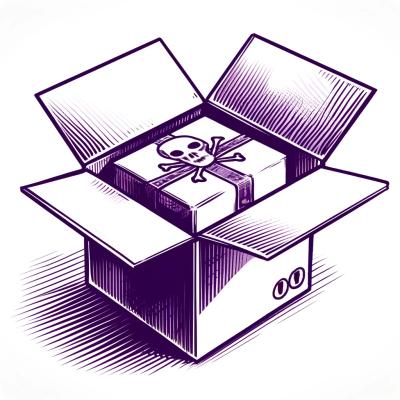
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
A pure JavaScript W3C standard-based (XML DOM Level 2 Core) DOMParser and XMLSerializer module.
The xmldom npm package is a pure JavaScript W3C standard-based (XML DOM Level 2 Core) DOMParser and XMLSerializer module. It allows users to parse XML data into a DOM tree structure and serialize DOM trees back into XML. This package is particularly useful for server-side applications where DOM manipulation is needed without a browser environment.
Parsing XML to DOM
This feature allows you to parse a string of XML into a DOM tree, enabling programmatic access and manipulation of the structure and content.
const { DOMParser } = require('xmldom');
const xmlString = '<root>Hello World</root>';
const doc = new DOMParser().parseFromString(xmlString, 'text/xml');
console.log(doc.documentElement.nodeName); // 'root'
Serializing DOM to XML
This feature enables you to take a DOM tree and serialize it back into a string of XML, useful for outputting modified XML data.
const { XMLSerializer } = require('xmldom');
const doc = new DOMParser().parseFromString('<root>Hello World</root>', 'text/xml');
const xmlString = new XMLSerializer().serializeToString(doc);
console.log(xmlString); // '<root>Hello World</root>'
libxmljs is a native library for parsing and manipulating XML. It offers faster performance due to its native implementation but requires compilation, which can be a downside compared to the pure JavaScript implementation of xmldom.
cheerio is primarily used for web scraping and server-side manipulation of HTML. While it can handle XML and offers a jQuery-like syntax for manipulation, it's more tailored towards HTML content. Compared to xmldom, cheerio might be more user-friendly for those familiar with jQuery but less strict in terms of XML standards compliance.
A JavaScript implementation of W3C DOM for Node.js, Rhino and the browser. Fully
compatible with W3C DOM level2
; and some compatible with level3
. Supports
DOMParser
and XMLSerializer
interface such as in browser.
Original project location: https://github.com/jindw/xmldom
npm install xmldom
const { DOMParser } = require('xmldom')
const doc = new DOMParser().parseFromString(
'<xml xmlns="a" xmlns:c="./lite">\n' +
'\t<child>test</child>\n' +
'\t<child></child>\n' +
'\t<child/>\n' +
'</xml>',
'text/xml'
)
doc.documentElement.setAttribute('x', 'y')
doc.documentElement.setAttributeNS('./lite', 'c:x', 'y2')
console.info(doc)
const nsAttr = doc.documentElement.getAttributeNS('./lite', 'x')
console.info(nsAttr)
Note: in Typescript and ES6 you can use the import approach, as follows:
import { DOMParser } from 'xmldom'
parseFromString(xmlsource,mimeType)
//added the options argument
new DOMParser(options)
//errorHandler is supported
new DOMParser({
/**
* locator is always need for error position info
*/
locator:{},
/**
* you can override the errorHandler for xml parser
* @link http://www.saxproject.org/apidoc/org/xml/sax/ErrorHandler.html
*/
errorHandler:{warning:function(w){console.warn(w)},error:callback,fatalError:callback}
//only callback model
//errorHandler:function(level,msg){console.log(level,msg)}
})
serializeToString(node)
attribute:
nodeValue|prefix
readonly attribute:
nodeName|nodeType|parentNode|childNodes|firstChild|lastChild|previousSibling|nextSibling|attributes|ownerDocument|namespaceURI|localName
