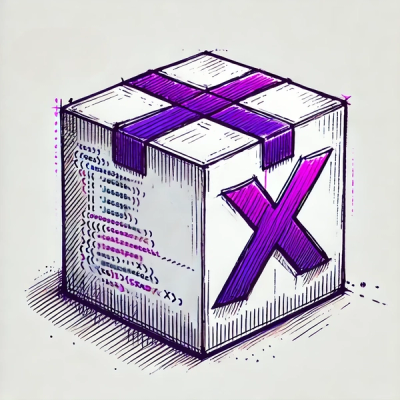
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@notabene/javascript-sdk
Advanced tools
This library is the JavaScript SDK for loading the widget on a frontend.
There are two options for loading the Notabene SDK:
<script id="notabene" async src="https://unpkg.com/@notabene/javascript-sdk@1.31.0/dist/es/index.js"></script>
Or installing the library:
yarn add @notabene/javascript-sdk
Use the customer token endpoint with your access token to receive a token with a customer's scope.
⚠️ IMPORTANT ⚠️
When requesting the
customer token
you must pass a uniquecustomerRef
per customer for ownership proof reusability, otherwise you might encounter unwanted behavior.
Create a new Notabene instance:
const notabene = new Notabene({
vaspDID: 'did:ethr:0x94c38fd29ef36f5cb2f7bc771f9d5bd9f7d05f27',
widget: 'https://beta-widget.notabene.id',
container: '#container',
authToken: '{CUSTOMER_TOKEN}',
onValidStateChange: (isValid) => {
// Use this value to determine if the transaction is ready to be created.
console.log('is transaction valid', isValid);
},
onError: (err) => {
// If any errors are encountered, they will be passed to this function
alert(err.message);
},
});
Then render the widget:
// Use this method when you need to collect missing information from the sender about the recipient (normal withdrawal)
notabene.renderWidget('WITHDRAWAL');
// Use this method when you need to collect missing information from the recipient about the sender (for transactions created via notification)
notabene.renderWidget('POST_DEPOSIT');
To update the widget as users enter transaction details:
notabene.setTransaction({
transactionAsset: 'ETH',
beneficiaryAccountNumber: '0x8d12a197cb00d4747a1fe03395095ce2a5cc6819',
transactionAmount: '2000000000',
});
Once the widget determines the transaction is valid, it will call the onValidStateChange
callback, to access the transaction details:
const currentTransactionInfo = notabene.tx;
USDC
the simple asset code passed as a string
, in case different chain (polygon) -> USDC-POLY
CAIP19
format
{
caip19: 'eip155:1/erc20:0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48'; // for USDC
}
coingeckoId
and network
format
{
coingeckoId: "usd-coin",
network: "ethereum"
}
WARNING: this shouldn't be used often, if you need to change the transaction's initial fields call setTransaction
again. (This should be used only for tearing down the component).
The container of the widget must be in the DOM before calling destroyWidget
or renderWidget
.
If you need to close and re-render the widget, call the destroyWidget
method like so:
// Will remove the widget
notabene.destroyWidget();
// Will re-render the widget
notabene.renderWidget();
Calling the renderWidget
methods without destroying it first will not work.
If any error occurs, the onError
function from the Notabene instance will be triggered. The error
passed as argument contains a data
property that has the following type ErrorData
.
⚠️ Note: These errors are mainly used for debugging internal issues while implementing the widget. They are not meant to be shown directly to the end user.
type ErrorData = {
title: string;
detail: string;
code: number;
type: string;
};
const notabene = new Notabene({
...,
onError: (err) => {
const errorData = err.data;
// Do something
},
});
Notabene Internal Errors
Type | Code | Title | Detail |
---|---|---|---|
BAD_REQUEST | 400 | Bad Request | NotabeneBadRequest: ... |
TRANSACTION_INVALID | 400 | Transaction Invalid | NotabeneTransactionInvalid: ... |
TOKEN_INVALID | 401 | Token Invalid | NotabeneTokenInvalid: ... |
ASSET_NOT_SUPPORTED | 404 | Asset Not Supported | NotabeneAssetNotSupported: ... |
SERVICE_UNAVAILABLE | 500 | Service Unavailable | NotabeneServiceUnavailable: ... |
WALLET_CONNECTED_FAILED | 500 | Wallet Connection Failed | NotabeneWalletConnectionFailed: ... |
WALLET_NOT_SUPPORTED | 501 | Wallet Not Supported | NotabeneWalletNotSupported: ... |
transactionTypeAllowed
: ALL
| VASP_2_VASP_ONLY
| SELF_TRANSACTION_ONLY
For limiting the type of transaction destinations you can pass.
ALL
: All transaction destinations are allowed. (DEFAULT)
VASP_2_VASP_ONLY
: Only transaction destinations that are VASPs are allowed.
SELF_TRANSACTION_ONLY
: Only transaction destinations that the originator owns are allowed.
nonCustodialDeclarationType
: SIGNATURE
| DECLARATION
For deciding which ownership proof type you want to use.
SIGNATURE
: The ownership proof will be signed by the originator using a wallet. (DEFAULT)
DECLARATION
: The ownership proof will be declared by the originator using a checkbox.
const notabene = new Notabene({
vaspDID: 'did:ethr:0x94c38fd29ef36f5cb2f7bc771f9d5bd9f7d05f27',
widget: 'https://beta-widget.notabene.id',
container: '#container',
authToken: '{CUSTOMER_TOKEN}'
allowedTransactionTypes: 'VASP_2_VASP_ONLY',
nonCustodialDeclarationType: 'DECLARATION',
});
Pass a theme
object when creating an instance
Here you can pass configuration which will customize the styles of the widget to meet your brand needs, all values are optional.
