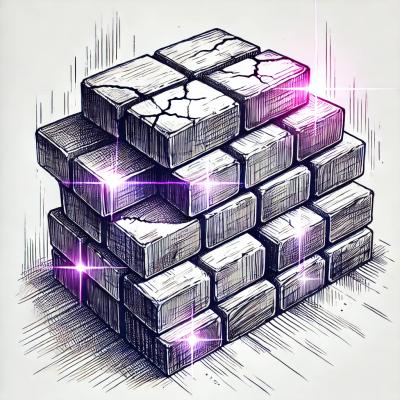
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@openfin/bloomberg
Advanced tools
WARNING: this is a non-production preview of an OpenFin product. Please contact support@openfin.co before trying to use.
The OpenFin Bloomberg Integration is a client API for connecting OpenFin apps to the local instance of the Bloomberg Terminal service running on a user's desktop.
This API uses Bloomberg's Terminal Connect API in order to communicate with the Bloomberg Terminal.
Use this API to:
In order for an app to use this API, there must be a logged in Bloomberg Terminal running on the user's desktop.
In order for an app to use Bloomberg's Terminal Connect API, the origin of the app must first be registered with Bloomberg before it can communicate with the Bloomberg Terminal. The origin must include a Fully Qualified Domain Name (FQDN), and cannot include localhost. To register your app's origin, contact Bloomberg's App Portal team either via live help in the Terminal or raise a ticket via the Customer Service Center.
First, add the package as a dependency for your app.
npm install @openfin/bloomberg
or
yarn add @openfin/bloomberg
If you plan on creating FDC3 connections with Bloomberg, ensure the service has been declared in your application manifest.
"services": [
{
"name": "fdc3"
}
]
Import required functions and types into your codebase.
import { connect, connectFdc3, connectInterApplicationBus } from '@openfin/bloomberg';
Connections enable you to continuously send and receive securities to and from Bloomberg Terminal panels and Launchpad groups.
Before a connection can be created, ensure that the Bloomberg Terminal is running and a user is logged in by calling isBloombergTerminalReady
.
import { isBloombergTerminalReady } from '@openfin/bloomberg';
// Only proceed if the Terminal is running and logged in
const terminalReady = await isBloombergTerminalReady();
if (!terminalReady) {
return;
}
When creating a connection you must specify a source
and/or a target
, and callback functions. The source
is the name of a Launchpad group that will trigger the onChange
callback function when it's security is changed from within Bloomberg. The target
is the name of a Launchpad group or panel number out of the four Terminal panels that will have it's security updated.
Note: Terminal panels do not trigger events when their security is changed and so cannot be used as a source
. When a panel is used as a target
, the current function will be set to 'DES' when it receives a security update.
You can create multiple connections with different combinations of source
and target
. To specify all groups, provide '*'
as the value instead of the group name.
import { connect } from '@openfin/bloomberg';
// Send updates to Terminal panel 1 and ignore group updates
const conn1 = await connect(null, 1, console.log, console.error);
// Send updates to Terminal panel 2 and receive updates from Launchpad group 'A'
const conn2 = await connect('Group-A', 2, console.log, console.error);
// Send updates to Launchpad group 'A' and receive updates from all groups
const conn3 = await connect('*', 'Group-A', console.log, console.error);
// Send updates to all Launchpad groups and receive updates from my custom-named group
const conn4 = await connect('My Group', '*', console.log, console.error);
The connect
function returns a promise that will resolve with a connection object. Update the security of the target
by calling the setSecurity
function, providing a full Bloomberg security string.
// Update the displayed security in Terminal panel 1 to 'TSLA US Equity'
await conn1.setSecurity('TSLA US Equity');
// Update the displayed security in Terminal panel 2 to 'GBP Curncy' using it's FIGI
await conn2.setSecurity('BBG0013HFH84 Curncy');
// Providing an incomplete security string throws an error
await conn3.setSecurity('25468PBW5');
When a source
has it's security changed from within Bloomberg, the onChange
callback will be called with the new Bloomberg security string.
// Log console message whenever Launchpad group 'A' security changes
const conn5 = await connect('Group-A', null, (security) => console.log(`New security: ${security}`));
// Group A security is updated to 'TSLA US Equity', console message logged:
// New security: TSLA US Equity
When finished with a connection, call close
to clean up resources.
await conn1.close();
The OpenFin InterApplicationBus (IAB) is a secure desktop messaging system that supports a number of different interoperability strategies, including pub/sub and topic based messaging, direct connections between applications, and one-to-many connections.
OpenFin's Bloomberg Integration builds on this technology and connects the Bloomberg Terminal to apps over the IAB. When creating a Bloomberg IAB connection, define the topic
for the connection and an optional applicationId
to target, otherwise all apps will be targeted. Any messages received over the IAB on the defined topic
will be considered a security and the target
panel/group security will be updated to match. When a source
group security is changed, the new security will be sent over the IAB as a message to the app matching the applicationId
(or published to all apps if no applicationId
was set) on the specified topic
.
// app1
// Subscribe to an IAB topic 'bbg-security' with app2
// (security updates from Bloomberg received over IAB will be output to console)
fin.InterApplicationBus.subscribe({ uuid: 'app2' }, 'bbg-security', console.log);
// app2
import { connectInterApplicationBus } from '@openfin/bloomberg';
// Create an IAB connection with Launchpad group 'A' on topic 'bbg-security' to app1
const connection = await connectInterApplicationBus(
'bbg-security',
'app1',
'Group-A',
'Group-A',
console.log,
console.error
);
// app1
// Update Bloomberg to 'TSLA US Equity' using IAB
fin.InterApplicationBus.send({ uuid: 'app2' }, 'bbg-security', 'TSLA US Equity');
// or
fin.InterApplicationBus.publish('bbg-security', 'TSLA US Equity');
When finished with the connection, call close
to clean up resources.
