
Nest is a powerful web framework for Node.js, which helps you effortlessly build efficient, scalable applications. It uses modern JavaScript, is built with TypeScript and combines best concepts of both OOP (Object Oriented Progamming) and FP (Functional Programming).
It is not just another framework. You do not have to wait for a large community, because Nest is built with awesome, popular well-known libraries - Express and socket.io! It means, that you could quickly start using framework without worrying about a third party plugins.
Installation
Git:
$ git clone https://github.com/kamilmysliwiec/nest-typescript-starter.git project
$ cd project
$ npm install
$ npm run start
NPM:
$ npm i --save @nestjs/core @nestjs/common @nestjs/microservices @nestjs/websockets @nestjs/testing reflect-metadata rxjs
Philosophy
JavaScript is awesome. This language is no longer just a trash to create simple animations in the browser. Right now, the front end world is rich in variety of tools. We have a lot of amazing frameworks / libraries such as Angular, React or Vue, which improves our development process and makes our applications fast and flexible.
Node.js gave us a possibility to use this language also on the server side. There are a lot of superb libraries, helpers and tools for node, but non of them do not solve the main problem - the architecture.
We want to create scalable, modern and easy to maintain applications. Nest helps us with it.
Features
- Easy to learn - syntax is similar to Angular
- Compatible with both TypeScript and ES6 (I strongly recommend to use TypeScript)
- Based on well-known libraries (Express / socket.io) so you could share your experience
- Supremely useful Dependency Injection, built-in Inversion of Control container
- Hierarchical injector - increase abstraction in your application by creating reusable, loosely coupled modules with type injection
- Own modularity system (split your system into reusable modules)
- WebSockets module (based on socket.io)
- Reactive microservices support with messages patterns (transport via TCP / Redis)
- Exceptions handler layer
- Testing utilities
Documentation & Quick Start
Documentation & Tutorial
Starter repos
Useful references
People
Backers
I am on a mission to provide an architecture to create truly flexible, scalable and loosely coupled systems using Node.js platform. It takes a lot of time, so if you want to support me, let's become a backer / sponsor. I'll appreciate each help. Thanks!

License
MIT