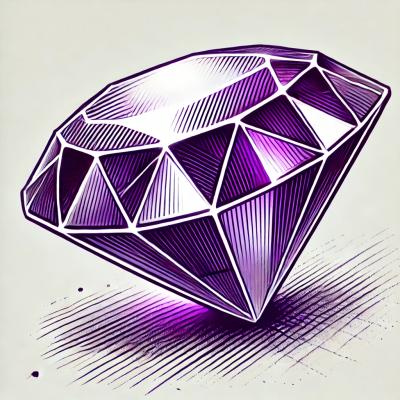
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@nutrify/quill-emoji-mart-picker
Advanced tools
Module and Blot for Quill.js that supports Emoji Mart Picker and Emoji Mart.
With this plugin emojis within your Quill Editor become consistent among all devices and browsers.
This extension converts unicode emojis, emoticons and emoji names into emoji images from Apple, Google, Twitter, EmojiOne, Facebook Messenger or Facebook.
Custom emojis in the form of "Emoji Mart emojis" are also supported.
In comparison to other modules it also features copy & pasting and replacement as you type of emojis, custom emojis and emoticons from within and outside the editor.
The emoji's image URL is the same as Emoji Mart's to not send unnecessary requests.
Although the plugin should be used with Emoji Mart Picker, it can also be used with Emoji Mart or as a standalone without the picker.
You can not use a suitable picker (Emoji Mart Picker / Emoji Mart) if you are not using Angular, React or Vue which is required for Emoji Mart.
This package requires Quill
npm install quill @nutrify/quill-emoji-mart-picker --save
For styling import @nutrify/quill-emoji-mart-picker/emoji.quill.css
On Angular you should install Emoji Mart Picker instead of Emoji Mart to be able to use the replacement of emoji short names at the same time as emoticons.
Emoji Mart Picker also has a few bug fixes.
npm install quill @nutrify/quill-emoji-mart-picker @nutrify/ngx-emoji-mart-picker --save
Additionally install a Quill wrapper (ngx-quill or ngx-quill-wrapper) for Angular:
npm install ngx-quill --save
Additionally install Emoji Mart according to your platform.
Any Quill wrapper should work.
this.set = 'apple';
// Optional custom emojis
this.customEmojis = [
{
name: 'Party Parrot',
shortNames: ['parrot'],
keywords: ['party'],
imageUrl: './assets/images/parrot.gif',
},
{
name: 'Test Flag',
shortNames: ['test'],
keywords: ['test', 'flag'],
spriteUrl: 'https://unpkg.com/emoji-datasource-twitter@4.0.4/img/twitter/sheets-256/64.png',
sheet_x: 1,
sheet_y: 1,
size: 64,
sheetColumns: 52,
sheetRows: 52,
},
];
const quill = new Quill(editor, {
// ...
modules: {
// ...
'emoji-module': {
emojiData: emojis,
customEmojiData: this.customEmojis,
preventDrag: true,
showTitle: true,
indicator: '*',
convertEmoticons: true,
convertShortNames: true,
set: () => this.set
},
toolbar: false
},
formats: ['emoji'] // Use formats with toolbar: false, if you want to allow text and emojis only
});
This example is using ngx-quill, other wrappers also work.
app.module.ts:
import '@nutrify/quill-emoji-mart-picker';
import { QuillModule } from 'ngx-quill';
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { PickerModule } from '@nutrify/ngx-emoji-mart-picker';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
QuillModule.forRoot(),
PickerModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
component.ts:
import { Component, VERSION } from '@angular/core';
import { emojis } from '@nutrify/ngx-emoji-mart-picker/ngx-emoji/esm5/data/emojis';
import { EmojiEvent } from '@nutrify/ngx-emoji-mart-picker/ngx-emoji/public_api';
import { Emoji } from '@nutrify/quill-emoji-mart-picker';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
set = 'apple';
modules = {};
formats: string[] = [];
quill = null;
customEmojis = [
{
name: 'Party Parrot',
shortNames: ['parrot'],
keywords: ['party'],
imageUrl: './assets/images/parrot.gif',
},
{
name: 'Test Flag',
shortNames: ['test'],
keywords: ['test', 'flag'],
spriteUrl: 'https://unpkg.com/emoji-datasource-twitter@4.0.4/img/twitter/sheets-256/64.png',
sheet_x: 1,
sheet_y: 1,
size: 64,
sheetColumns: 52,
sheetRows: 52,
},
];
created(quill: any) {
this.quill = quill;
}
insertEmoji(event: EmojiEvent) {
Emoji.insertEmoji(this.quill, event);
}
constructor() {
this.modules = {
'emoji-module': {
emojiData: emojis,
customEmojiData: this.customEmojis,
preventDrag: true,
showTitle: true,
indicator: '*',
convertEmoticons: true,
convertShortNames: true,
set: () => this.set
},
toolbar: false
};
this.formats = ['emoji'];
}
}
component.html:
<!-- ... -->
<div>
<quill-editor [formats]="formats" [modules]="modules" (onEditorCreated)="created($event)"></quill-editor>
</div>
<div class="emoji-picker">
<emoji-mart [custom]="customEmojis" [set]="set" title="Pick an emoji" emoji="slightly-smiling-face" [style]="{ width: 'none' }" (emojiClick)="insertEmoji($event)"></emoji-mart>
</div>
<!-- ... -->
Check out the source code for further informations.
