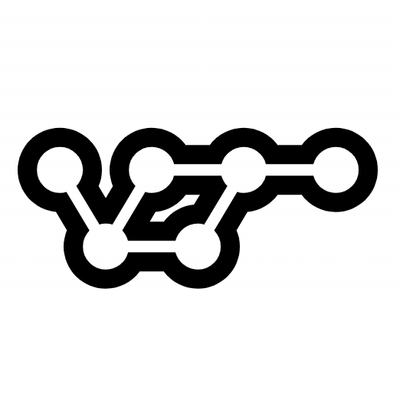
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@open-wc/building-webpack
Advanced tools
Part of Open Web Components
Open Web Components provides a set of defaults, recommendations and tools to help facilitate your web component project. Our recommendations include: developing, linting, testing, building, tooling, demoing, publishing and automating.
Our webpack configuration will help you get started using webpack. Our configuration lets you write code using modern javascript syntax and features, providing the necessary syntax transformation and polyfills for older browsers.
See 'config features' for all details. See the extending section for customization, such as supporting non-standard syntax or adding babel plugins.
npm init @open-wc building-webpack
npm i -D @open-wc/building-webpack webpack http-server webpack-dev-server
webpack.config.js
and pass in your app's js entry point and index.html.If you don't need to support IE11 or other legacy browsers, use @open-wc/building-webpack/modern-config
. Otherwise, use @open-wc/building-webpack/modern-and-legacy-config
.
import createDefaultConfig from '@open-wc/building-webpack/modern-and-legacy-config';
// If you don't need IE11 support, use the modern-config instead
// import createDefaultConfig from '@open-wc/building-webpack/modern-config';
export default createDefaultConfig({
entry: path.resolve(__dirname, './my-app.js'),
indexHTML: path.resolve(__dirname, './index.html'),
});
index.html
:<!doctype html>
<html>
<head></head>
<body>
<your-app></your-app>
</body>
</html>
We use html-webpack-plugin to work with your index.html. View the documentation for the plugin to learn how you can use it.
Remember to not add your app's entry point to your index.html, as it will be injected dynamically by html-webpack-plugin
.
.browserslistrc
in the root of your project. Adjust it based on your browser support, for example:last 2 Chrome major versions
last 2 ChromeAndroid major versions
last 2 Edge major versions
last 2 Firefox major versions
last 2 Safari major versions
last 2 iOS major versions
Do not put IE11 in your .browserslistrc
. modern-and-legacy-config
already creates a separate build for IE11, the .browserslistrc
is used for the modern build.
package.json
:{
"scripts": {
"build": "webpack --mode production",
"start": "webpack-dev-server --mode development --open",
"start:build": "http-server dist -o",
}
}
build
builds your app and outputs it in your dist
directorystart
builds and runs your app, rebuilding on file changesstart:build
runs your built app from dist
directory, it uses a simple http-server to make sure that it runs without magicmodern-config.js
creates a single build of your app, based on your .browserslistrc
. This is recommended if you only need to support modern browsers, otherwise you will need to ship compatibility code for browsers which don't need it.
modern-and-legacy-config.js
creates two builds of your app. A modern build based on your .browserslistrc
and a legacy build for IE11. Additional code is injected to load polyfills and the correct version of your app. This is recommended if you need to support IE11.
All configs:
.browserslistrc
import { html } from 'lit-html'
)import.meta.url
value from before bundlingmodern-config.js
:
modern-and-legacy-config.js
:
.browserslistrc
Array.from
, String.prototype.includes
etc.)See 'extending' to add more configuration.
You can define your own babel plugins by adding a .babelrc
or babel.config.js
to your project. See babeljs config for more information.
For example to add support for class properties:
{
"plugins": [
"@babel/plugin-proposal-class-properties"
]
}
A webpack config is an object. To extend it, we recommend using webpack-merge
to ensure plugins are merged correctly. For example to adjust the output folder:
const merge = require('webpack-merge');
const createDefaultConfig = require('@open-wc/building-webpack/modern-config');
const config = createDefaultConfig({
entry: path.resolve(__dirname, './my-app.js'),
indexHTML: path.resolve(__dirname, './index.html'),
});
module.exports = merge(config, {
output: {
path: 'build'
},
});
If you use modern-and-legacy-config.js
, it is actually an array of configs so that webpack outputs a modern and a legacy build. Simply map over the array to adjust both configs:
const merge = require('webpack-merge');
const createDefaultConfigs = require('@open-wc/building-webpack/modern-and-legacy-config');
const configs = createDefaultConfigs({
entry: path.resolve(__dirname, './my-app.js'),
indexHTML: path.resolve(__dirname, './index.html'),
});
module.exports = configs.map(config => merge(config, {
output: {
path: 'build'
},
}));
::: warning Some extensions add non-native syntax to your code, which can be bad for maintenance longer term. We suggest avoiding adding plugins for using experimental javascript proposals or for importing non-standard module types. :::
Web apps often include assets such as css files and images. These are not part of your regular dependency graph, so they need to be copied into the build directory.
copy-webpack-plugin is a popular plugin fo this.
const path = require('path');
const merge = require('webpack-merge');
const CopyWebpackPlugin = require('copy-webpack-plugin');
const createDefaultConfigs = require('@open-wc/building-webpack/modern-and-legacy-config');
const configs = createDefaultConfigs({
entry: path.resolve(__dirname, './demo-app.js'),
indexHTML: path.resolve(__dirname, './index.html'),
});
// with modern-and-legacy-config, the config is actually an array of configs for a modern and
// a legacy build. We don't need to copy files twice, so we aadd the copy job only to the first
// config
module.exports = [
// add plugin to the first config
merge(configs[0], {
plugins: [
new CopyWebpackPlugin([
'images/**/*.png',
]),
],
}),
// the second config left untouched
configs[1],
];
Make sure to prevent any compilation done by the typescript compiler tsconfig.json
, as babel and webpack do this for you:
{
"compilerOptions": {
"target": "ESNEXT",
"module": "ESNext",
}
}
Within webpack there are two options to add typescript support.
We recommend using the babel typescript plugin. Add this to your .babelrc
:
{
"presets": [
"@babel/preset-typescript"
],
}
This the fastest method, as it strips away types during babel transformormation of your code. It will not perform any type checking though. We recommend setting up the type checking as part of your linting setup, so that you don't need to run the typechecker during development for faster builds.
It is also possible to add the webpack typescript plugin, which does typechecking and compiling for you:
const path = require('path');
const merge = require('webpack-merge');
const createDefaultConfigs = require('@open-wc/building-webpack/modern-and-legacy-config');
const configs = createDefaultConfigs({
entry: path.resolve(__dirname, './demo-app.ts'),
indexHTML: path.resolve(__dirname, './index.html'),
});
module.exports = configs.map(config =>
merge(config, {
module: {
rules: [{ test: /\.ts$/, loader: 'ts-loader' }],
},
}),
);
FAQs
Default configuration for working with webpack
The npm package @open-wc/building-webpack receives a total of 643 weekly downloads. As such, @open-wc/building-webpack popularity was classified as not popular.
We found that @open-wc/building-webpack demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.