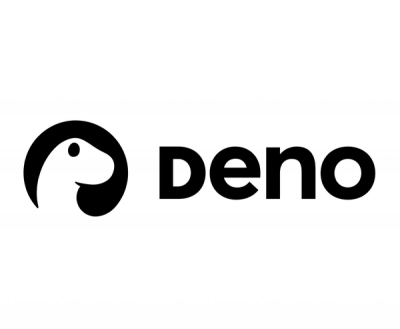
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@octokit/auth-app
Advanced tools
@octokit/auth-app is an npm package that provides authentication for GitHub Apps. It allows you to authenticate as a GitHub App or as an installation of a GitHub App, enabling you to interact with the GitHub API in a secure and efficient manner.
Authenticate as a GitHub App
This feature allows you to authenticate as a GitHub App using the app's ID, private key, client ID, and client secret. The code sample demonstrates how to create an app authentication object.
const { createAppAuth } = require('@octokit/auth-app');
const auth = createAppAuth({
id: process.env.APP_ID,
privateKey: process.env.PRIVATE_KEY,
clientId: process.env.CLIENT_ID,
clientSecret: process.env.CLIENT_SECRET
});
const appAuthentication = await auth({ type: 'app' });
console.log(appAuthentication);
Authenticate as an installation
This feature allows you to authenticate as an installation of a GitHub App. The code sample demonstrates how to create an installation authentication object using the installation ID.
const { createAppAuth } = require('@octokit/auth-app');
const auth = createAppAuth({
id: process.env.APP_ID,
privateKey: process.env.PRIVATE_KEY,
clientId: process.env.CLIENT_ID,
clientSecret: process.env.CLIENT_SECRET
});
const installationAuthentication = await auth({
type: 'installation',
installationId: process.env.INSTALLATION_ID
});
console.log(installationAuthentication);
Authenticate as an installation with refresh
This feature allows you to authenticate as an installation and refresh the token if needed. The code sample demonstrates how to create an installation authentication object with token refresh.
const { createAppAuth } = require('@octokit/auth-app');
const auth = createAppAuth({
id: process.env.APP_ID,
privateKey: process.env.PRIVATE_KEY,
clientId: process.env.CLIENT_ID,
clientSecret: process.env.CLIENT_SECRET
});
const { token } = await auth({
type: 'installation',
installationId: process.env.INSTALLATION_ID,
refresh: true
});
console.log(token);
The 'octokit' package is a comprehensive toolkit for interacting with the GitHub API. It includes various plugins for authentication, including GitHub App authentication. Compared to @octokit/auth-app, 'octokit' provides a broader range of functionalities beyond just authentication.
The 'node-github' package is another library for interacting with the GitHub API. It supports various authentication methods, including OAuth and personal access tokens. While it offers similar functionalities, it is not as specialized in GitHub App authentication as @octokit/auth-app.
The 'github-api' package is a lightweight library for interacting with the GitHub API. It supports basic authentication methods but does not provide the same level of support for GitHub App authentication as @octokit/auth-app.
GitHub App authentication for JavaScript
@octokit/auth-app
implements one of GitHub’s authentication strategies.
It implements authentication using a JSON Web Token for apps as well as installation access tokens.
import { request } from "@octokit/request";
import { createAppAuth } from "@octokit/auth-app";
const auth = createAppAuth({
id: 1,
privateKey: "-----BEGIN RSA PRIVATE KEY-----\n..."
});
(async () => {
// Retrieve JSON Web Token (JWT) to authenticate as app
const appAuthentication = await auth();
const { data: appDetails } = await request("GET /app", {
headers: appAuthentication.headers,
previews: ["machine-man"]
});
// Retrieve installation access token
const installationAuthentication = await auth({ installationId: 123 });
const { data: repositories } = await request(
"GET /installation/repositories",
{
headers: installationAuthentication.headers,
previews: ["machine-man"]
}
);
// Retrieve JSON Web Token (JWT) or installation access token based on request url
const url = "/installation/repositories";
const authentication = await auth({
installationId: 123,
url
});
const { data: repositories } = await request(url, {
headers: authentication.headers,
previews: ["machine-man"]
});
})();
name | type | description |
---|---|---|
id
|
number
| Required. Find App ID on the app’s about page in settings. |
privateKey
|
string
|
Required. Content of the *.pem file you downloaded from the app’s about page. You can generate a new private key if needed.
|
installationId
|
number
|
A default installationId to be used when calling auth() .
|
request
|
function
|
You can pass in your own @octokit/request instance. For usage with enterprise, set baseUrl to the hostname + /api/v3 . Example:
|
cache
|
object
|
Installation tokens expire after an hour. By default, @octokit/auth-app is caching up to 15000 tokens simultaneously using lru-cache. You can pass your own cache implementation by passing options.cache.{get,set} to the constructor. Example:
|
name | type | description |
---|---|---|
installationId
|
number
|
Required if url , repositoryIds or permissions passed, unless a default installationId option was passed to createAppAuth() . ID of installation to retrieve authentication for.
|
repositoryIds
|
array of string
| The `id`s of the repositories that the installation token can access. |
premissions
|
object
| The permissions granted to the access token. The permissions object includes the permission names and their access type. For a complete list of permissions and allowable values, see GitHub App permissions. |
url
|
string
|
An absolute URL or endpoint route path. Examples:
url , the resulting authentication object is either a JWT or an installation token.
|
refresh
|
boolean
|
Installation tokens expire after one hour. By default, tokens are cached and returned from cache until expired. To bypass and update a cached token for the given installationId , set refresh to true .Defaults to false .
|
There are two possible results
installationId
was not passed to auth()
url
passed to auth()
matches an endpoint that requires JWT authentication.installationId
passed to auth()
url
passed to auth()
does not match an endpoint that requires JWT authentication.name | type | description |
---|---|---|
type
|
string
|
"app"
|
token
|
string
| The JSON Web Token (JWT) to authenticate as the app. |
appId
|
number
| GitHub App database ID. |
expiration
|
number
|
Number of seconds from 1970-01-01T00:00:00Z UTC. A Date object can be created using new Date(authentication.expiration * 1000) .
|
headers
|
object
|
{ authorization } - value for the "Authorization" header.
|
query
|
object
|
{} - not used
|
name | type | description |
---|---|---|
type
|
string
|
"token"
|
token
|
string
| The installation access token. |
tokenType
|
string
|
"installation"
|
installationId
|
number
| Installation database ID. |
expiresAt
|
string
|
Timestamp in UTC format, e.g. 2019-06-11T22:22:34Z .
|
repositoryIds
|
array of numbers
|
Only present if repositoryIds option passed to auth(options) .
|
permissions
|
object
|
An object where keys are the permission name and the value is either "read" or "write" . See the list of all GitHub App Permissions.
|
singleFileName
|
string
|
If the single file permission is enabled, the singleFileName property is set to the path of the accessible file.
|
headers
|
object
|
{ authorization } - value for the "Authorization" header.
|
query
|
object
|
{} - not used
|
FAQs
GitHub App authentication for JavaScript
The npm package @octokit/auth-app receives a total of 1,001,489 weekly downloads. As such, @octokit/auth-app popularity was classified as popular.
We found that @octokit/auth-app demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.