Make sure to include @nutrify/quill-emoji-mart-picker and use following Quill modules inside your Quill wrapper:
modules: {
// ...
'emoji-module': {
emojiData: emojis,
customEmojiData: this.customEmojis,
preventDrag: true,
showTitle: true,
indicator: '*',
convertEmoticons: true,
convertShortNames: true,
set: () => this.set
},
toolbar: false
}
Quill Emoji Mart Picker exports insertEmoji, if you want to use it with Emoji Mart:
import { Emoji } from '@nutrify/quill-emoji-mart-picker';
insertEmoji(emojiClickEvent) {
// It expects the Quill instance and Emoji Mart's click event
Emoji.insertEmoji(quillInstance, emojiClickEvent);
}
The emoji data needs to be hand over to the quill module.
component.ts:
import { emojis } from '@nutrify/ngx-emoji-mart-picker/ngx-emoji/esm5/data/emojis';
// ...
this.modules = {
'emoji-module': {
emojiData: emojis,
// ...
}
};
// ...
component.ts:
import { emojis } from '@ctrl/ngx-emoji-mart/ngx-emoji/esm5/data/emojis';
// ...
this.modules = {
'emoji-module': {
emojiData: emojis,
// ...
}
};
// ...
Check if your Emoji Mart wrapper exports CompressedEmojiData[]
, else
use Quill Emoji Mart Picker's emoji data:
import { emojis } from '@nutrify/quill-emoji-mart-picker';
// ...
modules: {
// ...
'emoji-module': {
emojiData: emojis,
customEmojiData: this.customEmojis,
preventDrag: true,
showTitle: true,
indicator: '*',
convertEmoticons: true,
convertShortNames: true,
set: () => this.set
},
toolbar: false
}
// ...
Property | Default | Description |
---|---|---|
emojiData | Exported CompressedEmojiData[] of either Emoji Mart or Emoji Mart Picker. If you are not using any of these, use: import { emojis } from '@nutrify/ngx-emoji-mart-picker'; | |
customEmojiData | undefined | `ICustomEmoji[]``in the form of Emoji Mart's custom emoji Data |
showTitle | true | Shows a title when hovering over the emoji |
preventDrag | true | Prevents emoji images from dragging |
indicator | ':' | Specifies the pre- and postfix of emoji short names e.g. :grin: . For Emoji Mart Picker choose: * |
convertEmoticons | true | Converts emoticons into emoji images as well * |
convertShortNames | true | Converts short names into emoji images as well * |
set | () => 'apple' | Function that returns what set of emojis should be used. The return values may be: 'apple' | 'google' | 'twitter' | 'emojione' | 'messenger' | 'facebook' |
backgroundImageFn | DEFAULT_BACKGROUNDFN | Function that returns the emoji sheet image. It uses the standard Emoji Mart image by default. The format has to match Emoji Mart's expected layout |
* Can not use convertEmoticons and convertShortNames at the same time if not using Emoji Mart Picker
If you need more functionality than the insertEmoji method, the emoji blot accepts a unicode emoji character or an emojiData object.
It can be used this way:
// myEmoji may be a unicode character or an object
new Delta().insert({ emoji: myEmoji });
The plugin uses CSS for styling.
Just import the stylesheet and apply changes to it.
@import '~@nutrify/quill-emoji-mart-picker/emoji.quill';
angular-cli.json:
"styles": [
"styles.css",
"../node_modules/@nutrify/quill-emoji-mart-picker/emoji.quill.css"
]
FAQs
Quill Emoji Plugin
The npm package @nutrify/quill-emoji-mart-picker receives a total of 433 weekly downloads. As such, @nutrify/quill-emoji-mart-picker popularity was classified as not popular.
We found that @nutrify/quill-emoji-mart-picker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.