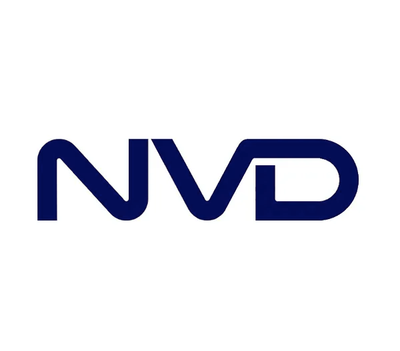
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
@nuxtjs/axios
Advanced tools
@nuxtjs/axios is a Nuxt.js module that integrates the Axios HTTP client with Nuxt.js applications. It simplifies making HTTP requests in a Nuxt.js project by providing a consistent API and additional features tailored for Nuxt.js.
Making GET Requests
This feature allows you to make GET requests to fetch data from an API endpoint. The example demonstrates how to use the $axios.$get method within the asyncData lifecycle hook to fetch data before rendering the page.
async asyncData({ $axios }) {
const data = await $axios.$get('https://api.example.com/data');
return { data };
}
Making POST Requests
This feature allows you to make POST requests to send data to an API endpoint. The example shows how to use the $axios.$post method to submit form data to a server.
async submitForm({ $axios }, formData) {
const response = await $axios.$post('https://api.example.com/submit', formData);
return response;
}
Setting Base URL
This feature allows you to set a base URL for all Axios requests. The example demonstrates how to configure the base URL in the Nuxt.js configuration file.
export default {
axios: {
baseURL: 'https://api.example.com'
}
}
Handling Errors
This feature allows you to handle errors that occur during HTTP requests. The example shows how to use a try-catch block to catch errors and handle them appropriately within the asyncData lifecycle hook.
async asyncData({ $axios, error }) {
try {
const data = await $axios.$get('https://api.example.com/data');
return { data };
} catch (e) {
error({ statusCode: 500, message: 'Internal Server Error' });
}
}
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and is the core library that @nuxtjs/axios is built upon. While @nuxtjs/axios is tailored for Nuxt.js, axios can be used in any JavaScript environment.
Vue-resource is an HTTP client for Vue.js applications. It provides services for making web requests and handling responses using a simple and easy-to-understand API. Compared to @nuxtjs/axios, vue-resource is more tightly integrated with Vue.js but lacks some of the advanced features and flexibility of axios.
Fetch is a native JavaScript API for making HTTP requests. It is built into modern browsers and provides a more low-level approach compared to axios. While fetch is more lightweight and does not require additional dependencies, it lacks some of the convenience features provided by axios, such as request and response interceptors.
Secure and Easy Axios integration with Nuxt.js.
If you are coming from an older release please be sure to read Migration Guide.
✓ Automatically set base URL for client & server side
✓ Exposes setToken
function to $axios
so we can easily and globally set authentication tokens
✓ Automatically enables withCredentials
when requesting to base URL
✓ Proxy request headers in SSR (Useful for auth)
✓ Fetch Style requests
✓ Integrated with Nuxt.js Progressbar while making requests
✓ Integrated with Proxy Module
✓ Auto retry requests with axios-retry
Install with yarn:
yarn add @nuxtjs/axios
Install with npm:
npm install @nuxtjs/axios
nuxt.config.js
{
modules: [
'@nuxtjs/axios',
],
axios: {
// proxyHeaders: false
}
}
asyncData
async asyncData({ app }) {
const ip = await app.$axios.$get('http://icanhazip.com')
return { ip }
}
methods
/created
/mounted
/etc
methods: {
async fetchSomething() {
const ip = await this.$axios.$get('http://icanhazip.com')
this.ip = ip
}
}
nuxtServerInit
async nuxtServerInit ({ commit }, { app }) {
const ip = await app.$axios.$get('http://icanhazip.com')
commit('SET_IP', ip)
}
// In store
{
actions: {
async getIP ({ commit }) {
const ip = await this.$axios.$get('http://icanhazip.com')
commit('SET_IP', ip)
}
}
}
If you need to customize axios by registering interceptors and changing global config, you have to create a nuxt plugin.
nuxt.config.js
{
modules: [
'@nuxtjs/axios',
],
plugins: [
'~/plugins/axios'
]
}
plugins/axios.js
export default function ({ $axios, redirect }) {
$axios.onRequest(config => {
console.log('Making request to ' + config.url)
})
$axios.onError(error => {
const code = parseInt(error.response && error.response.status)
if (code === 400) {
redirect('/400')
}
})
}
Axios plugin provides helpers to register axios interceptors easier and faster.
onRequest(config)
onResponse(response)
onError(err)
onRequestError(err)
onResponseError(err)
This functions don't have to return anything by default.
