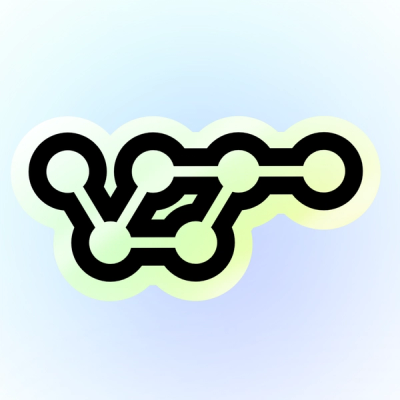
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
@opentelemetry/api
Advanced tools
The @opentelemetry/api package provides a set of APIs to instrument JavaScript applications for telemetry purposes. It allows developers to collect traces and metrics from their applications, which can then be exported to various observability backends for monitoring and analysis. The API is designed to be minimal, extensible, and vendor-neutral.
Tracing
This feature allows the creation and management of traces to monitor the flow of a request through various services. The code sample demonstrates how to create a tracer, start a new span, and then end the span.
const { trace } = require('@opentelemetry/api');
const tracer = trace.getTracer('example-tracer');
const span = tracer.startSpan('example-span');
span.end();
Context Propagation
This feature enables the propagation of context information across asynchronous operations or service boundaries. The code sample shows how to associate a span with a context and execute a function within this context.
const { context, trace } = require('@opentelemetry/api');
const currentContext = context.active();
const span = trace.getTracer('example-tracer').startSpan('example-span');
context.with(trace.setSpan(currentContext, span), () => {
// Your synchronous or asynchronous operation here
span.end();
});
Metrics
This feature supports the collection of quantitative measurements of operational events, such as request counts. The code sample illustrates how to create a meter, define a counter metric, and increment the counter.
const { metrics } = require('@opentelemetry/api');
const meter = metrics.getMeter('example-meter');
const counter = meter.createCounter('example-counter');
counter.add(1);
Jaeger client is a package for tracing applications and sending the traces to Jaeger, a distributed tracing system. Unlike @opentelemetry/api, which is designed to be vendor-neutral and supports multiple backends, jaeger-client is specifically tailored for integration with Jaeger.
Prom-client is a package for collecting metrics in Node.js applications and exporting them to Prometheus, a monitoring and alerting toolkit. While @opentelemetry/api provides a more general approach to metrics collection compatible with various backends, prom-client is specifically focused on Prometheus integration.
This package provides everything needed to interact with the OpenTelemetry API, including all TypeScript interfaces, enums, and no-op implementations. It is intended for use both on the server and in the browser.
To get started tracing you need to install the SDK and plugins, create a TracerProvider, and register it with the API.
$ # Install tracing dependencies
$ npm install \
@opentelemetry/core \
@opentelemetry/node \
@opentelemetry/tracing \
@opentelemetry/exporter-jaeger \ # add exporters as needed
@opentelemetry/plugin-http # add plugins as needed
$ # Install metrics dependencies
$ npm install \
@opentelemetry/metrics \
@opentelemetry/exporter-prometheus # add exporters as needed
Before any other module in your application is loaded, you must initialize the global tracer and meter providers. If you fail to initialize a provider, no-op implementations will be provided to any library which acquires them from the API.
To collect traces and metrics, you will have to tell the SDK where to export telemetry data to. This example uses Jaeger and Prometheus, but exporters exist for other tracing backends. If you're not sure if there is an exporter for your tracing backend, contact your tracing provider.
const { NodeTracerProvider } = require("@opentelemetry/node");
const { SimpleSpanProcessor } = require("@opentelemetry/tracing");
const { JaegerExporter } = require("@opentelemetry/exporter-jaeger");
const tracerProvider = new NodeTracerProvider();
/**
* The SimpleSpanProcessor does no batching and exports spans
* immediately when they end. For most production use cases,
* OpenTelemetry recommends use of the BatchSpanProcessor.
