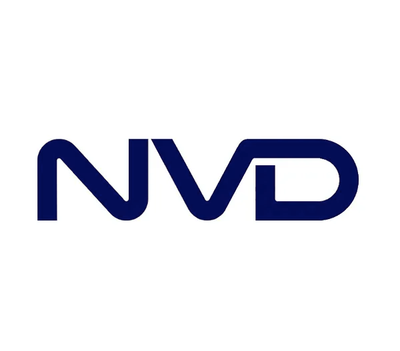
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
@node-rs/argon2
Advanced tools
@node-rs/argon2 is a Node.js binding for the Argon2 password hashing algorithm, which is designed to be memory-hard and CPU-intensive to resist brute-force attacks. This package provides a fast and efficient way to hash and verify passwords using Argon2.
Hashing a password
This feature allows you to hash a password using the Argon2 algorithm. The code sample demonstrates how to hash a password asynchronously and log the resulting hash.
const argon2 = require('@node-rs/argon2');
(async () => {
const hash = await argon2.hash('password123');
console.log(hash);
})();
Verifying a password
This feature allows you to verify a password against a previously generated hash. The code sample demonstrates how to hash a password and then verify it, logging the result of the verification.
const argon2 = require('@node-rs/argon2');
(async () => {
const hash = await argon2.hash('password123');
const isValid = await argon2.verify(hash, 'password123');
console.log(isValid); // true
})();
The 'argon2' package is another Node.js binding for the Argon2 password hashing algorithm. It provides similar functionality to @node-rs/argon2, including hashing and verifying passwords. However, @node-rs/argon2 is known for its performance improvements and efficiency due to its Rust-based implementation.
The 'bcrypt' package is a popular alternative for password hashing in Node.js. It uses the bcrypt algorithm, which is also designed to be computationally expensive to resist brute-force attacks. While bcrypt is widely used and trusted, Argon2 (used by @node-rs/argon2) is considered to be more secure due to its memory-hard properties.
The 'scrypt' package provides bindings for the scrypt key derivation function, which is another algorithm designed to be memory-hard and resistant to brute-force attacks. Similar to @node-rs/argon2, scrypt is used for secure password hashing, but Argon2 is generally preferred for its modern design and security features.
@node-rs/argon2
RustCrypto: Argon2 binding for Node.js.
Argon2 is a key derivation function that was selected as the winner of the Password Hashing Competition(PHC) in July 2015.
Argon2 summarizes the state of the art in the design of memory-hard functions and can be used to hash passwords for credential storage, key derivation, or other applications.
It has a simple design aimed at the highest memory filling rate and effective use of multiple computing units, while still providing defense against tradeoff attacks (by exploiting the cache and memory organization of the recent processors).
Faster performance.
No node-gyp and postinstall.
Cross-platform support, including Apple M1.
Smaller file size after npm installation(476K vs node-argon2 3.7M).
@node-rs/argon2
supports all three algorithms:
node12 | node14 | node16 | node17 | |
---|---|---|---|---|
Windows x64 | ✓ | ✓ | ✓ | ✓ |
Windows x32 | ✓ | ✓ | ✓ | ✓ |
Windows arm64 | ✓ | ✓ | ✓ | ✓ |
macOS x64 | ✓ | ✓ | ✓ | ✓ |
macOS arm64(m chip) | ✓ | ✓ | ✓ | ✓ |
Linux x64 gnu | ✓ | ✓ | ✓ | ✓ |
Linux x64 musl | ✓ | ✓ | ✓ | ✓ |
Linux arm gnu | ✓ | ✓ | ✓ | ✓ |
Linux arm64 gnu | ✓ | ✓ | ✓ | ✓ |
Linux arm64 musl | ✓ | ✓ | ✓ | ✓ |
Android arm64 | ✓ | ✓ | ✓ | ✓ |
Android armv7 | ✓ | ✓ | ✓ | ✓ |
FreeBSD x64 | ✓ | ✓ | ✓ | ✓ |
See benchmark/.
export const enum Algorithm {
Argon2d = 0,
Argon2i = 1,
Argon2id = 2,
}
export const enum Version {
/** Version 16 (0x10 in hex) */
V0x10 = 0,
/**
* Default value
* Version 19 (0x13 in hex, default)
*/
V0x13 = 1,
}
export interface Options {
/**
* The amount of memory to be used by the hash function, in kilobytes. Each thread will have a memory pool of this size. Note that large values for highly concurrent usage will cause starvation and thrashing if your system memory gets full.
*
* Value is an integer in decimal (1 to 10 digits), between 1 and (2^32)-1.
*
* The default value is 4096, meaning a pool of 4 MiB per thread.
*/
memoryCost?: number | undefined | null
/**
* The time cost is the amount of passes (iterations) used by the hash function. It increases hash strength at the cost of time required to compute.
*
* Value is an integer in decimal (1 to 10 digits), between 1 and (2^32)-1.
*
* The default value is 3.
*/
timeCost?: number | undefined | null
/**
* The hash length is the length of the hash function output in bytes. Note that the resulting hash is encoded with Base 64, so the digest will be ~1/3 longer.
*
* The default value is 32, which produces raw hashes of 32 bytes or digests of 43 characters.
*/
outputLen?: number | undefined | null
/**
* The amount of threads to compute the hash on. Each thread has a memory pool with memoryCost size. Note that changing it also changes the resulting hash.
*
* Value is an integer in decimal (1 to 3 digits), between 1 and 255.
*
* The default value is 1, meaning a single thread is used.
*/
parallelism?: number | undefined | null
algorithm?: Algorithm | undefined | null
version?: Version | undefined | null
secret?: Buffer | undefined | null
}
export function hash(
password: string | Buffer,
options?: Options | undefined | null,
abortSignal?: AbortSignal | undefined | null,
): Promise<string>
export function verify(
hashed: string | Buffer,
password: string | Buffer,
options?: Options | undefined | null,
abortSignal?: AbortSignal | undefined | null,
): Promise<boolean>
FAQs
RustCrypto: Argon2 binding for Node.js
The npm package @node-rs/argon2 receives a total of 80,492 weekly downloads. As such, @node-rs/argon2 popularity was classified as popular.
We found that @node-rs/argon2 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.