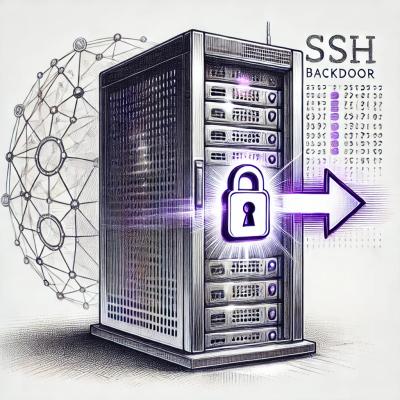
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@open-rpc/server-js
Advanced tools
JSON-RPC 2.0 + OpenRPC Server implementation that supports multiple transport protocols. Built to run with node 10+.
Need help or have a question? Join us on Discord!
install server, and optionally schema-utils-js if you want to dereference/validate the open-rpc document before running.
npm install --save @open-rpc/server-js @open-rpc/schema-utils-js
Write an open-rpc document describing your service
./src/openrpc.json
see: https://raw.githubusercontent.com/open-rpc/examples/master/service-descriptions/simple-math-openrpc.json
or write your own in playground.
For each of the methods, create a function that has the same name
src/method-mapping
import { MethodMapping } from "@open-rpc/server-js/build/router";
export const methodMapping: MethodMapping = {
addition: (a: number, b: number) => a + b,
subtraction: (a: number, b: number) => a - b
};
export default methodMapping;
Create a server with the methods and the document, serve it over http and websocket
src/server.ts
import { Server, ServerOptions } from "@open-rpc/server-js";
import { HTTPServerTransportOptions } from "@open-rpc/server-js/build/transports/http";
import { WebSocketServerTransportOptions } from "@open-rpc/server-js/build/transports/websocket";
import { OpenrpcDocument } from "@open-rpc/meta-schema";
import { parseOpenRPCDocument } from "@open-rpc/schema-utils-js";
import methodMapping from "./method-mapping";
import doc from "./openrpc.json";
export async function start() {
const serverOptions: ServerOptions = {
openrpcDocument: await parseOpenRPCDocument(doc as OpenrpcDocument),
transportConfigs: [
{
type: "HTTPTransport",
options: {
port: 3330,
middleware: [],
} as HTTPServerTransportOptions,
},
{
type: "WebSocketTransport",
options: {
port: 3331,
middleware: [],
} as WebSocketServerTransportOptions,
},
],
methodMapping,
};
console.log("Starting Server"); // tslint:disable-line
const s = new Server(serverOptions);
s.start();
}
import { types } from "@open-rpc/meta-schema";
import { Router } from "@open-rpc/server-js";
const openrpcDocument = {
openrpc: "1.0.0",
info: {
title: "node-json-rpc-server example",
version: "1.0.0"
},
methods: [
{
name: "addition",
params: [
{ name: "a", schema: { type: "integer" } },
{ name: "b", schema: { type: "integer" } }
],
result: {
{ name: "c", schema: { type: "integer" } }
}
}
]
} as types.OpenRPC;
const methodHandlerMapping = {
addition: (a: number, b: number) => Promise.resolve(a + b)
};
const router = new Router(openrpcDocument, methodHandlerMapping);
const router = new Router(openrpcDocument, { mockMode: true });
import { TCPIPCServerTranport, UDPIPCServerTranport } from "@open-rpc/server-js";
const ipcOptions = { maxConnetions: 20 }; // https://www.npmjs.com/package/node-ipc#ipc-config
const TCPIPCOptions = { ...ipcOptions, networkPort: 4343 };
const UDPIPCOptions = { ...ipcOptions, networkPort: 4343, udp: true };
const tcpIpcTransport = new IPCServerTranport(TCPIPCTransportOptions);
const UdpIpcTransport = new IPCServerTranport(UDPIPCTransportOptions);
import { HTTPServerTransport, HTTPSServerTransport } from "@open-rpc/server-js";
const httpOptions = {
middleware: [ cors({ origin: "*" }) ],
port: 4345
};
const httpsOptions = { // extends https://nodejs.org/api/https.html#https_https_createserver_options_requestlistener
middleware: [ cors({ origin: "*" }) ],
port: 4346,
key: await fs.readFile("test/fixtures/keys/agent2-key.pem"),
cert: await fs.readFile("test/fixtures/keys/agent2-cert.pem"),
ca: fs.readFileSync("ssl/ca.crt")
};
const httpTransport = new HTTPServerTransport(httpOptions);
const httpsTransport = new HTTPSServerTransport(httpsOptions); // Defaults to using HTTP2, allows HTTP1.
import { WebSocketServerTransport } from "@open-rpc/server-js";
const webSocketFromHttpsOptions = { // extends https://github.com/websockets/ws/blob/master/doc/ws.md#new-websocketserveroptions-callback
server: httpsTransport.server
};
const webSocketOptions = { // extends https://github.com/websockets/ws/blob/master/doc/ws.md#new-websocketserveroptions-callback
port: 4347
};
const wsFromHttpsTransport = new WebSocketServerTransport(webSocketFromHttpsOptions); // Accepts http transport as well.
const wsTransport = new WebSocketServerTransport(webSocketOptions); // Accepts http transport as well.
const server = new Server();
server.start();
server.addTransport(httpsTransport); // will be started immediately
server.setRouter(router);
server.addTransports([ wsTransport, wsFromHttpsTransport, httpsTransport ]); // will be started immediately.
How to contribute, build and release are outlined in CONTRIBUTING.md, BUILDING.md and RELEASING.md respectively. Commits in this repository follow the CONVENTIONAL_COMMITS.md specification.
FAQs
<center> <img alt="Dependabot status"
The npm package @open-rpc/server-js receives a total of 4,702 weekly downloads. As such, @open-rpc/server-js popularity was classified as popular.
We found that @open-rpc/server-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.