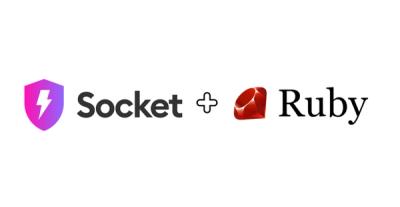
Product
Introducing Ruby Support in Socket
Socket is launching Ruby support for all users. Enhance your Rails projects with AI-powered security scans for vulnerabilities and supply chain threats. Now in Beta!
@nevware21/ts-async
Advanced tools
support for asynchronous development with a Promise based task Scheduler, several different Promise implementations (synchronous, idle, asynchronous and native runtime wrappers), await helpers, and aliases all built and tested using TypeScript.
@nevware21/ts-async is a TypeScript library that provides utilities for working with asynchronous operations. It includes features such as deferred promises, cancellation tokens, and utilities for working with async iterables.
Deferred Promises
Deferred promises allow you to create a promise and resolve or reject it at a later time. This is useful for scenarios where you need to control the timing of the promise resolution.
const { createDeferred } = require('@nevware21/ts-async');
const deferred = createDeferred();
setTimeout(() => {
deferred.resolve('Hello, World!');
}, 1000);
deferred.promise.then((message) => {
console.log(message); // Outputs: Hello, World!
});
Cancellation Tokens
Cancellation tokens provide a way to cancel asynchronous operations. This is useful for scenarios where you need to abort an operation based on certain conditions.
const { createCancellationTokenSource } = require('@nevware21/ts-async');
const cts = createCancellationTokenSource();
async function fetchData(token) {
for (let i = 0; i < 5; i++) {
if (token.isCancellationRequested) {
console.log('Operation cancelled');
return;
}
console.log('Fetching data...');
await new Promise((resolve) => setTimeout(resolve, 1000));
}
console.log('Data fetched');
}
fetchData(cts.token);
setTimeout(() => {
cts.cancel();
}, 2500);
Async Iterables
Async iterables allow you to work with asynchronous sequences of data. This is useful for scenarios where you need to process data that is received over time.
const { createAsyncIterable } = require('@nevware21/ts-async');
async function* asyncGenerator() {
for (let i = 0; i < 5; i++) {
await new Promise((resolve) => setTimeout(resolve, 1000));
yield i;
}
}
const asyncIterable = createAsyncIterable(asyncGenerator);
(async () => {
for await (const value of asyncIterable) {
console.log(value); // Outputs: 0, 1, 2, 3, 4 (one per second)
}
})();
Bluebird is a fully featured promise library with focus on innovative features and performance. It provides utilities for working with promises, including deferred promises and cancellation. Compared to @nevware21/ts-async, Bluebird is more mature and widely used, but it is not TypeScript-specific.
RxJS is a library for reactive programming using Observables, to make it easier to compose asynchronous or callback-based code. It provides powerful utilities for working with asynchronous data streams. Compared to @nevware21/ts-async, RxJS offers a more comprehensive set of features for reactive programming but has a steeper learning curve.
p-cancelable is a library that provides a cancelable promise implementation. It allows you to create promises that can be canceled. Compared to @nevware21/ts-async, p-cancelable is more focused on providing a simple and lightweight solution for cancelable promises.
This package provides support for asynchronous development with a Promise based task Scheduler, several different Promise implementations (synchronous, idle, asynchronous and native runtime wrappers), await helpers, and aliases all built and tested using TypeScript. One of the primary focuses of this package is to provide support for the creation of code that can be better minified, resulting in a smaller runtime payload which can directly assist with Page Load performance.
The Promise based Task Scheduler supports the serialized execution of tasks using promises to control when the next task should be executed (after the previous task completes), this leverages the supplied Promise implementations so that you can easily create an Idle Task Scheduler which executes the tasks using requestIdleCallback
when using the createIdlePromise
implementation.
All of the provided Promise implementations support usage patterns via either
await
or with the included helper functions (doAwait
,doFinally
,doAwaitresponse
), you can also mix and match them (use both helper andawait
) as required by your use cases.
See the documentation generated from source code via typedoc for a full list and details of all of the available types, functions, interfaces with included examples.
