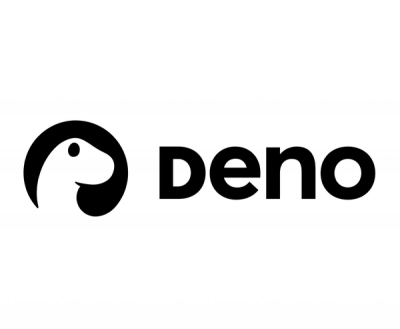
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@ngrx/component-store
Advanced tools
@ngrx/component-store is a library for managing local/component state in Angular applications. It provides a simple and reactive way to manage state within Angular components, leveraging RxJS for state management and side effects.
State Management
This feature allows you to manage the state within a component. The `ComponentStore` class is used to define the state and provide methods to update it.
```typescript
import { ComponentStore } from '@ngrx/component-store';
interface State {
count: number;
}
@Injectable()
class CounterStore extends ComponentStore<State> {
constructor() {
super({ count: 0 });
}
readonly increment = this.updater((state) => ({ count: state.count + 1 }));
readonly decrement = this.updater((state) => ({ count: state.count - 1 }));
readonly reset = this.updater(() => ({ count: 0 }));
}
```
Selectors
Selectors are used to derive and expose slices of the state. The `select` method allows you to create observables that emit state changes.
```typescript
@Injectable()
class CounterStore extends ComponentStore<State> {
constructor() {
super({ count: 0 });
}
readonly count$ = this.select(state => state.count);
}
```
Effects
Effects are used to handle side effects, such as HTTP requests. The `effect` method allows you to define observables that can trigger state changes based on external events.
```typescript
@Injectable()
class CounterStore extends ComponentStore<State> {
constructor(private readonly http: HttpClient) {
super({ count: 0 });
}
readonly loadCount = this.effect((trigger$) =>
trigger$.pipe(
switchMap(() => this.http.get<number>('/api/count').pipe(
tapResponse(
(count) => this.patchState({ count }),
(error) => console.error(error)
)
))
)
);
}
```
NGXS is a state management library for Angular that is modeled after the Redux pattern. It provides a more opinionated and structured approach to state management compared to @ngrx/component-store, with features like actions, selectors, and middleware.
Akita is a state management library for Angular applications that focuses on simplicity and performance. It provides a more flexible and less opinionated approach compared to @ngrx/component-store, with features like entity stores and query services.
Angular-Redux is a library that integrates Redux with Angular. It provides a way to manage global state using the Redux pattern, which can be more complex and boilerplate-heavy compared to the local state management provided by @ngrx/component-store.
The sources for this package are in the main NgRx repo. Please file issues and pull requests against that repo.
16.0.0-beta.0 (2023-04-27)
any
type with unknown
type (#3827) (0ea2933)createActionGroup
function.BEFORE:
All letters of the event name will be lowercase, except for the initial letters of words starting from the second word, which will be uppercase.
const authApiActions = createActionGroup({
source: 'Auth API',
events: {
'LogIn Success': emptyProps(),
'login failure': emptyProps(),
'Logout Success': emptyProps(),
logoutFailure: emptyProps(),
},
});
// generated actions:
const { loginSuccess, loginFailure, logoutSuccess, logoutfailure } = authApiActions;
AFTER:
The initial letter of the first word of the event name will be lowercase, and the initial letters of the other words will be uppercase. The case of other letters in the event name will remain the same.
const { logInSuccess, loginFailure, logoutSuccess, logoutFailure } = authApiActions;
createFeature
signature with root state is removed in favor of a signature without root state.
An automatic migration is added to remove this signature.BEFORE:
interface AppState {
users: State;
}
export const usersFeature = createFeature<AppState>({
name: 'users',
reducer: createReducer(initialState /* case reducers */),
});
AFTER:
export const usersFeature = createFeature({
name: 'users',
reducer: createReducer(initialState /* case reducers */),
});
getSelectors
function has been removed from the @ngrx/router-store
package.BEFORE:
The @ngrx/router-store package exports the getSelectors
function.
AFTER:
The @ngrx/router-store package no longer exports the getSelectors
function. A migration has been provided to replace existing usage
any
types to define actions, these are replaced with the unknown
type.BEFORE:
Schematics used the any
type to declare action payload type.
AFTER:
Schematics use the unknown
type to declare action payload type.
getMockStore
function is removed in favor of createMockStore
BEFORE:
import { getMockStore } from '@ngrx/store/testing';
const mockStore = getMockStore();
AFTER:
import { createMockStore } from '@ngrx/store/testing';
const mockStore = createMockStore();
BEFORE:
Projector function arguments of selectors generated by createFeature are not strongly typed:
const counterFeature = createFeature({
name: 'counter',
reducer: createReducer({ count: 0 }),
});
counterFeature.selectCount.projector;
// type: (...args: any[]) => number
AFTER:
Projector function arguments of selectors generated by createFeature are strongly typed:
const counterFeature = createFeature({
name: 'counter',
reducer: createReducer({ count: 0 }),
});
counterFeature.selectCount.projector;
// type: (featureState: { count: number; }) => number
<a name="15.4.0"></a>
FAQs
Reactive store for component state
The npm package @ngrx/component-store receives a total of 200,592 weekly downloads. As such, @ngrx/component-store popularity was classified as popular.
We found that @ngrx/component-store demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.