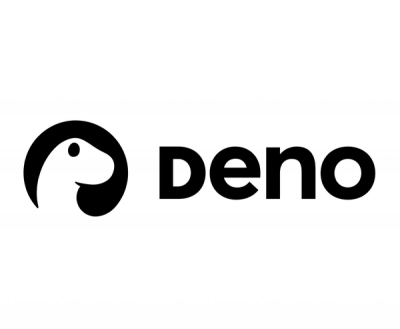
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@octokit/oauth-app
Advanced tools
@octokit/oauth-app is a Node.js library that simplifies the process of implementing GitHub OAuth applications. It provides a set of tools to handle OAuth flows, manage tokens, and interact with GitHub's API securely.
OAuth App Initialization
This feature allows you to initialize an OAuth application with your GitHub client ID and client secret.
const { OAuthApp } = require('@octokit/oauth-app');
const app = new OAuthApp({
clientId: 'your-client-id',
clientSecret: 'your-client-secret'
});
Handling OAuth Web Flow
This feature allows you to handle the OAuth web flow, including exchanging the authorization code for an access token and using the token to authenticate API requests.
app.on('oauth', async ({ token, octokit }) => {
const { data: { login } } = await octokit.request('GET /user');
console.log('Authenticated as', login);
});
Token Management
This feature allows you to manage tokens, including creating, refreshing, and revoking tokens.
app.on('token', async ({ token }) => {
console.log('New token created:', token);
});
passport-github is a GitHub authentication strategy for Passport, a popular authentication middleware for Node.js. It provides a way to authenticate users using their GitHub accounts. Compared to @octokit/oauth-app, passport-github is more focused on user authentication and session management, while @octokit/oauth-app provides a more comprehensive set of tools for managing OAuth flows and interacting with GitHub's API.
simple-oauth2 is a library that provides a simple and consistent way to handle OAuth2 flows in Node.js applications. It supports various OAuth2 providers, including GitHub. Compared to @octokit/oauth-app, simple-oauth2 is more generic and can be used with multiple OAuth2 providers, while @octokit/oauth-app is specifically designed for GitHub OAuth applications.
node-oauth2-server is a complete, framework-agnostic implementation of the OAuth2 server specification for Node.js. It allows you to create your own OAuth2 server and manage tokens. Compared to @octokit/oauth-app, node-oauth2-server is more focused on providing a full OAuth2 server implementation, while @octokit/oauth-app is focused on client-side OAuth flows and interacting with GitHub's API.
GitHub OAuth toolset for Node.js
Browsers |
|
---|---|
Node |
Install with
|
public/
folder: https://glitch.com/~github-oauth-clientname | type | description |
---|---|---|
clientId
|
number
| Required. Find Client ID on the app’s about page in settings. |
clientSecret
|
number
| Required. Find Client Secret on the app’s about page in settings. |
allowSignup
|
boolean
|
Sets the default value for app.getAuthorizationUrl(options) .
|
defaultScopes
|
Array of strings
|
Sets the default |
log
|
object
|
Used for internal logging. Defaults to console .
|
Octokit
|
Constructor
|
You can pass in your own Octokit constructor with custom defaults and plugins. The Octokit Constructor must accept For usage with enterprise, set
Defaults to |
app.on(eventName, eventHandler)
Called whenever a new OAuth access token is created for a user. It accepts two parameters, an event name and a function with one argument
app.on("token.created", async context => {
const { data } = await context.octokit.request("GET /user");
app.log.info(`New token created for ${data.login}`);
});
The eventName
can be one of (or an array of)
token.created
token.reset
token.deleted
token.before_deleted
authorization.before_deleted
authorization.deleted
All event handlers are awaited before continuing.
context
can have the following properties
property | type | description |
---|---|---|
context.name
|
string
|
Name of the event. One of: token , authorization
|
context.action
|
string
|
Action of the event. One of: created , reset , deleted , before_deleted
|
context.token
|
string
| The OAuth access token. |
context.octokit
|
Octokit instance
|
Authenticated instance using the Not set for |
scopes
|
Array of strings
|
An array of scopes the Not set for |
app.octokit
Octokit instance with OAuth App authentication. Uses Octokit
constructor option
app.getAuthorizationUrl(options)
Returns a URL string.
const url = app.getAuthorizationUrl({
state: "state123",
scopes: ["repo"]
});
name | type | description |
---|---|---|
redirectUrl
|
string
| The URL in your application where users will be sent after authorization. See Redirect URLs in GitHub’s Developer Guide. |
login
|
string
| Suggests a specific account to use for signing in and authorizing the app. |
scopes
|
array of strings
| An array of scope names (or: space-delimited list of scopes). If not provided, scope defaults to an empty list for users that have not authorized any scopes for the application. For users who have authorized scopes for the application, the user won't be shown the OAuth authorization page with the list of scopes. Instead, this step of the flow will automatically complete with the set of scopes the user has authorized for the application. For example, if a user has already performed the web flow twice and has authorized one token with user scope and another token with repo scope, a third web flow that does not provide a scope will receive a token with user and repo scope. |
state
|
string
|
An unguessable random string. It is used to protect against cross-site request forgery attacks.
