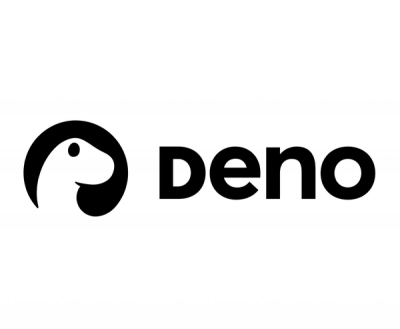
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@octokit/core
Advanced tools
The @octokit/core package is a core library for GitHub's REST API v3. It provides a simplified interface to interact with GitHub's API, allowing developers to authenticate, send requests, and process responses. This package is part of the Octokit SDK, which is a collection of libraries for working with the GitHub API.
Authentication
Authenticate with the GitHub API using a personal access token. This is essential for performing actions that require GitHub permissions.
{
const { Octokit } = require('@octokit/core');
const octokit = new Octokit({ auth: `personal_access_token` });
}
Send Requests
Send requests to the GitHub API. This example fetches a user's profile information using their username.
{
const { Octokit } = require('@octokit/core');
const octokit = new Octokit();
async function fetchUser() {
const response = await octokit.request('GET /users/{username}', {
username: 'octocat'
});
console.log(response.data);
}
fetchUser();
}
Custom Requests
Create custom requests to perform specific actions, such as creating an issue in a repository. This demonstrates how to use the package to interact with various parts of the GitHub API.
{
const { Octokit } = require('@octokit/core');
const octokit = new Octokit();
async function createIssue(owner, repo, title, body) {
const response = await octokit.request('POST /repos/{owner}/{repo}/issues', {
owner,
repo,
title,
body
});
console.log(response.data);
}
createIssue('octocat', 'Hello-World', 'New issue title', 'Issue body content');
}
The 'github' package is a Node.js wrapper for the GitHub API. It offers similar functionalities to @octokit/core but is less modular and not as actively maintained. @octokit/core benefits from being part of the larger Octokit SDK, which provides more comprehensive tools and a consistent API design.
Similar to 'github', 'node-github' is another wrapper for the GitHub API designed for Node.js. It provides access to the GitHub API but lacks the modularity and extensibility of @octokit/core. The Octokit libraries, including @octokit/core, are officially maintained by GitHub, offering better support and integration with GitHub's evolving API.
Extendable client for GitHub's REST & GraphQL APIs
Browsers |
Load @octokit/core directly from cdn.pika.dev
|
---|---|
Node |
Install with
|
const octokit = new Octokit({ auth: `secret123` });
const response = await octokit.request("GET /orgs/:org/repos", {
org: "octokit",
type: "private"
});
See https://github.com/octokit/request.js for full documentation of the .request
method.
const octokit = new Octokit({ auth: `secret123` });
const response = await octokit.graphql(
`query ($login: String!) {
organization(login: $login) {
repositories(privacy: PRIVATE) {
totalCount
}
}
}`,
{ login: "octokit" }
);
See https://github.com/octokit/graphql.js for full documentation of the .graphql
method.
The auth
option is a string and can be one of
GITHUB_TOKEN
environment variable)More complex authentication strategies will be supported by passing an @octokit/auth instance (🚧 currently work in progress).
You can customize Octokit's request lifecycle with hooks.
octokit.hook.before("request", async options => {
validate(options);
});
octokit.hook.after("request", async (response, options) => {
console.log(`${options.method} ${options.url}: ${response.status}`);
});
octokit.hook.error("request", async (error, options) => {
if (error.status === 304) {
return findInCache(error.headers.etag);
}
throw error;
});
octokit.hook.wrap("request", async (request, options) => {
// add logic before, after, catch errors or replace the request altogether
return request(options);
});
See before-after-hook for more documentation on hooks.
Octokit’s functionality can be extended using plugins. THe Octokit.plugin()
method accepts a function or an array of functions and returns a new constructor.
A plugin is a function which gets two arguments:
// index.js
const MyOctokit = require("@octokit/core").plugin([
require("./lib/my-plugin"),
require("octokit-plugin-example")
]);
const octokit = new MyOctokit({ greeting: "Moin moin" });
octokit.helloWorld(); // logs "Moin moin, world!"
octokit.request("GET /"); // logs "GET / - 200 in 123ms"
// lib/my-plugin.js
module.exports = (octokit, options = { greeting: "Hello" }) => {
// add a custom method
octokit.helloWorld = () => console.log(`${options.greeting}, world!`);
// hook into the request lifecycle
octokit.hook.wrap("request", async (request, options) => {
const time = Date.now();
const response = await request(options);
console.log(
`${options.method} ${options.url} – ${response.status} in ${Date.now() -
time}ms`
);
return response;
});
};
FAQs
Extendable client for GitHub's REST & GraphQL APIs
The npm package @octokit/core receives a total of 8,213,286 weekly downloads. As such, @octokit/core popularity was classified as popular.
We found that @octokit/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.