Example: (plugins/axios.js
)
export default function ({ $axios, redirect }) {
$axios.onError(error => {
if(error.code === 500) {
redirect('/sorry')
}
})
}
Axios plugin also supports fetch style requests with $
prefixed methods:
// Normal usage with axios
let data = (await $axios.get('...')).data
// Fetch Style
let data = await $axios.$get('...')
setHeader(name, value, scopes='common')
Axios instance has a helper to easily set any header.
Parameters:
common
meaning all types of requestsget
, post
, delete
, ...// Adds header: `Authorization: 123` to all requests
this.$axios.setHeader('Authorization', '123')
// Overrides `Authorization` header with new value
this.$axios.setHeader('Authorization', '456')
// Adds header: `Content-Type: application/x-www-form-urlencoded` to only post requests
this.$axios.setHeader('Content-Type', 'application/x-www-form-urlencoded', [
'post'
])
// Removes default Content-Type header from `post` scope
this.$axios.setHeader('Content-Type', false, ['post'])
setToken(token, type, scopes='common')
Axios instance has an additional helper to easily set global authentication header.
Parameters:
Bearer
).common
meaning all types of requestsget
, post
, delete
, ...// Adds header: `Authorization: 123` to all requests
this.$axios.setToken('123')
// Overrides `Authorization` header with new value
this.$axios.setToken('456')
// Adds header: `Authorization: Bearer 123` to all requests
this.$axios.setToken('123', 'Bearer')
// Adds header: `Authorization: Bearer 123` to only post and delete requests
this.$axios.setToken('123', 'Bearer', ['post', 'delete'])
// Removes default Authorization header from `common` scope (all requests)
this.$axios.setToken(false)
You can pass options using module options or axios
section in nuxt.config.js
prefix
, host
and port
This options are used for default values of baseURL
and browserBaseURL
.
Can be customized with API_PREFIX
, API_HOST
(or HOST
) and API_PORT
(or PORT
) environment variables.
Default value of prefix
is /
.
baseURL
http://[HOST]:[PORT][PREFIX]
Base URL which is used and prepended to make requests in server side.
Environment variable API_URL
can be used to override baseURL
.
browserBaseURL
baseURL
(or prefix
when options.proxy
is enabled)Base URL which is used and prepended to make requests in client side.
Environment variable API_URL_BROWSER
can be used to override browserBaseURL
.
https
false
If set to true
, http://
in both baseURL
and browserBaseURL
will be changed into https://
.
progress
true
Integrate with Nuxt.js progress bar to show a loading bar while making requests. (Only on browser, when loading bar is available.)
proxy
false
You can easily integrate Axios with Proxy Module and is much recommended to prevent CORS and deployment problems.
nuxt.config.js
{
modules: [
'@nuxtjs/axios'
],
axios: {
proxy: true // Can be also an object with default options
},
proxy: {
'/api/': 'http://api.example.com',
'/api2/': 'http://api.another-website.com'
}
}
Note: It is not required to manually register @nuxtjs/proxy
module, but it does need to be in your dependencies.
Note: /api/
will be added to all requests to the API end point. If you need to remove it use pathRewrite
:
proxy: {
'/api/': { target: 'http://api.example.com', pathRewrite: {'^/api/': ''} }
}
retry
false
Automatically intercept failed requests and retries them whenever posible using axios-retry.
By default, number of retries will be 3 times, if retry
value is set to true
. You can change it by passing an object like this:
axios: {
retry: { retries: 3 }
}
credentials
false
Adds an interceptor to automatically set withCredentials
config of axios when requesting to baseUrl
which allows passing authentication headers to backend.
debug
false
Adds interceptors to log request and responses.
proxyHeaders
true
In SSR context, sets client request header as axios default request headers. This is useful for making requests which need cookie based auth on server side. Also helps making consistent requests in both SSR and Client Side code.
NOTE: If directing requests at a url protected by CloudFlare's CDN you should set this to false to prevent CloudFlare from mistakenly detecting a reverse proxy loop and returning a 403 error.
proxyHeadersIgnore
['host', 'accept']
Only efficient when proxyHeaders
is set to true. Removes unwanted request headers to the API backend in SSR.
MIT License - Copyright (c) 2017 Nuxt Community
FAQs
Secure and easy Axios integration with Nuxt.js
The npm package @nuxtjs/axios receives a total of 97,716 weekly downloads. As such, @nuxtjs/axios popularity was classified as popular.
We found that @nuxtjs/axios demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.