Defaults to Math.random().toString(36).substr(2) .
|
allowSignup
|
boolean
|
Whether or not unauthenticated users will be offered an option to sign up for GitHub during the OAuth flow. The default is true . Use false in the case that a policy prohibits signups.
|
app.createToken(options)
const { token, scopes } = await app.createToken({
state: "state123",
code: "code123"
});
name | type | description |
---|---|---|
code
|
string
|
Required. Pass the code that was passed as ?code query parameter in the authorization redirect URL.
|
state
|
string
|
Required. Pass the state that was passed as ?state query parameter in the authorization redirect URL.
|
Resolves with result
name | type | description |
---|---|---|
result.token
|
string
| The OAuth access token |
result.scopes
|
array of strings
| The names of the scopes the token has access to |
app.checkToken(options)
try {
const { created_at, app, user } = await app.checkToken({ token });
console.log(
`token valid, created on %s by %s for %s`,
created_at,
user.login,
app.name
);
} catch (error) {
// token invalid or request error
}
name | type | description |
---|---|---|
token
|
string
| Required. |
Resolves with response body from "Check a token" request.
app.resetToken(options)
const { token } = await app.resetToken({
token: "token123"
});
// "token123" is no longer valid. Use `token` instead
name | type | description |
---|---|---|
token
|
string
| Required. |
Resolves with response body from "Reset a token" request.
app.deleteToken(options)
await app.deleteToken({
token: "token123"
});
// "token123" is no longer valid.
name | type | description |
---|---|---|
token
|
string
| Required. |
Resolves with response body from "Delete a token" request.
app.deleteAuthorization(options)
await app.deleteAuthorization({
token: "token123"
});
// "token123" is no longer valid, and no tokens can be created until the app gets re-authorized.
name | type | description |
---|---|---|
token
|
string
| Required. |
Resolves with response body from "Delete an app authorization" request.
Each method can be loaded separately to minimize the payload. That is particularly useful for serverless environment, as the payload will be the smallest possible.
const {
getAuthorizationUrl,
createToken,
checkToken,
resetToken,
deleteToken,
deleteAuthorization
} = require("@octokit/oauth-app");
getAuthorizationUrl(options)
Returns a URL string.
const { getAuthorizationUrl } = require("@octokit/oauth-app");
const url = getAuthorizationUrl({
clientId: "0123",
state: "state123"
});
name | type | description |
---|---|---|
clientId
|
string
| Required. The client ID you received from GitHub when you registered. |
state
|
string
|
Required. An unguessable random string. It is used to protect against cross-site request forgery attacks.
Defaults to Math.random().toString(36).substr(2) .
|
redirectUrl
|
string
| The URL in your application where users will be sent after authorization. See Redirect URLs in GitHub’s Developer Guide. |
login
|
string
| Suggests a specific account to use for signing in and authorizing the app. |
scopes
|
array of strings
| An array of scope names (or: space-delimited list of scopes). If not provided, scope defaults to an empty list for users that have not authorized any scopes for the application. For users who have authorized scopes for the application, the user won't be shown the OAuth authorization page with the list of scopes. Instead, this step of the flow will automatically complete with the set of scopes the user has authorized for the application. For example, if a user has already performed the web flow twice and has authorized one token with user scope and another token with repo scope, a third web flow that does not provide a scope will receive a token with user and repo scope. |
allowSignup
|
boolean
|
Whether or not unauthenticated users will be offered an option to sign up for GitHub during the OAuth flow. The default is true . Use false in the case that a policy prohibits signups.
|
log
|
object
|
When invalid options are passed, warnings are logged using log.warn(message) . Defaults to console .
|
baseUrl
|
string
|
When using GitHub Enterprise Server, set the baseUrl to the origin, e.g. https://github.my-enterprise.com/ .
|
createToken(options)
const { createToken } = require("@octokit/oauth-app");
const { token, scopes } = await createToken({
clientId: "0123",
clientSecret: "0123secret",
state: "state123",
code: "code123"
});
name | type | description |
---|---|---|
clientId
|
number
| Required. Find Client ID on the app’s about page in settings. |
clientSecret
|
number
| Required. Find Client Secret on the app’s about page in settings. |
code
|
string
|
Required. Pass the code that was passed in the ?code query parameter in the authorization redirect URL.
