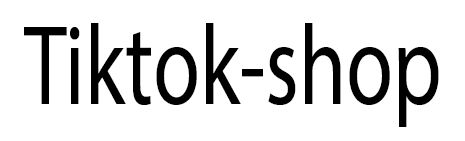
Generate "signature" and "token" for Tiktok Shop.

I am very happy and grateful for everyone's help. These meaningful contributions will greatly help me in expanding the useful library to help people.
Content
Installation
Installation is done using the
npm install
command:
$ npm install tiktok-shop-client
Back
Features
- Generate Signature
- Generate Token using Auth Code
- Generate Token using Refresh Token
Back
Generate Signature using Url
const tiktokShop = require('tiktok-shop')
const url = 'https://open-api.tiktokglobalshop.com/order/202309/orders?access_token=ROW_CBxxx&app_key=6a6xxx&ids=5779xxx&shop_cipher=ROW_Y-vWxxx&shop_id=×tamp=1697708762&version=202309';
const appSecret = '4ebxxx';
const signature = tiktokShop.signByUrl(url, appSecret);
console.info(signature);
Response Data
{
signature: '96f15922fbacd220cea0d8370ba7dff2273674f2a2856868b7e32f7d98da0efe',
timestamp: 1697540200
}
Back
Generate Signature using Config
const tiktokShop = require('tiktok-shop-client')
const config = {
app_key: 'yourAppKey',
app_secret: 'yourAppSecret',
shop_id: 'yourShopId',
shop_cipher: 'yourShopCipher',
version: '202306',
}
const path = '/api/orders/search';
const signature = tiktokShop.signature(config, path);
console.info(signature);
Response Data
{
signature: '96f15922fbacd220cea0d8370ba7dff2273674f2a2856868b7e32f7d98da0efe',
timestamp: 1697540200
}
Back
Generate Token using Auth Code
const tiktokShop = require('tiktok-shop-client')
const config = {
app_key: 'yourAppKey',
app_secret: 'yourAppSecret',
}
const authCode = 'yourAuthCode';
const accessToken = tiktokShop.authCodeToken(config, authCode);
console.info(accessToken);
Response Data
{
"access_token": "ROW_-3_uKAAAAADYdCab***",
"access_token_expire_in": 1696992654,
"refresh_token": "ROW_RBHCjwAAAACgH1O***",
"refresh_token_expire_in": 4818450857,
"open_id": "D3MazQAAAAAi5AmxAvxkSaBRs***",
"seller_name": "Test",
"seller_base_region": "VN",
"user_type": 0
}
Back
Generate Token using Refresh Token
const tiktokShop = require('tiktok-shop-client')
const config = {
app_key: 'yourAppKey',
app_secret: 'yourAppSecret',
}
const refreshToken = 'yourRefreshToken';
const accessToken = tiktokShop.authCodeToken(config, refreshToken);
console.info(accessToken);
Response Data
{
"access_token": "ROW_-3_uKAAAAADYdCab***",
"access_token_expire_in": 1696992654,
"refresh_token": "ROW_RBHCjwAAAACgH1O***",
"refresh_token_expire_in": 4818450857,
"open_id": "D3MazQAAAAAi5AmxAvxkSaBRs***",
"seller_name": "Test",
"seller_base_region": "VN",
"user_type": 0
}
Back
Using TikTok Shop Client API
The TikTok Shop Client API can be easily used to interact with the TikTok API. Here is an example of how it can be used:
const tiktokShop = require('tiktok-shop-client');
const client = tiktokShop.TikTokClient(
{
appKey:'yourAppKey',
appSecret:'yourAppSecret',
accessToken:'yourAccessToken',
shopCipher:'yourShopCipher',
shopId:'yourShopId'
});
client.getProduct('productId').then(product => {
console.log(product);
});
In this example, we are creating a new TikTok client and then we use the getProduct method to get product details.
Back