{
primaryColor: '#fff',
secondaryColor: '#fff',
primaryFontColor: '#fff',
secondaryFontColor: '#fff',
backgroundColor: '#fff',
fontFamily: Arial,
logo: {LOGO_URL},
mode: 'dark', // default: 'light'
// If you are using the Signature flow, here is the full list of custom theme that can be applied to the WalletConnect Modal.
'--w3m-font-family',
'--w3m-font-feature-settings',
'--w3m-overlay-background-color',
'--w3m-overlay-backdrop-filter',
'--w3m-z-index',
'--w3m-accent-color',
'--w3m-accent-fill-color',
'--w3m-background-color',
'--w3m-background-image-url',
'--w3m-logo-image-url',
'--w3m-background-border-radius',
'--w3m-container-border-radius',
'--w3m-wallet-icon-border-radius',
'--w3m-wallet-icon-large-border-radius',
'--w3m-wallet-icon-small-border-radius',
'--w3m-input-border-radius',
'--w3m-notification-border-radius',
'--w3m-button-border-radius',
'--w3m-secondary-button-border-radius',
'--w3m-icon-button-border-radius',
'--w3m-button-hover-highlight-border-radius',
}
To get a list of supported fonts, visit https://fonts.google.com/
To get more details about the WalletConnect theme variables, visit General style variables.
Pass a dictionary
object mapping in text in the widget to your own language, for example:
To replace Loading...
with Cargando...
{
'Loading...': 'Cargando...',
}
Possible fields customization support:
field name | forceDisplay | optional | description |
---|---|---|---|
counterparty | -- | ☑️ | Counterparty VASP or Wallet (destination or originator) |
dateAndPlaceOfBirth | ☑️ | -- | Recipient (or Sender) Date and Place of Birth |
geographicAddress | ☑️ | ☑️ | Recipient (or Sender) Address |
firstName | ☑️ | -- | Recipient (or Sender) First name |
name | ☑️ | -- | Recipient (or Sender) Last Name / Company Name |
nationalIdentification | ☑️ | --️ | Recipient (or Sender) National Identification |
country | ☑️ | ☑️ | Recipient (or Sender) Country of Residence (for natural person) / Country of Registration (for legal person) |
Field Options
{
counterparty: {
optional: true;
},
geographicAddress: {
forceDisplay: true,
optional: true;
},
}
Beneficiary Details [DEPRECATED]
You can require specific beneficiary fields that are not required by your jurisdiction.
Possible fields:
beneficiaryName
- Recipient NamebeneficiaryGeographicAddress
- Recipient AddressbeneficiaryNationalIdentification
- Recipient National IdentificationbeneficiaryDateAndPlaceOfBirth
- Recipient Date and Place of BirthbeneficiaryDetails: [
'benficiaryName',
'beneficiaryGeographicAddress',
'beneficiaryNationalIdentification',
'beneficiaryDateAndPlaceOfBirth',
];
Fallbacks are custom options provided when desired outcome is not achieved
Possible options and actions:
WALLET_NOT_SUPPORTED
- Performs one of the following options when wallet is not supported
DECLARATION
- Falls back to the self declaration flowREJECT
- Rejects the transaction and throws an error
{
"type": "WALLET_NOT_SUPPORTED",
"title": "Wallet Not Supported",
"status": 501,
"detail": "We don't support the wallet for the provided asset"
}
fallbacks: [{flow: "WALLET_NOT_SUPPORTED", action: "DECLARATION"}]
Finally you pass the configuration to a Notabene instance:
const notabene = new Notabene({
widget: 'https://beta-widget.notabene.id',
container: '#container',
authToken: '{CUSTOMER_TOKEN}'
theme: {THEME},
dictionary: {DICTIONARY},
fallbacks: [{flow: "WALLET_NOT_SUPPORTED", action: "DECLARATION"}]
});
const notabene = new Notabene({
widget: 'https://beta-widget.notabene.id',
container: '#container',
authToken: '{CUSTOMER_TOKEN}'
theme: {THEME},
dictionary: {DICTIONARY},
optInFeatures: ['REUSE_ADDRESS_OWNERSHIP_PROOF']
});
Available Opt-In Features
REUSE_ADDRESS_OWNERSHIP_PROOF
: For a given unique customer (identified by the customerRef
from the customer token used) + unique wallet address, the widget would not ask for data collection if the customer previously verified the address ownership in a previous first party self-hosted transfer.To use your own asset price as fallback in case an asset is not supported by Notabene, you can provide a customAssetPrice
object that will be used instead to render the widget with the correct jurisdiction requirements.
notabene.setTransaction({
transactionAsset: 'ASSET_UNSUPPORTED_FROM_NOTABENE',
beneficiaryAccountNumber: '0x8d12a197cb00d4747a1fe03395095ce2a5cc6819',
transactionAmount: '2000000000',
customAssetPrice: {
priceUSD: 1700.12, // Asset price in USD
decimals: 10 // Decimals used for the given transactionAmount
};
});
BSD 3-Clause © Notabene Inc.
FAQs
JavaScript SDK for Notabene
The npm package @notabene/javascript-sdk receives a total of 1,677 weekly downloads. As such, @notabene/javascript-sdk popularity was classified as popular.
We found that @notabene/javascript-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.