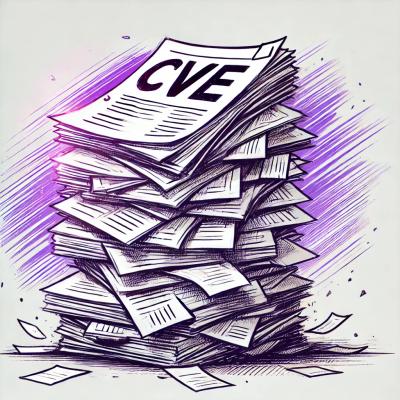
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@openpass/openpass-js-sdk
Advanced tools
A JavaScript SDK used to integrate with the OpenPass SSO Service.
Currently, the NPM package is not publicly available through NPM, and is a private package that belongs to the OpenPass organization scope. Consequently, to install the package the npm client must be authenticated with NPM.
The npm login
command can be used and the user can be added to the OpenPass organization See here.
Another option that can be used to authenticate with NPM is through the use of the .npmrc
file. See here for more information.
For example the .npmrc
file can be added to the root of your project with the following contents:
//registry.npmjs.org/:_authToken=${NPM_TOKEN}
@openpass:registry=https://registry.npmjs.org
@openpass:always-auth=true
Above the environment variable NPM_TOKEN
should contain a valid NPM access token with the permissions required to install the package.
The package can be installed with:
npm install @openpass/openpass-js-sdk
To use the sdk we first need to create an instance of the client.
const authClient = new openpass.OpenPassAuth({
clientId: <YOUR_CLIENT_ID>,
onAuthChange: (response) => {
console.log(response.authenticated);
console.log(response.advertising_token);
}
});
The client can be initialized with the following options:
Option | Required | Data Type | Description |
---|---|---|---|
clientId | Yes | String | Your OpenPass client identifier |
onAuthChange | No | Function | Callback to retrieve authentication status changes |
baseUrl | No | String | Optionally set the base url of the OpenPass SSO Service |
uid2BaseUrl | No | String | Optionally set the base url of the UID2 Operator Service |
The isAuthenticated function returns the current authentication status as a promise.
const authResponse = await authClient.isAuthenticated();
console.log(authResponse.authenticated);
console.log(authResponse.advertising_token);
The signInWithRedirect function will redirect to the authorization service to initiate a sign in attempt. On completion, the user will be redirected back to the given redirectUrl to complete the authentication process.
authClient.signInWithRedirect({
redirectUrl: <YOUR_REDIRECT_URL>,
clientState: <YOUR_CLIENT_STATE>
});
Option | Required | Data Type | Description |
---|---|---|---|
redirectUrl | Yes | String | A valid redirection url that processes a callback from the authorization server. This url must be configured with the authorization server. |
clientState | No | Object | An object containing any client side state that is stored and returned back, when an authentication session completes. This can be used to store the original url, to redirect back to after a successful authentication. |
The signInWithPopup function will initiate the sign-in process in a popup window instead of a redirecting the current browser window to the authorization service. If the popup fails to open or, sign-in fails an error may be thrown and, should be handled as shown below.
try {
await authClient.signInWithPopup();
} catch (e) {
//sign-in failed
console.log(e);
}
We can also configure the popup to revert to a full redirect for smaller devices, and in the event the popup cannot be opened.
try {
await authClient.signInWithPopup({
fallbackToRedirect: true,
redirectUrl: <YOUR_REDIRECT_URL>,
clientState: <YOUR_CLIENT_STATE>
});
} catch (e) {
console.log(e);
}
If fallback to redirect is enabled, we must also handle the response from the authorization service, by handling the redirect.
The following options will be used in the event the popup reverts to a full redirect.
Option | Required | Data Type | Description |
---|---|---|---|
fallbackToRedirect | No | Boolean | Set to true, to fallback to a redirect for smaller devices and in the event a popup cannot be opened. |
redirectUrl | No | String | Required if fallbackToRedirect is set to true. Should contain a valid redirection url that processes a callback from the authorization server. This url must be configured with the authorization server. If fallbackToRedirect is set to true and a redirect url is not specified an error will be thrown. |
clientState | No | Object | An object containing any client side state that is stored and returned back, when an authentication session completes. This can be used to store the original url, to redirect back to after a successful authentication. |
If there is no fallback to redirect, an object with the following properties is returned:
Property | Data Type | Description |
---|---|---|
authenticated | Boolean | Returns true if authenticated, otherwise false. |
uid2Identity | Uid2Identity | The Uid2 identity containing the advertising token |
String | The email of the signed in user. |
The uid2Identity
contains the following properties:
Property | Data Type | Description |
---|---|---|
advertising_token | String | The advertising token |
refresh_token | String | Token used to refresh the identity |
identity_expires | Number | Identity expiry time |
refresh_from | Number | Refresh start |
refresh_expires | Number | Refresh expiry time |
Determines if a sign in attempt with a redirect is currently in progress, and that the current browser url meets the conditions to consider it as callback from the authorization server.
authClient.isRedirect()
Determines if the current browser url meets the conditions to consider it as a valid redirection from the authorization server
authClient.isRedirectUrl(redirectUrl)
Parameter | Required | Data Type | Description |
---|---|---|---|
redirectUrl | Yes | String | A valid redirect url that processes a callback from the authorization server. |
Call this method to complete the authentication process in response to receiving a callback to a redirect url from the authorization service.
if(authClient.isRedirect()) {
try {
const authResponse = await authClient.handleRedirect();
console.log(authResponse.authenticated);
console.log(authResponse.uid2Identity.advertising_token);
console.log(authResponse.clientState);
} catch (e) {
console.log(e);
}
}
If authentication was successful, the handleRedirect function returns an object with the following properties:
Property | Data Type | Description |
---|---|---|
authenticated | Boolean | Returns true if authenticated, otherwise false. |
uid2Identity | Uid2Identity | The Uid2 identity containing the advertising token |
String | The email of the signed in user. | |
clientState | Object | An object containing any client side state that is stored and returned back, when an authentication session completes. This can be used to store the original url, to redirect back to after a successful authentication. |
The uid2Identity
contains the following properties:
Property | Data Type | Description |
---|---|---|
advertising_token | String | The advertising token |
refresh_token | String | Token used to refresh the identity |
identity_expires | Number | Identity expiry time |
refresh_from | Number | Refresh start |
refresh_expires | Number | Refresh expiry time |
Terminates a user session locally and redirects the browser to a given sign out URL when present.
authClient.signOut(signOutUrl)
Parameter | Required | Data Type | Description |
---|---|---|---|
signOutUrl | No | String | A valid redirect url to point the browser to when the user signs out. |
FAQs
OpenPass SSO JavaScript SDK
The npm package @openpass/openpass-js-sdk receives a total of 90 weekly downloads. As such, @openpass/openpass-js-sdk popularity was classified as not popular.
We found that @openpass/openpass-js-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.