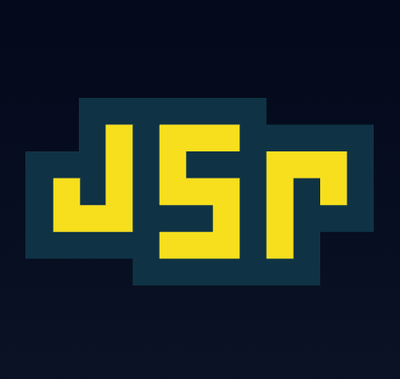
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@ngrx/store
Advanced tools
@ngrx/store is a state management library for Angular applications, inspired by Redux. It provides a way to manage state in a predictable manner using actions, reducers, and selectors.
State Management
This code demonstrates how to set up a basic state management system using @ngrx/store. The StoreModule is imported and configured with a reducer.
import { StoreModule } from '@ngrx/store';
import { counterReducer } from './counter.reducer';
@NgModule({
imports: [
StoreModule.forRoot({ count: counterReducer })
]
})
export class AppModule {}
Actions
This code shows how to create actions using the createAction function. Actions are dispatched to trigger state changes.
import { createAction } from '@ngrx/store';
export const increment = createAction('[Counter] Increment');
export const decrement = createAction('[Counter] Decrement');
export const reset = createAction('[Counter] Reset');
Reducers
This code defines a reducer function using createReducer and on functions. The reducer handles different actions to update the state.
import { createReducer, on } from '@ngrx/store';
import { increment, decrement, reset } from './counter.actions';
export const initialState = 0;
const _counterReducer = createReducer(initialState,
on(increment, state => state + 1),
on(decrement, state => state - 1),
on(reset, state => initialState)
);
export function counterReducer(state, action) {
return _counterReducer(state, action);
}
Selectors
This code demonstrates how to create selectors to read specific pieces of state. Selectors are used to derive data from the store.
import { createSelector, createFeatureSelector } from '@ngrx/store';
export const selectCounterState = createFeatureSelector<number>('count');
export const selectCount = createSelector(
selectCounterState,
(state: number) => state
);
Redux is a popular state management library for JavaScript applications. It is similar to @ngrx/store in that it uses actions, reducers, and a single store to manage state. However, Redux is framework-agnostic and can be used with any JavaScript framework or library.
MobX is a state management library that uses observables to manage state. Unlike @ngrx/store, which follows a more rigid, predictable state management pattern, MobX allows for more flexible and reactive state management.
Vuex is a state management library specifically designed for Vue.js applications. It is similar to @ngrx/store in that it uses a centralized store, actions, and mutations (similar to reducers) to manage state. However, it is tightly integrated with Vue.js.
RxJS powered state management inspired by Redux for Angular2 apps
http://plnkr.co/edit/Hb4pJP3jGtOp6b7JubzS?p=preview
npm install angular2
npm install @ngrx/store
//counter.ts
import {Reducer, Action} from '@ngrx/store';
export const INCREMENT = 'INCREMENT';
export const DECREMENT = 'DECREMENT';
export const RESET = 'RESET';
export const counter:Reducer<number> = (state:number = 0, action:Action) => {
switch (action.type) {
case INCREMENT:
return state + 1;
case DECREMENT:
return state - 1;
case RESET:
return 0;
default:
return state;
}
}
provideStore(reducers, initialState)
function to provide them to Angular's injector:
import {bootstrap} from 'angular2/platform/bootstrap';
import {provideStore} from '@ngrx/store';
import {App} from './myapp';
import {counter} from './counter';
bootstrap(App, [ provideStore({counter}, {counter: 0}) ]);
Store
service into your Components and Services:import {Store} from '@ngrx/store';
import {INCREMENT, DECREMENT, RESET} from './counter';
@Component({
selector: 'my-app',
template: `
<button (click)="increment()">Increment</button>
<div>Current Count: {{ counter | async }}</div>
<button (click)="decrement()">Decrement</button>
`
})
class MyApp {
counter: Observable<number>;
constructor(public store: Store<number>){
this.counter = store.select('counter');
}
increment(){
this.store.dispatch({ type: INCREMENT });
}
decrement(){
this.store.dispatch({ type: DECREMENT });
}
reset(){
this.store.dispatch({ type: RESET });
}
}
###Middleware
####(observable: Observable<any>): Observable<any>
Store middleware provides pre and post reducer entry points for all dispatched actions. Middleware can be used for everything from logging, asynchronous event handling, to action or post action manipulation.
#####Dispatched action pipeline:
(observable: Observable<Action>): Observable<Action>
(observable: Observable<State>): Observable<State>
Middleware can be configured during application bootstrap by utilizing the usePreMiddleware(...middleware: Middleware[])
and usePostMiddleware(...middleware: Middleware[])
helper functions.
import {bootstrap} from 'angular2/platform/browser';
import {App} from './myapp';
import {provideStore, usePreMiddleware, usePostMiddleware, Middleware} from "@ngrx/store";
import {counter} from "./counter";
const actionLog : Middleware = action => {
return action.do(val => {
console.warn('DISPATCHED ACTION: ', val)
});
};
const stateLog : Middleware = state => {
return state.do(val => {
console.info('NEW STATE: ', val)
});
};
bootstrap(App, [
provideStore({counter},{counter : 0}),
usePreMiddleware(actionLog),
usePostMiddleware(stateLog)
]);
For a more complex example check out store-saga, an ngrx store middleware implementation inspired by redux saga.
Please read contributing guidelines here.
FAQs
RxJS powered Redux for Angular apps
The npm package @ngrx/store receives a total of 545,457 weekly downloads. As such, @ngrx/store popularity was classified as popular.
We found that @ngrx/store demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.