See Browser Support for details on the supported browser environments.
Type / Function | Details |
---|---|
Scheduler | |
createTaskScheduler | Create a Task Scheduler using the optional promise implementation and scheduler name. The newPromise can be any value promise creation function, where the execution of the queued tasks will be processed based on how the promise implementation processes it's chained promises (asynchrounsly; synchronously; idle processing, etc) There is no limit on the number of schedulers that you can create, when using more than one scheduler it is recommended that you provide a name to each scheduler to help identify / group promises during debugging. |
Interfaces | |
IPromise | This package uses and exports the IPromise<T> interface which extends both the PromiseLike<T> and Promise<T> to provide type compatibility when passing the Promises created with this package to any function that expects a Promise (including returning from an async function ). As part of it's definition it exposes an [optional] string status to represent the current state of the Promise . These values include pending , resolved rejected and resolving which is a special case when a Promise is being resolved via another Promise returned via the thenable onResolved / onRejected functions. |
AwaitResponse | This interface is used to represent the result whether resolved or rejected when using the doAwaitResponse , this function is useful when you want to avoid creating both a resolved and rejected functions, as this function only requires a single callback function to be used which will receive an object that conforms to this interface. |
Alias | |
createPromise createAllPromise createRejectedPromise createResolvedPromise setCreatePromiseImpl | These function use the current promise creator implementation that can be set via Unless otherwise set this defaults to the createNativePromise implementation, to provide a simplified wrapper around any native runtime Promise support. Available implementation: By using these aliases, it's possible to switch between any of these implementations as needed without duplicating your code. This will not change any existing promises, it only affects new promises. |
createNativePromise createNativeAllPromise createNativeRejectedPromise createNativeResolvedPromise | These are effectively wrappers around the runtime Promise class, so this is effectivly the same as new Promise(...) but as a non-global class name it can be heavily minified to something like a(...) . These wrappers also add an accessible status property for identifying the current status of this promise. However, the status property is NOT available on any returned chained Promise (from calling then , catch or finally ) |
Asynchronous | |
createAsyncPromise createAsyncAllPromise createAsyncRejectedPromise createAsyncResolvedPromise | Provides an implementation of the Promise contract that uses timeouts to process any chained promises (returned by then(...) , catch(...) , finally(...) ), when creating the initial promise you can also provide (override) the default duration of the timeout (defaults to 0ms) to further delay the execution of the chained Promises., but can also be provided) to process any chained promises (of any type) |
Synchronous | |
createSyncPromise createSyncAllPromise createSyncRejectedPromise createSyncResolvedPromise | Also implements the Promise contract but will immediately execute any chained promises at the point of the original promise getting resolved or rejected, or if already resolved, rejected then at the point of registering the then , catch or finally |
Idle | |
createIdlePromise createIdleAllPromise createIdleRejectedPromise createIdleResolvedPromise | Implements the Promise contract and will process any chained promises using the available requestIdleCallback (with no timeout by default - but can also be changes by setDetaultIdlePromiseTimeout ). And when requestIdleCallback is not supported this will default to using a timeout via the scheduleIdleCallback from @nevware21/ts-utils |
Helpers | |
doAwait | Helper which handles await "handling" via callback functions to avoid the TypeScript boilerplate code that is added for multiple branches. Internal it handles being passed an IPromise or a result value so you avoid needing to test whether the result you have is also a Promise .It takes three (3) optional callback functions for resolved , rejected and finally . |
doAwaitResponse | Helper which handles await "handling" via a single callback where resolved and rejected cases are handled by the same callback, this receives an AwaitResponse object that provides the value or reason and a flag indicating whether the Promise was rejected ) |
doFinally | Helper to provide finally handling for any promise using a callback implementation, analogous to using try / finally around an await function or using the finally on the promise directly |
All promise implementations are validated using TypeScript with async
/ await
and the internal helper functions doAwait
, doFinally
and doAwaitResponse
helpers. Usage of the doAwait
or doAwaitResponse
is recommended as this avoids the additional boiler plate code that is added by TypeScript when handling the branches in an async
/ await
operations, this does however, mean that your calling functions will also need to handle this async
operations via callbacks rather than just causing the code path to "halt" at the await
and can therefore may be a little more complex (depending on your implementation).
