What is @nestjs/platform-fastify?
@nestjs/platform-fastify is an adapter package for the NestJS framework that allows you to use Fastify as the underlying HTTP server instead of the default Express. Fastify is known for its high performance and low overhead, making it a good choice for applications that require high throughput and low latency.
What are @nestjs/platform-fastify's main functionalities?
Basic Setup
This code demonstrates how to set up a basic NestJS application using Fastify as the HTTP server. The `FastifyAdapter` is passed to the `NestFactory.create` method to initialize the application with Fastify.
const { NestFactory } = require('@nestjs/core');
const { FastifyAdapter } = require('@nestjs/platform-fastify');
const { AppModule } = require('./app.module');
async function bootstrap() {
const app = await NestFactory.create(AppModule, new FastifyAdapter());
await app.listen(3000);
}
bootstrap();
Middleware Integration
This code shows how to integrate middleware in a NestJS application using Fastify. The `app.use` method is used to add a middleware function that logs each incoming request.
const { NestFactory } = require('@nestjs/core');
const { FastifyAdapter } = require('@nestjs/platform-fastify');
const { AppModule } = require('./app.module');
async function bootstrap() {
const app = await NestFactory.create(AppModule, new FastifyAdapter());
app.use((req, res, next) => {
console.log('Request...');
next();
});
await app.listen(3000);
}
bootstrap();
Route Handling
This code demonstrates how to define a simple route in a NestJS application using Fastify. The `ExampleController` class defines a route that responds with 'Hello, Fastify!' when accessed via a GET request.
const { Controller, Get } = require('@nestjs/common');
@Controller('example')
class ExampleController {
@Get()
getExample() {
return 'Hello, Fastify!';
}
}
const { NestFactory } = require('@nestjs/core');
const { FastifyAdapter } = require('@nestjs/platform-fastify');
const { AppModule } = require('./app.module');
async function bootstrap() {
const app = await NestFactory.create(AppModule, new FastifyAdapter());
await app.listen(3000);
}
bootstrap();
Other packages similar to @nestjs/platform-fastify
@nestjs/platform-express
@nestjs/platform-express is the default HTTP server adapter for NestJS, using Express as the underlying server. It is more widely used and has a larger ecosystem of middleware and plugins compared to Fastify.
fastify
Fastify is a standalone web framework for Node.js that focuses on high performance and low overhead. While it can be used independently, it lacks the built-in structure and features of NestJS, such as dependency injection and modular architecture.
express
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It is the most popular web framework for Node.js but does not offer the same level of performance as Fastify.
A progressive Node.js framework for building efficient and scalable server-side applications, heavily inspired by Angular.
Description
Nest is a framework for building efficient, scalable Node.js server-side applications. It uses modern JavaScript, is built with TypeScript (preserves compatibility with pure JavaScript) and combines elements of OOP (Object Oriented Programming), FP (Functional Programming), and FRP (Functional Reactive Programming).
Under the hood, Nest makes use of Express, but also, provides compatibility with a wide range of other libraries, like e.g. Fastify, allowing for easy use of the myriad third-party plugins which are available.
Philosophy
In recent years, thanks to Node.js, JavaScript has become the “lingua franca” of the web for both front and backend applications, giving rise to awesome projects like Angular, React and Vue which improve developer productivity and enable the construction of fast, testable, extensible frontend applications. However, on the server-side, while there are a lot of superb libraries, helpers and tools for Node, none of them effectively solve the main problem - the architecture.
Nest aims to provide an application architecture out of the box which allows for effortless creation of highly testable, scalable, loosely coupled and easily maintainable applications.
Getting started
Support
Nest is an MIT-licensed open source project. It can grow thanks to the sponsors and support by the amazing backers. If you'd like to join them, please read more here.
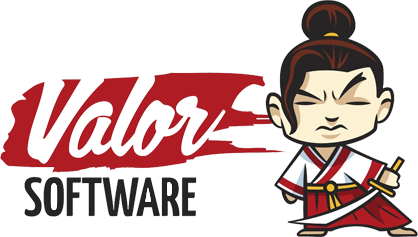



Backers

Stay in touch
License
Nest is MIT licensed.