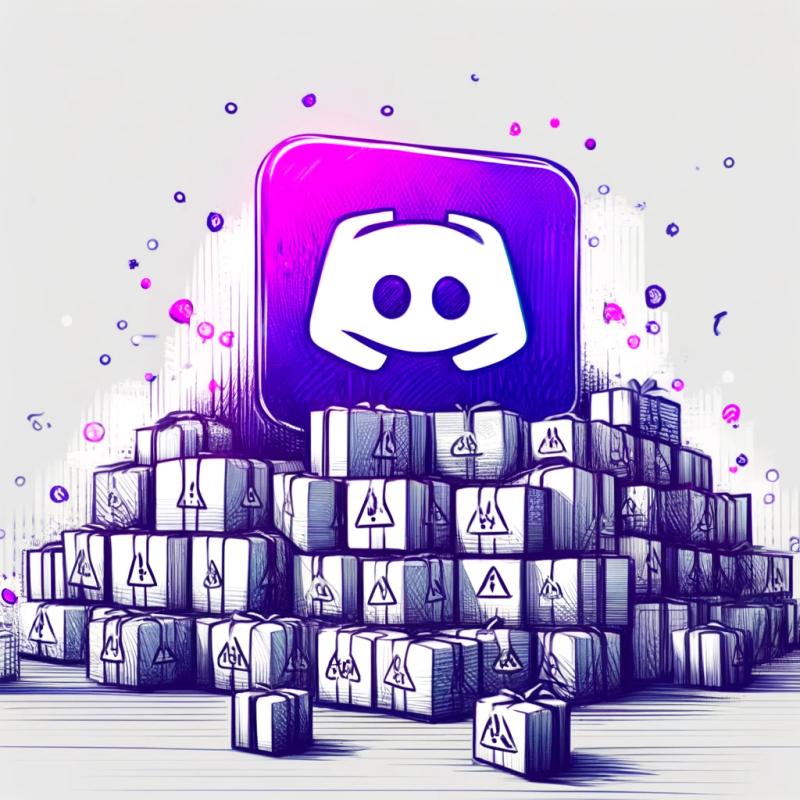
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
@npmcli/ci-detect
Advanced tools
Readme
Detect what kind of CI environment the program is in
const ciDetect = require('@npmcli/ci-detect')
// false if not in CI
// otherwise, a string indicating the CI environment type
const inCI = ciDetect()
Returns one of the following strings, or false
if none match, by looking
at the appropriate environment variables.
'gerrit'
Gerrit'gitlab'
GitLab'circleci'
Circle-CI'semaphore'
Semaphore'drone'
Drone'github-actions'
GitHub Actions'tddium'
TDDium'jenkins'
Jenkins'bamboo'
Bamboo'gocd'
GoCD'codeship'
CodeShip (or any that set CI_NAME
environment variable)'travis-ci'
Travis-CI - A few other CI systems set TRAVIS=1
in the
environment, because devs use that to indicate "test mode", so this one
can get some false positives.'aws-codebuild'
AWS CodeBuild'builder'
Google Cloud Builder - This one is a bit weird. It doesn't
really set anything that can be reliably detected except
BUILDER_OUTPUT
, so it can get false positives pretty easily.'custom'
anything else that sets CI
environment variableNote that since any program can set or unset whatever environment variables they want, this is not 100% reliable.
Also, note that if your program does different behavior in CI/test/deployment than other places, then there's a good chance that you're doing something wrong!
But, for little niceties like setting colors or other output parameters, or logging and that sort of non-essential thing, this module provides a way to tweak without checking a bunch of things in a bunch of places. Mostly, it's a single place to keep a note of what CI system sets which environment variable.
FAQs
Detect what kind of CI environment the program is in
We found that @npmcli/ci-detect demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.