However, you are NOT restricted to only using
await
ORdoAwait
they can be used together. For example your public API can still be defined as asynchronous, but internally you could usedoAwait
async function myApi()
{
await doAwait(thePromise, (result) => { ... }, (reason) => { ... });
}
All implementations will "emit/dispatch" the unhandled promise rejections event (unhandledRejection
(node) or unhandledrejection
) (if supported by the runtime) using the standard runtime mechanisms. So any existing handlers for native (new Promise
) unhandled rejections will also receive them from the idle
, sync
and async
implementations. The only exception to this is when the runtime (like IE) doesn't support this event in those cases "if" an onunhandledrejection
function is registered it will be called and if that also doesn't exist it will logged to the console (if possible).
The package provides a simple polyfill wrapper which is built around the asynchronous
promise implementation which is tested and validated against the standard native (Promise()
) implementations for node, browser and web-worker to ensure compatibility.
Install the npm packare: npm install @nevware21/ts-async --save
And then just import the helpers and use them.
import { createTaskScheduler } from "@nevware21/ts-async";
import { scheduleTimeout } from "@nevware21/ts-utils";
let scheduler = createTaskScheduler();
// Schedule (and start) task 1 using native promise
// Because this is a new scheduler there are no other
// pending tasks so this will be started synchronously
let task1 = scheduler.queue(() => {
return new Promise((resolve) => {
setTimeout(() => {
resolve(42);
}, 100);
});
});
// task1.status; => "pending" (and running)
// Schedule task 2 using helper function
// The startTask function for task2 will not be called
// until task1 has completed
let task2 = scheduler.queue(() => {
return createPromise((resolve) => {
scheduleTimeout(() => {
resolve(21);
}, 100);
});
});
// task2.status; => "pending" (not running)
await task1;
// task1.status => "resolved"
// task2.status => "pending" (but running)
import { createPromise } from "@nevware21/ts-async";
import { scheduleTimeout } from "@nevware21/ts-utils";
const myPromise = createPromise((resolve, reject) => {
scheduleTimeout(() => {
resolve("foo");
}, 300);
});
myPromise
.then(handleFulfilledA, handleRejectedA)
.then(handleFulfilledB, handleRejectedB)
.then(handleFulfilledC, handleRejectedC)
.catch(handleRejectedAny);
// Create a new promise that resolves with an inner promise that will auto resolve after 1 second
createPromise((resolveOuter) => {
resolveOuter(
createPromise((resolveInner) => {
scheduleTimeout(resolveInner, 1000);
})
);
});
doAwait(myPromise, (result) => {
// Handle the result this would normally be
// let result = await myPromise;
}, (reason) => {
// Handle cases when the `myPromise` is rejected
// Using await you would handle this via try / catch
});
While the examples above are using the createPromise
you can directly use the createSyncPromise
, createAsyncPromise
, createIdlePromise
or createNativePromise
, you can also mix and match them (ie. they don't all have to be the same implementation). The createPromise
alias is provided to enable switching the underlying Promise implementation without changing any of your code.
General support is currently set to ES5 supported runtimes higher.
Internal polyfills are used to backfill ES5 functionality which is not provided by older browsers.
![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|
Latest ✔ | Latest ✔ | 9+ ✔ | Latest ✔ | Latest ✔ |
Note: A PolyFill
PolyPromise
provides the standard promise implementations for IE and ES5+ environments.
Read our contributing guide to learn about our development process, how to propose bugfixes and improvements, and how to build and test your changes.
FAQs
support for asynchronous development with a Promise based task Scheduler, several different Promise implementations (synchronous, idle, asynchronous and native runtime wrappers), await helpers, and aliases all built and tested using TypeScript.
The npm package @nevware21/ts-async receives a total of 0 weekly downloads. As such, @nevware21/ts-async popularity was classified as not popular.
We found that @nevware21/ts-async demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket is launching Ruby support for all users. Enhance your Rails projects with AI-powered security scans for vulnerabilities and supply chain threats. Now in Beta!
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.