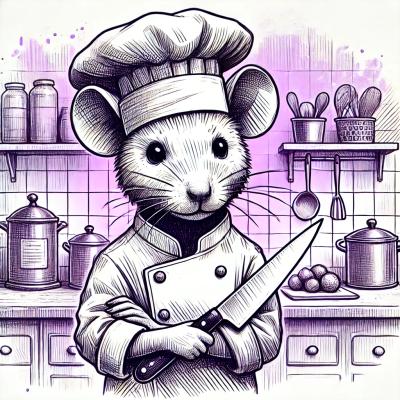
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@ones-design/dnd
Advanced tools
import { Meta } from '@storybook/blocks'
import treeStories from './dnd-tree.stories'
直接从 @dnd/core
中导出
import { DndContext } from '@dnd/core'
export { ONESDndContext }
树形结构拖拽上下文,包含拖拽指示线以及拖拽事件的处理,必须在 ONESDndContext
中使用
dropPosition
activeData
、initialActiveNodeRect
、initialCoordinates
等参数(虚拟滚动支持)@dnd/core
在虚拟滚动时会丢失数据,需要做额外保存(TODO:这个逻辑应该是公共逻辑,现在暂时放在 TreeDndContext 中处理了)<ONESDndContext draggable={isDraggable}>
<TreeDndContext<DndNodeItem> // Drag Event
onDragStart={onNodeDragStart}
onDragEnd={onNodeDragEnd}
onDragEnter={onNodeDragEnter}
onDragLeave={onNodeDragLeave}
onDragOver={onNodeDragOver}
onDrop={onNodeDrop}
>
{children}
</TreeDndContext>
</ONESDndContext>
// T 就是 useDndItem 中传入的 data 类型
export interface DragEventInfo<T = AnyData, N extends string = ''> {
name: N
key: React.Key
node: T
dragNode: T
active: Active
nativeEvent: Event
}
创建一个拖拽/放置元素,必须在 ONESDndContext
中使用
参数:
DragOverlay
、影响事件回调参数等返回值:
const { setNodeRef, isDragging, isOver, listeners, attributes } = useDndItem({
id: nodeKey,
data: {
key: nodeKey,
content,
// ...any data
},
})
return (
<div className="dnd-item" ref={setNodeRef} {...listeners} {...attributes}></div>
)
可以获取到目前的拖拽上下文
export function useTreeDndContext() {
const dndKitContext = useDndContext()
const TreeDndContext = React.useContext(Context)
return {
...dndKitContext,
...TreeDndContext,
}
}
@ones-design/dnd
内置了一些拖拽修饰器,用于调整拖拽节点的位置
export declare type Modifier = (args: {
activatorEvent: Event | null
active: Active | null
activeNodeRect: ClientRect | null
draggingNodeRect: ClientRect | null
containerNodeRect: ClientRect | null
over: Over | null
overlayNodeRect: ClientRect | null
scrollableAncestors: Element[]
scrollableAncestorRects: ClientRect[]
transform: Transform
windowRect: ClientRect | null
}) => Transform
被拖拽元素跟随鼠标移动,目前应用在 @ones-design/tree
上
TODO::这个 modifier 实际和树拖拽没有绑定关系,只是现在的实现依赖 TreeContext,因为目前没有一个 BaseContext 来导出坐标等数据
const mouseFollowModifier = useMouseFollowModifier()
<DragOverlay modifires={[mouseFollowModifier]}/>
被拖拽元素只能在垂直方向移动,目前应用在 @ones-design/table
上
const verticalRestrictModifier = useVerticalRestrictModifier()
<DragOverlay modifires={[verticalRestrictModifier]}/>
FAQs
The npm package @ones-design/dnd receives a total of 99 weekly downloads. As such, @ones-design/dnd popularity was classified as not popular.
We found that @ones-design/dnd demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.