method:
insertBefore(newChild, refChild)
replaceChild(newChild, oldChild)
removeChild(oldChild)
appendChild(newChild)
hasChildNodes()
cloneNode(deep)
normalize()
isSupported(feature, version)
hasAttributes()
DOMException
The DOMException class has the following constants (and value
of type Number
):
DOMException.INDEX_SIZE_ERR
(1
)DOMException.DOMSTRING_SIZE_ERR
(2
)DOMException.HIERARCHY_REQUEST_ERR
(3
)DOMException.WRONG_DOCUMENT_ERR
(4
)DOMException.INVALID_CHARACTER_ERR
(5
)DOMException.NO_DATA_ALLOWED_ERR
(6
)DOMException.NO_MODIFICATION_ALLOWED_ERR
(7
)DOMException.NOT_FOUND_ERR
(8
)DOMException.NOT_SUPPORTED_ERR
(9
)DOMException.INUSE_ATTRIBUTE_ERR
(10
)DOMException.INVALID_STATE_ERR
(11
)DOMException.SYNTAX_ERR
(12
)DOMException.INVALID_MODIFICATION_ERR
(13
)DOMException.NAMESPACE_ERR
(14
)DOMException.INVALID_ACCESS_ERR
(15
)The DOMException object has the following properties: code This property is of type Number.
method:
hasFeature(feature, version)
createDocumentType(qualifiedName, publicId, systemId)
createDocument(namespaceURI, qualifiedName, doctype)
Document : Node
readonly attribute:
doctype|implementation|documentElement
method:
createElement(tagName)
createDocumentFragment()
createTextNode(data)
createComment(data)
createCDATASection(data)
createProcessingInstruction(target, data)
createAttribute(name)
createEntityReference(name)
getElementsByTagName(tagname)
importNode(importedNode, deep)
createElementNS(namespaceURI, qualifiedName)
createAttributeNS(namespaceURI, qualifiedName)
getElementsByTagNameNS(namespaceURI, localName)
getElementById(elementId)
DocumentFragment : Node
Element : Node
readonly attribute:
tagName
method:
getAttribute(name)
setAttribute(name, value)
removeAttribute(name)
getAttributeNode(name)
setAttributeNode(newAttr)
removeAttributeNode(oldAttr)
getElementsByTagName(name)
getAttributeNS(namespaceURI, localName)
setAttributeNS(namespaceURI, qualifiedName, value)
removeAttributeNS(namespaceURI, localName)
getAttributeNodeNS(namespaceURI, localName)
setAttributeNodeNS(newAttr)
getElementsByTagNameNS(namespaceURI, localName)
hasAttribute(name)
hasAttributeNS(namespaceURI, localName)
Attr : Node
attribute:
value
readonly attribute:
name|specified|ownerElement
readonly attribute:
length
method:
item(index)
readonly attribute:
length
method:
getNamedItem(name)
setNamedItem(arg)
removeNamedItem(name)
item(index)
getNamedItemNS(namespaceURI, localName)
setNamedItemNS(arg)
removeNamedItemNS(namespaceURI, localName)
CharacterData : Node
method:
substringData(offset, count)
appendData(arg)
insertData(offset, arg)
deleteData(offset, count)
replaceData(offset, count, arg)
Text : CharacterData
method:
splitText(offset)
Comment : CharacterData
readonly attribute:
name|entities|notations|publicId|systemId|internalSubset
Notation : Node
readonly attribute:
publicId|systemId
Entity : Node
readonly attribute:
publicId|systemId|notationName
EntityReference : Node
ProcessingInstruction : Node
attribute:
data
readonly attribute:
target
attribute:
textContent
method:
isDefaultNamespace(namespaceURI){
lookupNamespaceURI(prefix)
[Node] Source position extension;
attribute:
//Numbered starting from '1'
lineNumber
//Numbered starting from '1'
columnNumber
FAQs
A pure JavaScript W3C standard-based (XML DOM Level 2 Core) DOMParser and XMLSerializer module.
We found that xmldom demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.