*/
tracerProvider.addSpanProcessor(
new SimpleSpanProcessor(
new JaegerExporter(
/* export options */
)
)
);
/**
* Registering the provider with the API allows it to be discovered
* and used by instrumentation libraries. The OpenTelemetry API provides
* methods to set global SDK implementations, but the default SDK provides
* a convenience method named `register` which registers same defaults
* for you.
*
* By default the NodeTracerProvider uses Trace Context for propagation
* and AsyncHooksScopeManager for context management. To learn about
* customizing this behavior, see API Registration Options below.
*/
tracerProvider.register();
const api = require("@opentelemetry/api");
const { MeterProvider } = require("@opentelemetry/metrics");
const { PrometheusExporter } = require("@opentelemetry/exporter-prometheus");
const meterProvider = new MeterProvider({
// The Prometheus exporter runs an HTTP server which
// the Prometheus backend scrapes to collect metrics.
exporter: new PrometheusExporter({ startServer: true }),
interval: 1000,
});
/**
* Registering the provider with the API allows it to be discovered
* and used by instrumentation libraries.
*/
api.metrics.setGlobalMeterProvider(meterProvider);
If you prefer to choose your own propagator or context manager, you may pass an options object into the tracerProvider.register()
method. Omitted or undefined
options will be replaced by a default value and null
values will be skipped.
const { B3Propagator } = require("@opentelemetry/core");
tracerProvider.register({
// Use B3 Propagation
propagator: new B3Propagator(),
// Skip registering a default context manager
contextManager: null,
});
If you are writing an instrumentation library, or prefer to call the API methods directly rather than using the register
method on the Tracer/Meter Provider, OpenTelemetry provides direct access to the underlying API methods through the @opentelemetry/api
package. API entry points are defined as global singleton objects trace
, metrics
, propagation
, and context
which contain methods used to initialize SDK implementations and acquire resources from the API.
const api = require("@opentelemetry/api");
/* Initialize TraceProvider */
api.trace.setGlobalTracerProvider(traceProvider);
/* returns traceProvider (no-op if a working provider has not been initialized) */
api.trace.getTracerProvider();
/* returns a tracer from the registered global tracer provider (no-op if a working provider has not been initialized); */
api.trace.getTracer(name, version);
/* Initialize MeterProvider */
api.metrics.setGlobalMeterProvider(meterProvider);
/* returns meterProvider (no-op if a working provider has not been initialized) */
api.metrics.getMeterProvider();
/* returns a meter from the registered global meter provider (no-op if a working provider has not been initialized); */
api.metrics.getMeter(name, version);
/* Initialize Propagator */
api.propagation.setGlobalPropagator(httpTraceContextPropagator);
/* Initialize Context Manager */
api.context.setGlobalContextManager(asyncHooksContextManager);
Library authors need only to depend on the @opentelemetry/api
package and trust that the application owners which use their library will initialize an appropriate SDK.
const api = require("@opentelemetry/api");
const tracer = api.trace.getTracer("my-library-name", "0.2.3");
async function doSomething() {
const span = tracer.startSpan("doSomething", { parent: tracer.getCurrentSpan() });
try {
const result = await doSomethingElse();
span.end();
return result;
} catch (err) {
span.setStatus({
// use an appropriate status code here
code: api.CanonicalCode.INTERNAL,
message: err.message,
});
span.end();
return null;
}
}
Apache 2.0 - See LICENSE for more information.
0.7.0
Released 2020-04-23
opentelemetry-exporter-collector
opentelemetry-api
, opentelemetry-metrics
opentelemetry-plugin-http
opentelemetry-core
opentelemetry-resources
opentelemetry-metrics
FAQs
Public API for OpenTelemetry
The npm package @opentelemetry/api receives a total of 10,420,944 weekly downloads. As such, @opentelemetry/api popularity was classified as popular.
We found that @opentelemetry/api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.