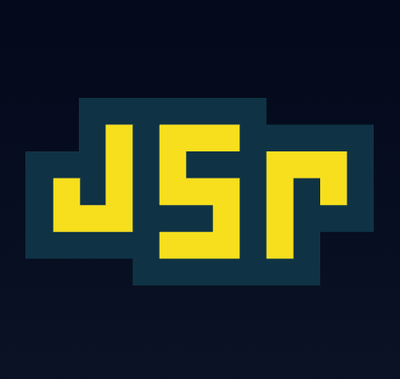
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@oozcitak/dom
Advanced tools
@oozcitak/dom is a lightweight, standards-compliant DOM implementation for Node.js. It allows you to create, manipulate, and traverse DOM trees in a server-side environment, similar to how you would in a browser.
Creating a DOM Document
This feature allows you to create a new DOM document. The code sample demonstrates how to create a new document with a root element and serialize it to a string.
const { DOMImplementation, XMLSerializer } = require('@oozcitak/dom');
const impl = new DOMImplementation();
const doc = impl.createDocument(null, 'root', null);
const serializer = new XMLSerializer();
console.log(serializer.serializeToString(doc));
Manipulating DOM Elements
This feature allows you to manipulate DOM elements. The code sample demonstrates how to create a new element, set its text content, and append it to the root element.
const { DOMImplementation, XMLSerializer } = require('@oozcitak/dom');
const impl = new DOMImplementation();
const doc = impl.createDocument(null, 'root', null);
const root = doc.documentElement;
const child = doc.createElement('child');
child.textContent = 'Hello, World!';
root.appendChild(child);
const serializer = new XMLSerializer();
console.log(serializer.serializeToString(doc));
Traversing the DOM
This feature allows you to traverse the DOM tree. The code sample demonstrates how to create multiple child elements, append them to the root, and then traverse the children to log their text content.
const { DOMImplementation, XMLSerializer } = require('@oozcitak/dom');
const impl = new DOMImplementation();
const doc = impl.createDocument(null, 'root', null);
const root = doc.documentElement;
const child1 = doc.createElement('child');
child1.textContent = 'Child 1';
const child2 = doc.createElement('child');
child2.textContent = 'Child 2';
root.appendChild(child1);
root.appendChild(child2);
const children = root.getElementsByTagName('child');
for (let i = 0; i < children.length; i++) {
console.log(children[i].textContent);
}
jsdom is a popular package that provides a complete, standards-compliant JavaScript environment, including the DOM, for Node.js. It is more feature-rich and closely mimics a browser environment compared to @oozcitak/dom, making it suitable for more complex use cases.
xmldom is another package that provides a DOM implementation for XML documents in Node.js. It is similar to @oozcitak/dom but focuses more on XML parsing and serialization, making it a good choice for XML-specific tasks.
domino is a fast and minimalistic DOM implementation for Node.js. It is designed to be lightweight and efficient, similar to @oozcitak/dom, but may lack some of the more advanced features found in jsdom.
A Javascript implementation of the DOM Living Standard.
Current version implements the standard as of commit 57512fa (Last Updated 24 September 2019).
This DOM implementation is for XML documents only.
npm install @oozcitak/dom
Create an instance of the DOMImplementation
class to construct the DOM tree.
const { DOMImplementation } = require("@oozcitak/dom");
const dom = new DOMImplementation();
const doc = dom.createDocument('ns', 'root');
The module also exports DOMParser
and XMLSerializer
classes as in the browser.
FAQs
A modern DOM implementation
We found that @oozcitak/dom demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.