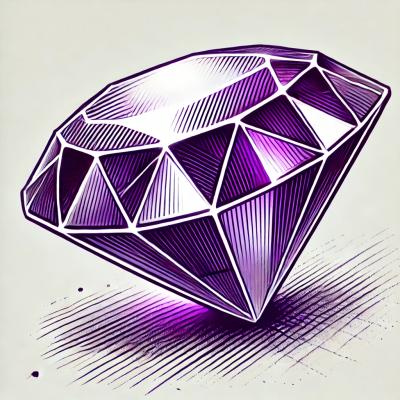
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@obliczeniowo/elementary
Advanced tools
This library was generated with [Angular CLI](https://github.com/angular/angular-cli) version 11.0.9.
17-10-2021 - v 1.1.13 move math class
17-10-2021 - v 1.1.11 move colors classes to separated lib folder call classes
17-10-2021 - v 1.1.10 change name lib-status-bar to lib-ball-progress-bar and move it to separated module
16-10-2021 - v 1.1.9 add autoIncreaseDecrease option for x & y axis
16-10-2021 - v 1.1.8 add dp option for scrale x and y
16-10-2021 - v 1.1.7 disable option for drawing line
16-10-2021 - v 1.1.6 add posibility of displaying points on diagram
15-10-2021 - v 1.1.5 add more then one settins for vector of points styles
15-10-2021 - v 1.1.4 add button for downloading diagram as PDF in lib-linear-diagram-2d
14-10-2021 - v 1.1.3 add posibility of add more then one vector of points for lib-linear-diagram-2d
Upgrade to Angular 12!
Inside library You can find components:
lib-post-diagram
lib-ball-progress-bar
lib-color-picker-control
lib-color-component
lib-horizontal-progress-bar
lib-linear-diagram-2d
Install package:
npm i @obliczeniowo/elementary
Import module:
import { PostDiagramModule } from '@obliczeniowo/elementary/lib/post-diagram';
...
imports: [
BrowserModule,
AppRoutingModule,
PostDiagramModule // here is import
],
Inside of your own component:
import { Component } from '@angular/core';
import { ColorHSV, ColorRGB } from '@obliczeniowo/elementary';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
title = 'Diagram';
xLabel = 'xLabel';
yLabel = 'yLabel';
diagramData: { [key: string]: number } = {
'key 1': 100,
'key 2': 200,
'key 3': 1500,
'key 4': 1,
'key 5': 2,
'key 6': 145,
'key 7': 200,
'key 8': 340,
'key 9': 885,
'key 10': 1234,
'key 11': 450,
'key 12': 800,
'key 13': 3900,
'key 14': 1300,
'key 15': 1200,
'key 16': 2500,
'key 17': 1300,
'key 18': 1200,
'key 19': 2500,
};
colorFunction = (
index: number,
dataLength: number,
value: number,
max: number
): ColorRGB => {
return ColorHSV.createColorHSV(
180 - (180 * value) / max,
0.5,
255
).convertToRGB();
}
}
In HTML:
<lib-post-diagram
[diagramData]="diagramData"
[diagramTitle]="title"
[xLabel]="xLabel"
[yLabel]="yLabel"
[colorFunction]="colorFunction"
>
</lib-post-diagram>
Live example of working component is on my page: https://obliczeniowo.com.pl/1142
Example of use in html without 3d effect:
<lib-ball-progress-bar [value]="20" [effect3d]="false"></lib-ball-progress-bar>
Example of use in html with 3d effect
<lib-ball-progress-bar [value]="20"></lib-ball-progress-bar>
WARNING! On Firefox component not working correctly
Live example of working component is on my page: https://obliczeniowo.com.pl/1144
Example of use in html for color picker with button:
<lib-color-picker-control></lib-color-picker-control>
Example of use in html for color component withour button:
<lib-color-component></lib-color-component>
Example of use in html for horizontal progress bar:
<lib-horizontal-progress-bar [value]="value" class="black"><lib-horizontal-progress-bar>
To change default colors use SCSS mixin:
@mixin vertical-progress-bar($class, $value-color, $background-color, $value-background-color, $frame-color) {
::ng-deep .#{$class} {
#value {
fill: $value-color !important;
}
#background-pattern {
fill: $background-color !important;
}
#value-pattern {
fill: $value-background-color !important;
}
#border-rect {
stroke: $frame-color !important;
}
}
}
and make some kind of include stuff:
@include vertical-progress-bar('black', white, #bebebe, black, #bebebe);
In html
<lib-linear-diagram-2d [points]="points" [labels]="{ x: xLabel, y: yLabel, title: title }">
</lib-linear-diagram-2d>
in ts file of component:
points: Point2D[] = []; // imported from import { Point2D } from '@obliczeniowo/elementary/lib/linear-diagram';
constructor() {
for (let i = 0; i < 100; i++) {
this.points.push(
new Point2D(i, Math.random() * 100)
);
}
}
Example with changing settings of vector points line color and drawing points:
<lib-linear-diagram-2d
[points]="points"
[labels]="{ x: xLabel, y: yLabel, title: title }"
[options]="{
set: [{
color: '#ffaa00',
stroke: 1,
linePattern: LinePattern.NONE,
drawPoint: PointType.CIRCLE
},
{
color: '#aaff00',
stroke: 2,
linePattern: LinePattern.NONE,
drawPoint: PointType.X
},
{
color: '#aa00ff',
stroke: 2,
linePattern: LinePattern.NONE,
drawPoint: PointType.STAR
},
{
color: '#aabbff',
stroke: 1,
linePattern: LinePattern.DISABLED,
drawPoint: PointType.STAR
}]
}"
>
</lib-linear-diagram-2d>
Where LinePattern need to be imported in ts component file:
import { LinePattern } from 'dist/components/lib/linear-diagram';
and then in component class:
LinePattern = LinePattern;
You can override more options just using interface:
export interface LinearDiagram2DOptions {
xAxis?: AxisOptions;
yAxis?: AxisOptions;
xMinMax?: MinMax;
yMinMax?: MinMax;
greed?: GridOptions;
set?: BaseDrawingOptions[];
}
You can see live example on my home page: https://obliczeniowo.com.pl/1239
FAQs
Library made in Angular version 18
The npm package @obliczeniowo/elementary receives a total of 55 weekly downloads. As such, @obliczeniowo/elementary popularity was classified as not popular.
We found that @obliczeniowo/elementary demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.