|
state
|
string
|
Required. Pass the state that was passed in the ?state query parameter in the authorization redirect URL.
|
Resolves with result
name | type | description |
---|---|---|
result.token
|
string
| The OAuth access token |
result.scopes
|
array of strings
| The names of the scopes the token has access to |
checkToken(options)
const { checkToken } = require("@octokit/oauth-app");
try {
const { created_at, app, user } = await checkToken({
clientId: "0123",
clientSecret: "0123secret",
token: "token123"
});
console.log(
`token valid, created on %s by %s for %s`,
created_at,
user.login,
app.name
);
} catch (error) {
// token invalid or request error
}
name | type | description |
---|---|---|
clientId
|
number
| Required. Find Client ID on the app’s about page in settings. |
clientSecret
|
number
| Required. Find Client Secret on the app’s about page in settings. |
token
|
string
| Required. |
Resolves with response body from "Check a token" request.
resetToken(options)
const { resetToken } = require("@octokit/oauth-app");
const { token } = await resetToken({
clientId: "0123",
clientSecret: "0123secret",
token: "token123"
});
// "token123" is no longer valid. Use `token` instead
name | type | description |
---|---|---|
clientId
|
number
| Required. Find Client ID on the app’s about page in settings. |
clientSecret
|
number
| Required. Find Client Secret on the app’s about page in settings. |
token
|
string
| Required. |
Resolves with response body from "Reset a token" request.
deleteToken(options)
const { deleteToken } = require("@octokit/oauth-app");
await deleteToken({
clientId: "0123",
clientSecret: "0123secret",
token: "token123"
});
// "token123" is no longer valid
name | type | description |
---|---|---|
clientId
|
number
| Required. Find Client ID on the app’s about page in settings. |
clientSecret
|
number
| Required. Find Client Secret on the app’s about page in settings. |
token
|
string
| Required. |
Resolves with response body from "Delete a token" request.
deleteAuthorization(options)
const { deleteAuthorization } = require("@octokit/oauth-app");
await deleteAuthorization({
clientId: "0123",
clientSecret: "0123secret",
token: "token123"
});
// "token123" is no longer valid
name | type | description |
---|---|---|
clientId
|
number
| Required. Find Client ID on the app’s about page in settings. |
clientSecret
|
number
| Required. Find Client Secret on the app’s about page in settings. |
token
|
string
| Required. |
Resolves with response body from "Delete an app authorization" request.
A middle ware is a method or set of methods to handle requests for common environments.
By default, all middlewares exposes the following routes
Route | Route Description |
---|---|
GET /api/github/oauth/login | Redirects to GitHub's authorization endpoint. Accepts optional ?state and ?scopes query parameters. ?scopes is a comma-separated list of supported OAuth scope names |
GET /api/github/oauth/callback | The client's redirect endpoint. This is where the token event gets triggered |
POST /api/github/oauth/token | Exchange an authorization code for an OAuth Access token. If successful, the token event gets triggered. |
GET /api/github/oauth/token | Check if token is valid. Must authenticate using token in Authorization header. Uses GitHub's POST /applications/:client_id/token endpoint |
PATCH /api/github/oauth/token | Resets a token (invalidates current one, returns new token). Must authenticate using token in Authorization header. Uses GitHub's PATCH /applications/:client_id/token endpoint. |
DELETE /api/github/oauth/token | Invalidates current token, basically the equivalent of a logout. Must authenticate using token in Authorization header. |
DELETE /api/github/oauth/grant | Revokes the user's grant, basically the equivalent of an uninstall. must authenticate using token in Authorization header. |
getNodeMiddleware(app, options)
Native http server middleware for Node.js
const { OAuthApp, getNodeMiddleware } = require("@octokit/oauth-app");
const app = new OAuthApp({
clientId: "0123",
clientSecret: "0123secret"
});
const middleware = getNodeMiddleware(app, {
pathPrefix: "/api/github/oauth/"
});
require("http")
.createServer(getNodeMiddleware(app))
.listen(3000);
// can now receive user authorization callbacks at /api/github/oauth/callback
name | type | description |
---|---|---|
app
|
OAuthApp instance
| Required. |
options.pathPrefix
|
string
|
All exposed paths will be prefixed with the provided prefix. Defaults to |
options.onUnhandledRequest
|
function
|
Defaults to
|
See CONTRIBUTING.md
FAQs
GitHub OAuth toolset for Node.js
The npm package @octokit/oauth-app receives a total of 635,922 weekly downloads. As such, @octokit/oauth-app popularity was classified as popular.
We found that @octokit/oauth-app demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.