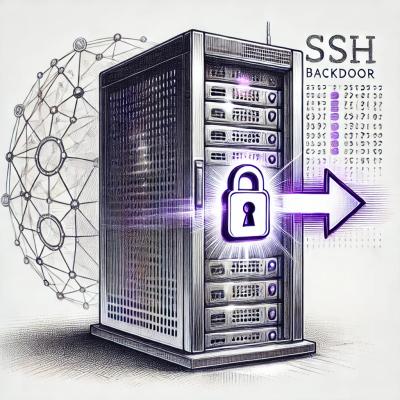
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@ng-select/ng-select
Advanced tools
@ng-select/ng-select is a highly customizable Angular component for creating select dropdowns. It provides a wide range of features including single and multiple selection, custom templates, filtering, and more.
Single Selection
This feature allows users to select a single item from a dropdown list. The `items` input takes an array of objects, and `bindLabel` specifies the property to display in the dropdown.
<ng-select [items]="cities" bindLabel="name" placeholder="Select a city"></ng-select>
Multiple Selection
This feature enables users to select multiple items from the dropdown. Setting the `multiple` input to `true` allows for multiple selections.
<ng-select [items]="cities" bindLabel="name" [multiple]="true" placeholder="Select cities"></ng-select>
Custom Templates
Custom templates allow for more control over the appearance of the dropdown items. The `ng-option-tmp` directive can be used to define a custom template for each item.
<ng-select [items]="cities" bindLabel="name" placeholder="Select a city">
<ng-template ng-option-tmp let-item="item" let-index="index">
<div class="custom-option">
<span>{{item.name}}</span>
</div>
</ng-template>
</ng-select>
Filtering
The filtering feature allows users to search through the dropdown items. Setting the `searchable` input to `true` enables this functionality.
<ng-select [items]="cities" bindLabel="name" [searchable]="true" placeholder="Select a city"></ng-select>
Async Data
This feature supports loading data asynchronously. The `items` input can take an observable, and the dropdown will update as the data is loaded.
<ng-select [items]="cities$ | async" bindLabel="name" placeholder="Select a city"></ng-select>
ngx-select-dropdown is another Angular package for creating dropdowns. It offers similar functionalities such as single and multiple selection, custom templates, and filtering. However, it may not be as feature-rich or customizable as @ng-select/ng-select.
ng-multiselect-dropdown is focused on providing a multi-select dropdown component for Angular. It supports features like filtering and custom templates but is primarily designed for multiple selections, unlike @ng-select/ng-select which supports both single and multiple selections.
angular-ng-autocomplete is an Angular component for creating autocomplete dropdowns. It provides features like filtering and custom templates, similar to @ng-select/ng-select, but is more focused on autocomplete functionality rather than general dropdowns.
Library is under active development and may not work as expected until stable 1.0.0 release.
After installing the above dependencies, install ng-select
via:
npm install --save @ng-select/ng-select
Once installed you need to import our main module:
import {NgSelectModule} from '@ng-select/ng-select';
The only remaining part is to list the imported module in your application module.:
import {NgSelectModule} from '@ng-select/ng-select';
@NgModule({
declarations: [AppComponent],
imports: [NgSelectModule],
bootstrap: [AppComponent]
})
export class AppModule {
}
You can also configure global configuration and localization messages by using NgSelectModule.forRoot:
NgSelectModule.forRoot({notFoundText: 'Your custom not found text', typeToSearchText: 'Your custom type to search text'})
If you are using SystemJS, you should also adjust your configuration to point to the UMD bundle.
In your systemjs config file, map
needs to tell the System loader where to look for ng-select
:
map: {
'@ng-select/ng-select': 'node_modules/@ng-select/ng-select/bundles/ng-select.umd.js',
}
Input | Type | Default | Required | Description |
---|---|---|---|---|
[items] | Array | [] | yes | Items array |
bindLabel | string | label | no | Object property to use for label. Default label |
bindValue | string | - | no | Object property to use for selected model. By default binds to whole object. |
[clearable] | boolean | true | no | Allow to clear selected value. Default true |
placeholder | string | - | no | Placeholder text. |
notFoundText | string | No items found | no | Set custom text when filter returns empty result |
typeToSearchText | string | Type to search | no | Set custom text when using Typeahead |
[typeahead] | Subject | - | no | Custom autocomplete or filter. |
Output | Description |
---|---|
(focus) | Fired on select focus |
(blur) | Fired on select blur |
(change) | Fired on selected value change |
(open) | Fired on select dropdown open |
(close) | Fired on select dropdown close |
This example in Plunkr
@Component({
selector: 'cities-page',
template: `
<label>City</label>
<ng-select [items]="cities"
bindLabel="name"
bindValue="id"
placeholder="Select city"
[(ngModel)]="selectedCityId">
</ng-select>
<p>
Selected city ID: {{selectedCityId}}
</p>
`
})
export class CitiesPageComponent {
cities = [
{id: 1, name: 'Vilnius'},
{id: 2, name: 'Kaunas'},
{id: 3, name: 'Pabradė'}
];
selectedCityId: any;
}
This example in Plunkr
In case of autocomplete you can get full control by creating simple EventEmmiter
and passing it as an input to ng-select. When you type text, ng-select will fire events to EventEmmiter to which you can subscribe and control bunch of things like debounce, http cancellation and so on.
