What is @nestjs/platform-ws?
@nestjs/platform-ws is a package that provides WebSocket support for NestJS applications using the ws library. It allows developers to create WebSocket gateways and handle real-time communication in a structured and scalable way.
What are @nestjs/platform-ws's main functionalities?
WebSocket Gateway
This feature allows you to create a WebSocket gateway using decorators. The `@WebSocketGateway()` decorator marks the class as a WebSocket gateway, and `@WebSocketServer()` injects the WebSocket server instance. The `@SubscribeMessage()` decorator listens for specific messages, and the `handleMessage` method processes incoming messages.
import { WebSocketGateway, WebSocketServer, SubscribeMessage, MessageBody } from '@nestjs/websockets';
import { Server } from 'ws';
@WebSocketGateway()
export class EventsGateway {
@WebSocketServer()
server: Server;
@SubscribeMessage('message')
handleMessage(@MessageBody() data: string): string {
return data;
}
}
Handling WebSocket Events
This feature demonstrates how to handle WebSocket lifecycle events such as initialization, connection, and disconnection. Implementing `OnGatewayInit`, `OnGatewayConnection`, and `OnGatewayDisconnect` interfaces allows you to define methods that are triggered during these events.
import { WebSocketGateway, WebSocketServer, OnGatewayInit, OnGatewayConnection, OnGatewayDisconnect } from '@nestjs/websockets';
import { Server, Socket } from 'ws';
@WebSocketGateway()
export class EventsGateway implements OnGatewayInit, OnGatewayConnection, OnGatewayDisconnect {
@WebSocketServer()
server: Server;
afterInit(server: Server) {
console.log('WebSocket server initialized');
}
handleConnection(client: Socket, ...args: any[]) {
console.log('Client connected:', client.id);
}
handleDisconnect(client: Socket) {
console.log('Client disconnected:', client.id);
}
}
Other packages similar to @nestjs/platform-ws
socket.io
Socket.IO is a popular library for real-time web applications. It provides a full-duplex communication channel over a single TCP connection. Compared to @nestjs/platform-ws, Socket.IO offers more features like automatic reconnection, disconnection detection, and rooms/namespaces, but it is not specifically designed for NestJS.
ws
ws is a simple to use, blazing fast, and thoroughly tested WebSocket client and server for Node.js. It is the underlying library used by @nestjs/platform-ws. While ws provides the core WebSocket functionality, @nestjs/platform-ws integrates it into the NestJS framework, offering decorators and structured event handling.
A progressive Node.js framework for building efficient and scalable server-side applications.
Description
Nest is a framework for building efficient, scalable Node.js server-side applications. It uses modern JavaScript, is built with TypeScript (preserves compatibility with pure JavaScript) and combines elements of OOP (Object Oriented Programming), FP (Functional Programming), and FRP (Functional Reactive Programming).
Under the hood, Nest makes use of Express, but also, provides compatibility with a wide range of other libraries, like e.g. Fastify, allowing for easy use of the myriad third-party plugins which are available.
Philosophy
In recent years, thanks to Node.js, JavaScript has become the “lingua franca” of the web for both front and backend applications, giving rise to awesome projects like Angular, React and Vue which improve developer productivity and enable the construction of fast, testable, extensible frontend applications. However, on the server-side, while there are a lot of superb libraries, helpers and tools for Node, none of them effectively solve the main problem - the architecture.
Nest aims to provide an application architecture out of the box which allows for effortless creation of highly testable, scalable, loosely coupled and easily maintainable applications.
Getting started
Consulting
With official support, you can get expert help straight from Nest core team. We provide dedicated technical support, migration strategies, advice on best practices (and design decisions), PR reviews, and team augmentation. Read more about support here.
Support
Nest is an MIT-licensed open source project. It can grow thanks to the sponsors and support by the amazing backers. If you'd like to join them, please read more here.
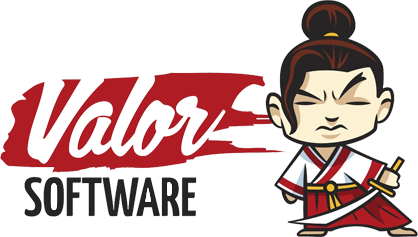


Backers

Stay in touch
License
Nest is MIT licensed.