// app2
await connection.close();
FDC3 stands for Financial Desktop Connectivity and Collaboration Consortium, and is an industry standard enabling desktop application interoperability to lower developer costs and transform user workflows.
Applications that are FDC3-enabled can take part in a workflow on the desktop without any coding or manual integration, allowing replacement of one application with another application serving the same functions to the desktop.
OpenFin's Bloomberg Integration connects the Bloomberg Terminal to FDC3-enabled apps, allowing them to set securities in Bloomberg and receiving context when securities are changed in the Terminal. When creating a Bloomberg FDC3 connection, specify the contextChannel
that the connection will use, otherwise the default channel will be used. When a broadcast is received on the specified channel, if the context that was broadcast is an InstrumentContext the target
panel/group security will be updated to match.
Note: in order that Bloomberg is updated correctly, the context object should define the BBG
id value as the Bloomberg security string. If no such value is set, the ticker
id value (if set) will be assumed as a US equity and used instead. If neither the BBG
or ticker
values are set, the context will be discarded and Bloomberg will not be updated.
When a source
group security is changed, the new security will be converted to an InstrumentContext and broadcast on the specified channel. The context object will contain the full Bloomberg security string as the BBG
id value, and the instrument code as the ticker
id value.
// app1
importas fdc3 from 'openfin-fdc3';
// Listen out for broadcasts on the default FDC3 channel
// (security updates from Bloomberg received over FDC3 will be output to console)
fdc3.addContextListener(console.log);
// app2
import { connectFdc3 } from '@openfin/bloomberg';
importas fdc3 from 'openfin-fdc3';
// Create an FDC3 connection with Launchpad group 'A' on the default channel
const connection = await connectFdc3(fdc3.defaultChannel, 'Group-A', 'Group-A', console.log, console.error);
// app1
import { getInstrumentContextFromSecurity } from '@openfin/bloomberg';
// Update Bloomberg to 'TSLA US Equity' over FDC3 default channel
const context = getInstrumentContextFromSecurity('TSLA US Equity');
await fdc3.broadcast(context);
When finished with the connection, call close
to clean up resources.
// app2
await connection.close();
The OpenFin Bloomberg Integration builds on top of Bloomberg's Terminal Connect API by adding the ability to create connections using OpenFin technologies. The terminal
instance can be imported in order to access Terminal Connect API functionality directly. This is useful when you want to update the current function in a Terminal panel, or retrieve the list of Launchpad groups.
import { terminal } from '@openfin/bloomberg';
// Update Terminal panel 1 to DIS 7 sell ticket 2mm @ 102
await terminal.runFunction('STT', 1, '25468PBW5 Corp', null, '2000 102');
// Output the names of all Launchpad groups to the console
const groups = await terminal.getAllGroups();
groups.forEach((group) => console.log(group.name));
When creating a Bloomberg connection, the optional onError
callback will be fired if and when an error occurs. The error object passed to onError
will be an instance of the BloombergApiError
class or a subclass if the error is relevant to a specific case. For example, if when a connection is created the Terminal is not running or a user is not logged in, a TerminalConnectionError
will be thrown. Another example, if the app calling the API has not been properly authorised with Bloomberg, a AppNotAuthorizedError
will be thrown.
API errors contain standard Error
properties as expected, but are also provided with user-friendly title
and description
properties which are handy for when you want to inform the user about the error.
import { BloombergApiError, connect } from '@openfin/bloomberg';
import { create, NotificationIndicatorType } from 'openfin-notifications';
// Display a notification in OpenFin if an API error occurs
const handleError = async (error) => {
if (error! instanceof BloombergApiError) {
await create({
title: error.title,
body: error.description,
category: 'Failure',
indicator: {
type: NotificationIndicatorType.FAILURE,
},
});
}
};
let connection;
try {
// If Bloomberg Terminal is not running this will throw a TerminalConnectionError
// Error will be handled in catch block and a notification will be displayed
connection = await connect(null, 1, console.log, handleError);
} catch (err) {
handleError(err);
return;
}
// Calling setSecurity with an invalid security string will result in a BloombergSecurityError
// being thrown, this will be caught by handleError and a notification will be displayed
await connection.setSecurity('25468PBW5');
This API also provides a number of utility functions that can be useful when working with Bloomberg.
import {
getInstrumentCodeFromSecurity,
getSecurityStringFromParts,
isCusip,
isEquity,
isFigi,
isIsin,
} from '@openfin/bloomberg';
// Returns '25468PBW5'
getInstrumentCodeFromSecurity('25468PBW5 Corp');
// Returns 'TSLA US Equity'
getSecurityStringFromParts('TSLA', 'US');
// Returns 'GBPUSD Curncy'
getSecurityStringFromParts('GBPUSD', null, 'Curncy');
// Returns true
isCusip('25468PBW5');
// Returns false
isCusip('BBG00005J747');
// Returns true
isEquity('TSLA US Equity');
// Returns false
isEquity('GBPUSD Curncy');
// Returns true
isFigi('BBG00005J747');
// Returns false
isFigi('US25468PBW59');
// Returns true
isIsin('US25468PBW59');
// Returns false
isIsin('BBG00005J747');
FAQs
Connect apps running in OpenFin with the Bloomberg Terminal.
The npm package @openfin/bloomberg receives a total of 58 weekly downloads. As such, @openfin/bloomberg popularity was classified as not popular.
We found that @openfin/bloomberg demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 58 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.