@Component({
selector: 'select-autocomplete',
template: `
<label>Search with autocomplete in Github accounts</label>
<ng-select [items]="items"
bindLabel="login"
placeholder="Type to search"
[typeahead]="typeahead"
[(ngModel)]="githubAccount">
<ng-template ng-option-tmp let-item="item">
<img [src]="item.avatar_url" width="20px" height="20px"> {{item.login}}
</ng-template>
</ng-select>
<p>
Selected github account:
<span *ngIf="githubAccount">
<img [src]="githubAccount.avatar_url" width="20px" height="20px"> {{githubAccount.login}}
</span>
</p>
`
})
export class SelectAutocompleteComponent {
githubAccount: any;
items = [];
// event emmiter is just RxJs Subject
typeahead = new EventEmitter<string>();
constructor(private http: HttpClient) {
this.typeahead
.distinctUntilChanged()
.debounceTime(200)
.switchMap(term => this.loadGithubUsers(term))
.subscribe(items => {
this.items = items;
}, (err) => {
console.log(err);
this.items = [];
});
}
loadGithubUsers(term: string): Observable<any[]> {
return this.http.get<any>(`https://api.github.com/search/users?q=${term}`).map(rsp => rsp.items);
}
}
This example in Plunkr
To customize look of input display or option item you can use ng-template
with ng-label-tmp
or ng-option-tmp
directives applied to it.
import {Component, NgModule} from '@angular/core';
import {BrowserModule} from '@angular/platform-browser';
import {FormsModule} from '@angular/forms';
import {NgSelectModule} from '@ng-select/ng-select';
import {HttpClient, HttpClientModule} from '@angular/common/http';
@Component({
selector: 'select-custom-templates',
template: `
<label>Demo for ng-select with custom templates</label>
<ng-select [items]="albums"
[(ngModel)]="selectedAlbumId"
bindLabel="title"
bindValue="id"
placeholder="Select album">
<ng-template ng-label-tmp let-item="item">
<b>({{item.id}})</b> {{item.title}}
</ng-template>
<ng-template ng-option-tmp let-item="item">
<div>Title: {{item.title}}</div>
<small><b>Id:</b> {{item.id}} | <b>UserId:</b> {{item.userId}}</small>
</ng-template>
</ng-select>
<p>Selected album ID: {{selectedAlbumId || 'none'}}</p>
`
})
export class SelectCustomTemplatesComponent {
albums = [];
selectedAlbumId = null;
constructor(http: HttpClient) {
http.get<any[]>('https://jsonplaceholder.typicode.com/albums').subscribe(albums => {
this.albums = albums;
});
}
}
Visit https://github.com/ng-select/ng-select/tree/master/src/demo/app for more examples
Contributions are welcome. You can start by looking at issues with label Help wanted https://github.com/ng-select/ng-select/issues?q=is%3Aopen+is%3Aissue+label%3A%22help+wanted%22 or creating new Issue with proposal or bug report. Note that we are using https://conventionalcommits.org/ commits format.
Perform the clone-to-launch steps with these terminal commands.
git clone https://github.com/ng-select/ng-select
cd ng-select
npm install
npm run serve-demo
npm run test
or
npm run test-watch
./prerelease.sh
Script will pull from master, run command npm run release
and npm run build
.
After this you need to check if CHANGELOG is correct and run npm publish --access=public
from dist
folder.
npm run gh-pages
// after swith to gh-pages branch run
./publish-demo.sh
This component is inspired by https://github.com/JedWatson/react-select and https://github.com/rintoj/angular2-virtual-scroll. Check theirs amazing work and components :)
FAQs
Angular ng-select - All in One UI Select, Multiselect and Autocomplete
The npm package @ng-select/ng-select receives a total of 292,937 weekly downloads. As such, @ng-select/ng-select popularity was classified as popular.
We found that @ng-select/ng-select demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.